Mastering Fundamentals: C++ and Data Structures
C++ Pointers
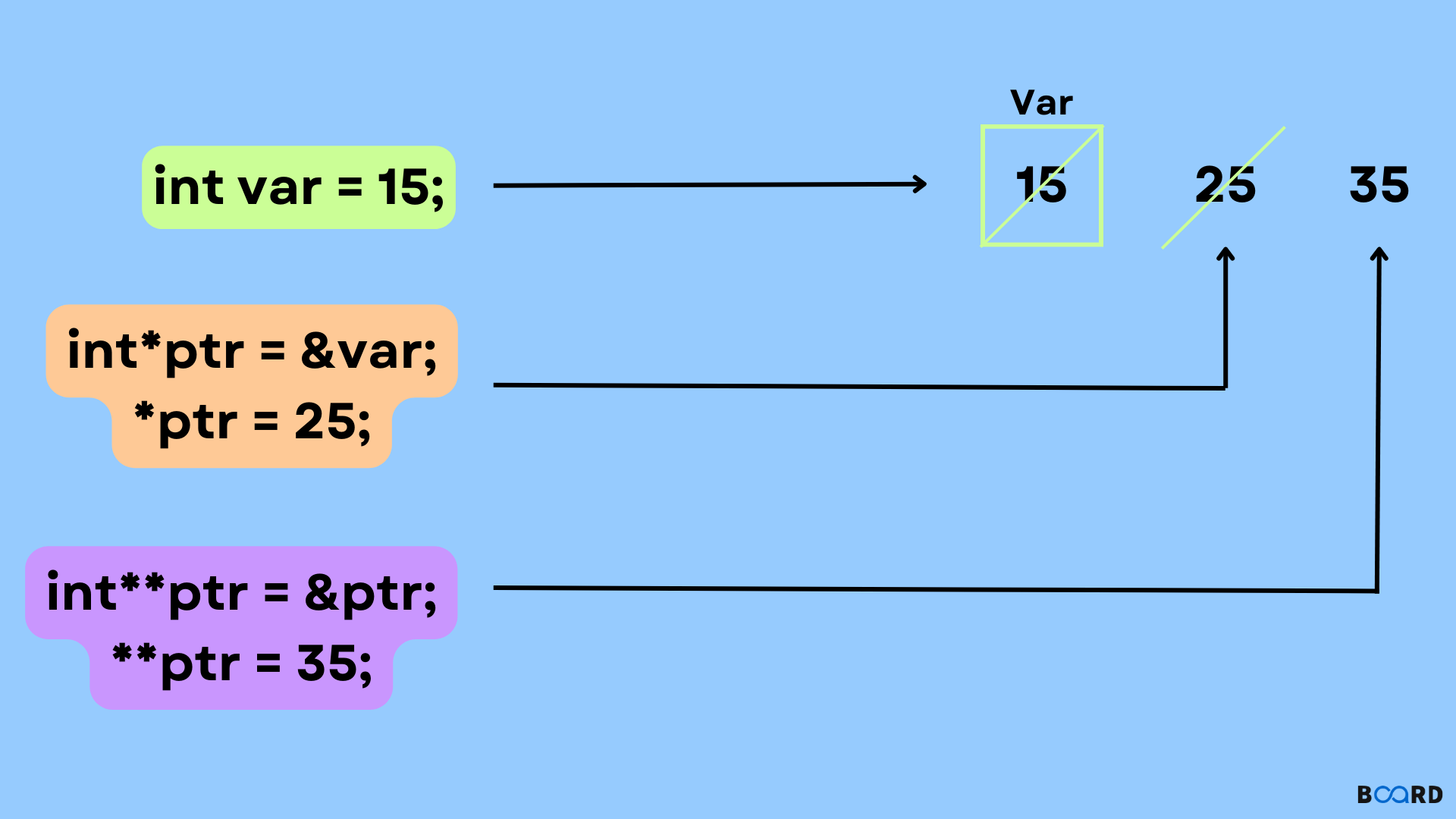
Introduction
Pointers is basically a variable that stores the address of some other variable. They are very powerful as they can create data structures dynamically and also can be used simulate call-by-reference. Another main use of pointers is used literate over array elements. A pointer can be declared using the following syntax:
Syntax:
Example:
How pointers can be used
- The very first step is to define a variable.
- In order to assign the address of a variable to another variable, the (&) unary operator is used that returns the address of the variable whose address is to be assigned.
- We can retrieve the value from the pointer variable using (*) unary operator.
Now let us consider the following program that illustrates the working of pointers:
Source Code
Output:
Output Description:
As you can see in the output, the address and the corresponding value have been displayed to console.
Passing values as a pointer
We can pass values as a pointer. The values in the pointer value can be changed by first dereferencing the pointer variable and assigning new values to it.
Consider the following program illustrating the working of call by pointer method:
Source Code
Output:
Output Description:
As you can see, the values of myInteger1 and myInteger2 have been modified to 20 and 30.
Array name as pointers
The array name possesses the address of the very first element of the array. It means that if we have an array as arr then using both arr and &arr[0] are the same thing.
Source Code
Output:
Advantage of pointers
- Pointers are quite helper as they reduce the code size and improves the performance.
- The allow us to construct trees or graph like data structure.
- Multiple values can be returned using pointers.
- Using pointers we can access memory location in computer’s memory.
Conclusion
In this article, we discussed about pointers. We mainly talked about passing pointers to function and manipulating array values using pointers. We believe that this article has helped to enhance your knowledge of pointers.