Fundamentals of Python Programming
Python For Loop
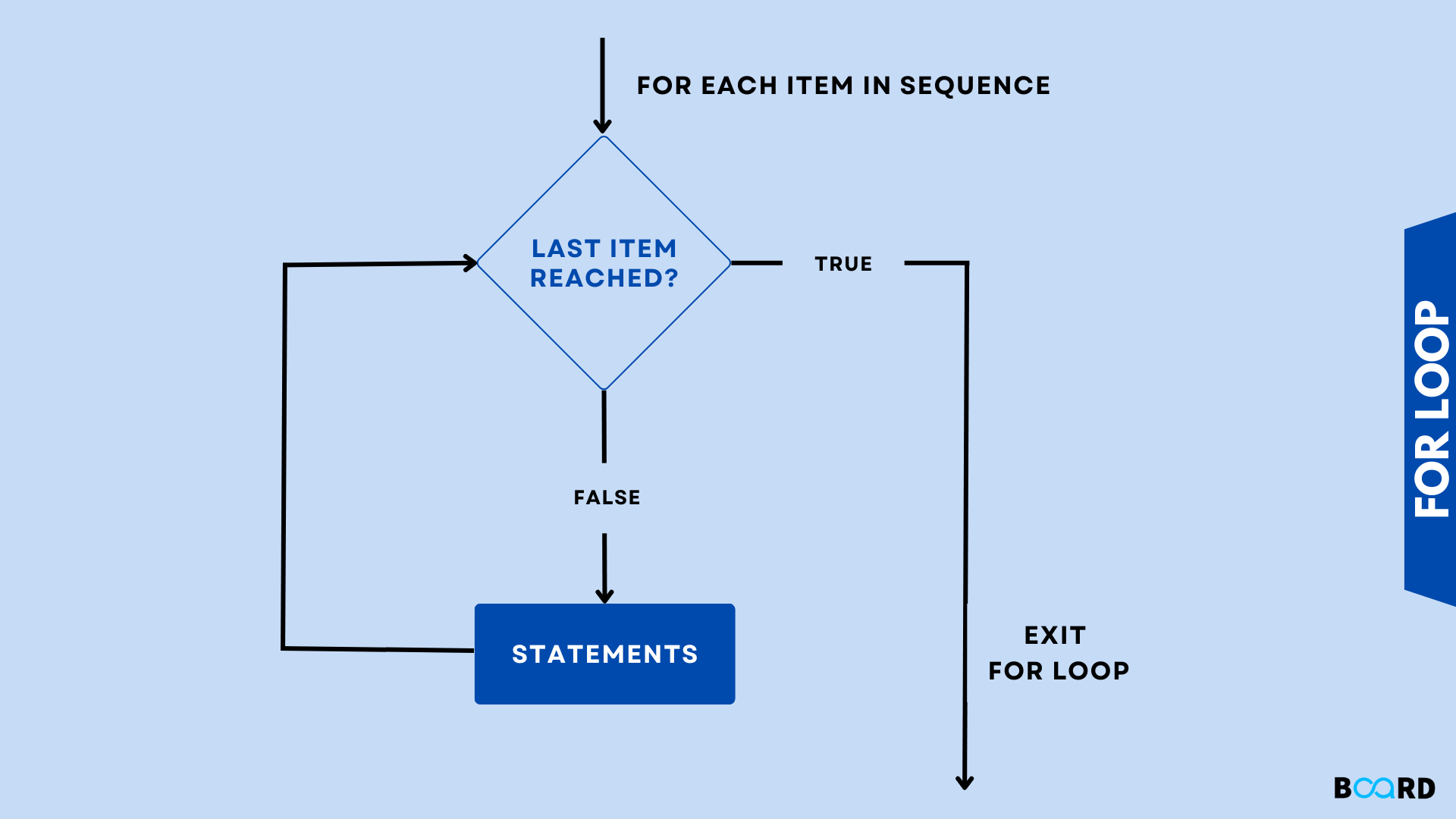
Introduction
Similar to the while loop, the for loop is an iteration statement in programming language that permits a code block to be repeated a predetermined number of times. There aren't many computer languages that don't include for loops, but they come in a variety of flavors, meaning that each programming language has a different syntax and semantics.
Syntax of the For Loop
A for loop based on an iterator is the Python for loop. It iteratively traverses the elements of lists, tuples, strings, dictionary keys, and other iterables. The word "for" precedes the name of an arbitrary variable in the Python for loop, which will retain the values of the subsequent sequence object that is iterated over. The basic syntax is as follows:
The sequence object's elements are assigned one by one to the loop variable, or more precisely, the variable is set to point to the items. The body of the loop is run for each item.
A simple Python for loop illustration
OUTPUT:
The for loop will be terminated and the programme flow will continue with the first line after the for loop, if any, if a break statement has to be performed in the for loop's programme flow. Break statements are often included in conditional statements, for example.
OUTPUT:
Taking "spam" out of our list of foods will have the following result:
Perhaps our dislike of spam is not as strong as our desire to quit eating other foods. This now activates the continue statement. When we hit a spam item in the script below, we use the continue statement to continue with our list of edibles. So keep going to stop us from consuming spam!
OUTPUT:
The range() Function
The best way to repeatedly iterate through a list of integers is the built-in function range(). It produces an iterator of mathematical progressions, such as: Example:
OUTPUT:
This result does not make sense on its own. It is a thing that can generate numbers ranging from 0 to 4. You will understand what is meant by this when we use it in a for loop:
OUTPUT:
The function range(n) creates an iterator that advances the integer numbers from 0 to n. (n -1). We must cast range() with list() to create the list containing these integers, as shown in the example that follows.
OUTPUT:
range() can also be called with two arguments:
The call mentioned above generates a list iterator of integers beginning with begin (inclusive) and ending with a number that is one less than end.
OUTPUT:
Range() has incremented by 1 thus far. With a third input, we are able to define a different increment. The step is the name for the increase. It can be either positive or negative, but it can't be zero:
OUTPUT:
You may also do it the other way around:
OUTPUT:
As we can see in the example below, the for loop and the range() method work very well together. The for loop uses the values from 1 to 100 supplied by the range() function to calculate the total of these integers:
OUTPUT: