C Programming: From Basic Syntax to Advanced Concepts
One Dimensional Array in C
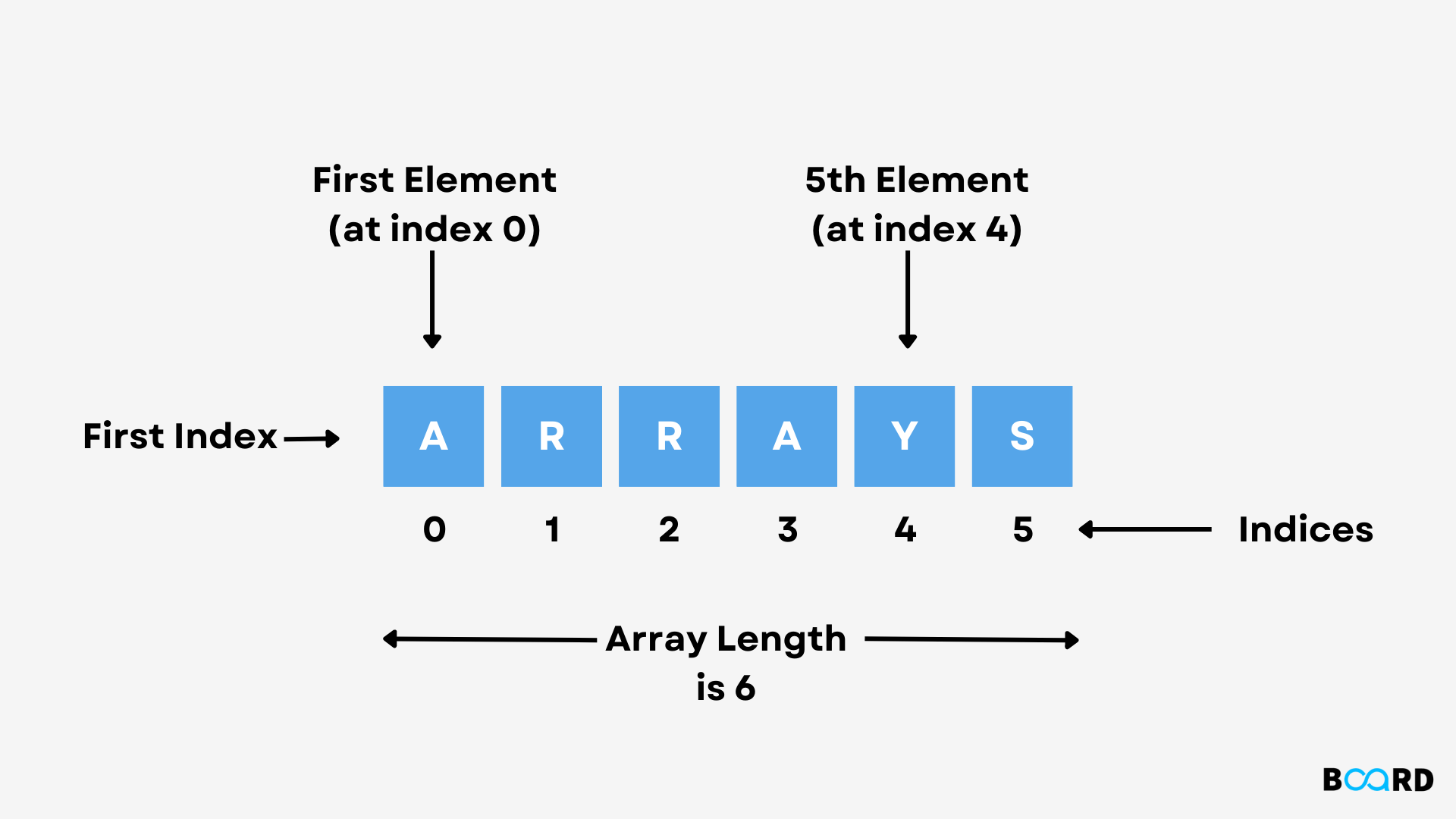
What in C is a one-dimensional array?
An array with one dimension has one subscript. In C(1D), a one-dimensional array is one that is shown as either one row or one column.
The vectors are one-dimensional arrays.
In other words, as shown in the following figure, it can be represented as a single dimension, either width or height:
Declaration of an Array in One Dimension in C
The declaration of a one-dimensional array in C is:
Syntax
Memory is allocated for the size elements. In a 1D array, the first element's subscript is 0 and the size of the last element's subscript is -1. The size of a 1D array must remain consistent.
For instance:
char str[100], int rollno[100],
The compiler sets aside or allocates memory to store the complete array when it is specified. y[5J is the sixth element since in C, subscripts always begin with 0 and increase by 1.
Instead of using a fixed integer quantity, it can be more convenient to express an array size in terms of a symbolic constant.
This makes it simpler to change an array-using programme because any references to the maximum array size (for example, in for loops and array definitions) can be changed by simply altering the symbolic constant's value.
For instance:
#define SIZE 100.
charr string [SIZE];
Assume we have the array int score[7] depicted in the diagram below:
The first element's address serves as the array's name, while the offset serves as the subscript.
Score's base address or starting address is 1000 (also known as score[0address), ]'s and the size of an int is 2 bytes. The address of the third element (score([2]) will be 1004, and so on, with the address of the second element (score([1]) being 1002).
Therefore,
The total array size in bytes is = The sum of the array's size and the datatype's size.
Example:
int a[40]; 402 bytes total, or 80 bytes. 50 bytes for character b; 501 total bytes.
double sal[20]; total bytes are (20*8), or 160 bytes.
The formula is: base address + (subscript * size of datatype) to get the address of any element in an array.
For instance, let's say the base address of A is 1000 (int A[40]). To obtain the address of A[5] element, multiply 1000 by (5*2) to get 1010.
Initializing One Dimensional Array in C
One-dimensional arrays can be initialized in C both during compilation and during runtime.
Initialization at Compile Time:
When an array is declared, it can be initialized. This is also referred to as initialization at build time.
When an array is declared, its elements can be initialized in the same manner as regular variables. Initializing an array often has the following form or syntax:
(a) Initializing all designated memory locations:
When an array's initial values are known before it is declared, it can be initialized. Data objects of type int, char, etc. can be used to initialize array elements.
Examples:
int a[5]={1,2,3,4,5};
The compiler reserves 5 contiguous memory spaces for the variable a during compilation, and all of these areas are initialized as indicated in the picture below.
(b) Partial Array Initialization:
The C programming language supports partial array initialization. if the array's size is smaller than the amount of values that need to be initialized.
The elements will then be initialized to zero automatically in the case of a numeric array and to space in the case of a non-numeric array.
For instance, int a[5]=0;
(c) Initialization without Size:
Take into account both the initialization and the declaration.
Example:
char b[]='C', 'O', 'M', 'P', 'U', 'T', 'E', 'R’
Even though the precise amount of elements to be used in array b is not stated in this declaration. The total number of initial values supplied will be used to determine the array size. As a result, the array size will be automatically set to 8.
The initialization of the array b is depicted in the figure below:
Run Time Initialization:
An array may get explicit run-time initialization. Large arrays are typically initialized using this method.
For instance, an array can be initialized with scanf().
int x[2]; scanf(“%d%d”,&x[0], &x[1], );
With the values entered via the keyboard, the aforementioned statements will initialize the elements of the array.
Accessing One Dimensional Array Elements
Using the array name and index within a pair of square brackets, we can retrieve a one-dimensional array element. Recall that array indexing begins at 0. N-1 is the index of the array's Nth element.
As an illustration, let's say we have an integer array with the name marks and a length of 5.
if marks[5] = {1, 2, 3, 4,};
Using subscript and the array name, we can now access the elements of the array points.
(1). points[0] = First element of array points=1
(2). points[1] = Second element of array points=2
(3). points[2] = Third element of array points =3
(4). points[3] = Fourth element of array points=4
(5). points[4] = Last element of array points =5
Giving array elements values:
Using the assignment operator, values can be assigned to specific array items.
array name[index]=value is the syntax.
For instance, a[0]=10, a[4]=100.
By only taking one element to another array, we cannot replicate all of the elements of one array to the second array:
As an illustration, if we have the two arrays a[5] and b[5],
This equation is invalid: int a[5]={1,2,3,4,5}; int b[5]; b=a.
Program 1: Create a programme to print an array's element sum
Code
Output
Program 2: This programme will locate the elements in an array that are highest and lowest
Code
Output: