Java Advanced Topics and Techniques
Encapsulation in Java: A Comprehensive Tutorial
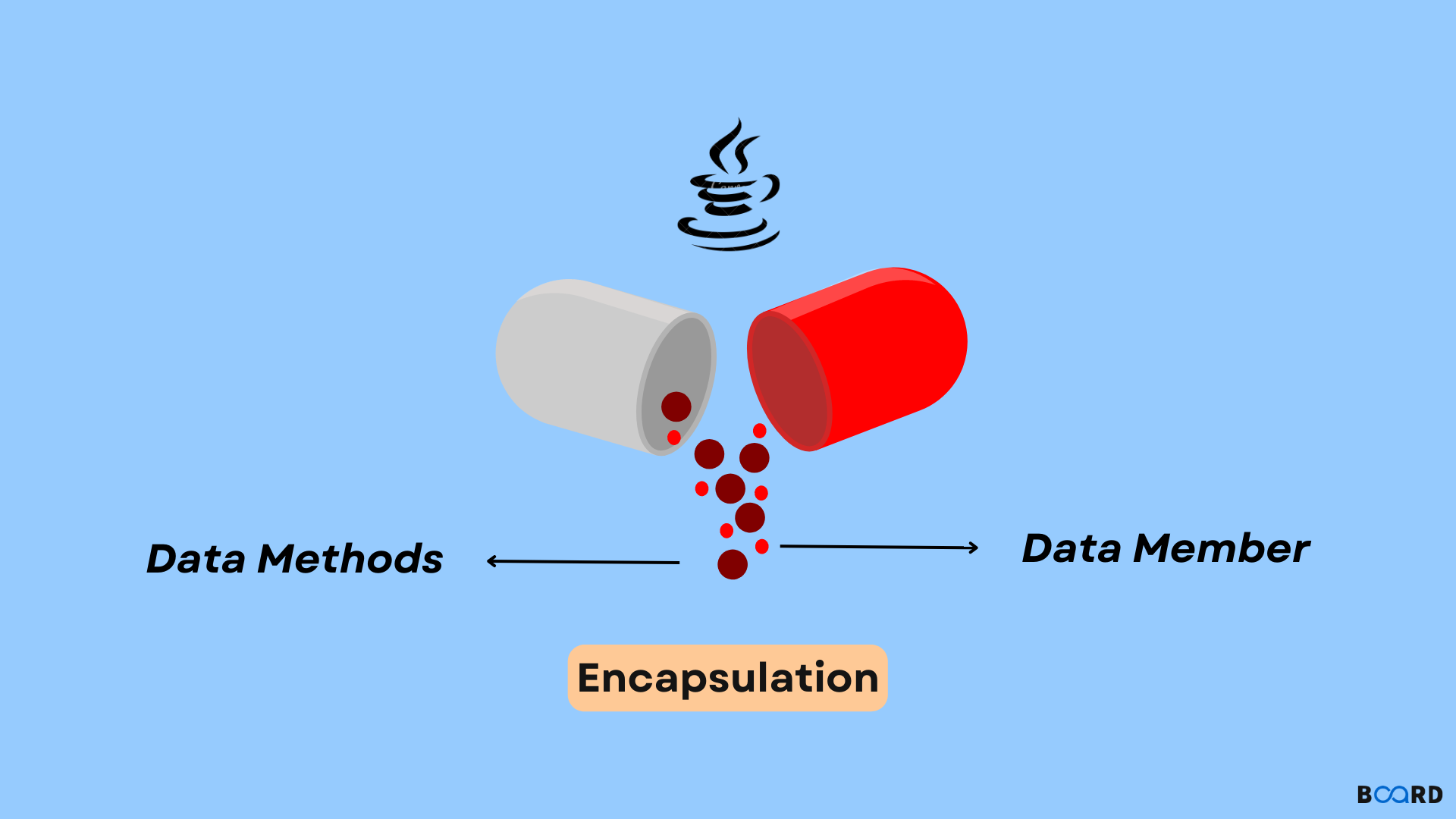
What is Encapsulation in Java?
Java encapsulates both variables (data) and code (methods). Encapsulation is the process of hiding variables from other classes and making them accessible only through the methods of their own class.
In Java, encapsulation involves combining data members and methods in a class with your own data. Private declarations are essential for this class.
After that, we will discuss the Java syntax that should get followed when encapsulating.
Syntax
Encapsulation Example in Java
The following sample program will help you better understand the encapsulation process.
Example:
Advantage of Encapsulation in Java
- If you only provide a getter or setter method, you can make the class read-only or write-only.
- You can control the data with it. You can implement logic inside the setter method if you want to set the id value to be greater than 100. The setter method can get modified to exclude negative numbers from storage.
- In Java, private data members prevent other classes from accessing the data.
- Encapsulate classes are easier to test, so they are better for unit testing.
- In Java, encapsulated classes can get created quickly and easily using the standard IDEs that generate getters and setters.
Getter and Setter Methods
Object-oriented programming languages commonly use getter and setter techniques. A getter method retrieves an attribute, while a setter method modifies it, as indicated by their names. The implementation method determines whether the attribute may be read and updated. Moreover, you can hide the attribute entirely or make it read-only.
Abstraction vs. Encapsulation
Abstraction and encapsulation get frequently misunderstood. Lets study-
Rather than "How" to achieve functionality, encapsulation is about "What" to do, while abstraction focuses more on "What" a class can do.
To understand this difference, let's take a look at a mobile phone as an example. An interface provides an abstraction of the circuit board logic by encapsulating it in a touch screen.
Conclusion
The Java encapsulation principle describes how data and methods that interact with it combine into a single unit. It often serves to conceal sensitive information. In this way, specific attributes can be protected from external access while still being accessible through public getter and setter methods by members of the current class. The methods allow you to specify which attributes can read or update and to validate values before updating them.