Learn about Thread Sleep Method in Java
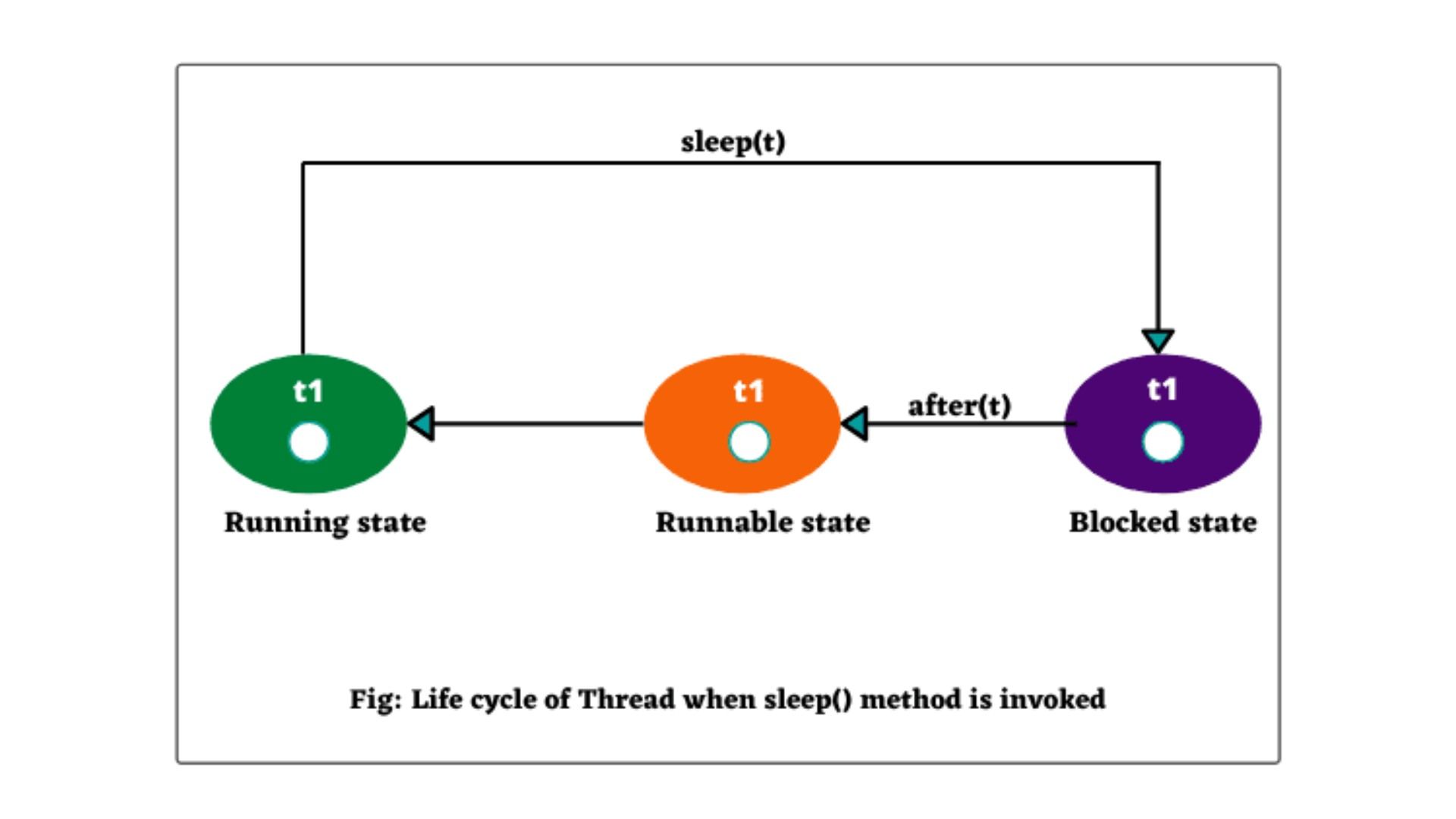
Introduction
The Java Thread.sleep() method allows you to pause a thread's execution for a specified period in milliseconds. Negative values are not allowed for milliseconds. Otherwise, it will throw an IllegalArgumentException.
sleep(long millis, int nanos) is another method for pausing thread execution for a specific time. The values of nanoseconds must fall between 0 and 999999. This article will explore Java's Thread.sleep ().
How Thread.sleep Works
Invoking Thread.sleep() pauses the current Thread by interfacing with its scheduler for a specified time. As soon as the wait time is over, the thread state gets changed to runnable, and it waits for the CPU to execute it.
A thread scheduler, part of the operating system, determines how long the current Thread sleeps.
The syntax for Thread.sleep():
Here is the syntax for Thread.sleep() method.
Here,
millis: Thread sleep duration in milliseconds
nanos: Additional duration specified in nanoseconds.
A Few Points to Remember About Sleep()
- Executing the thread.sleep() methods will result in a halt for the current thread execution.
- The program throws an InterruptedException exception when another thread interrupts the current thread in sleep mode.
- As the thread runs on a busy system, its sleeping time is generally longer than its arguments. In contrast, if the system executing sleep() has a lower load, the thread will sleep almost as long as the argument.
Code Sample
Negative Sleep Time Throws an IllegalArgumentException