C++ Fundamentals: Understanding the Basics
A quick guide to Unordered Map in C++
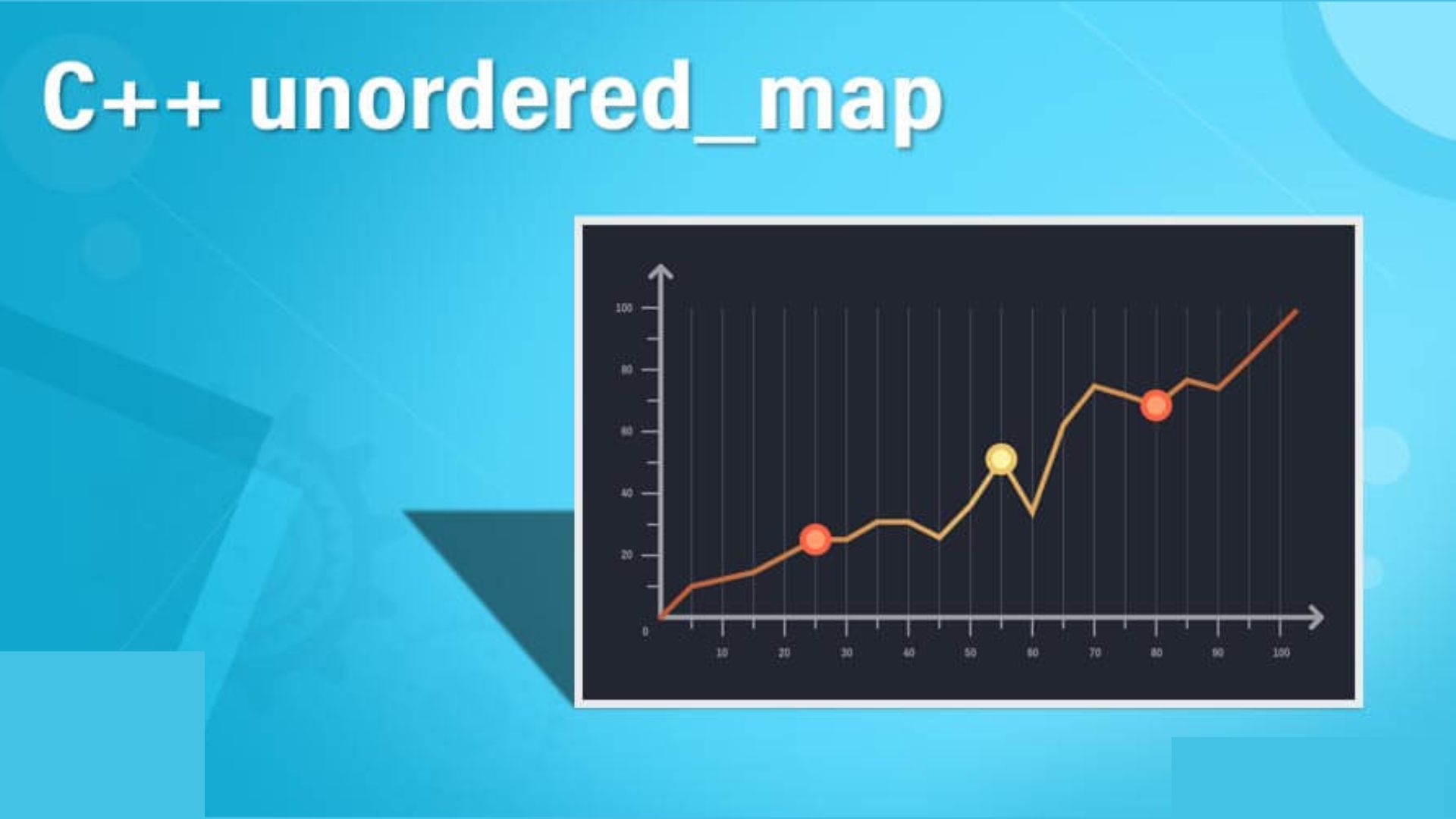
Introduction
In STL, there exist containers known as unordered maps that store the items as key-value pairs in any order. Every component has a different key value. Once they are put to the map, keys cannot be modified; they can only be added or removed. However, values may be updated. They are stored in memory as hash tables. The header file with the #include< map> tag contains the map. Iterators can be used to get access to the map's internal components.
Unordered_map Declaration
Syntax:
- key_data_type: data format for the key that will be kept inside the map
- value_data_type: a value to be put inside the map's data type
- initial_values:optional argument that sets the map's starting values
Example:
Functions on unordered_maps
- begin(): gives back an iterator for the unordered map's first element.
Parameters: None
Returns ->: iterator
- end(): gives an iterator to the next element in the unordered_map after the final element.
Parameters: None
Returns ->: iterator
- [key]:returns the value that matches the key supplied.
Parameters: Key
Returns ->: value, associated with the key
- size(): It provides the unordered_map size information.
Parameters: None
Returns ->: integer - total number of elements in the unordered_map
- insert(pair): In the unordered_map, add a pair.
Parameters: pair to insert
Returns ->: void
- erase(key):Take a component out of the unordered_map.
Parameters: The element's removal key or an iterator pointing to the spot where the element should be eliminated
Returns ->: void
- find(key): If the key is discovered, it returns an iterator referring to the element; otherwise, it yields an iterator referring to the unordered map's end.
Parameters: element to search
Returns ->: iterator
- clear(): The unordered_map whole components are removed.
Parameters: None
Returns ->: void
- empty(): It informs us whether or not the unordered_map is empty.
Parameters: None
Returns ->: True if empty, else false
Code
Hope this gave you a clear understanding of the concept.