JavaScript Fundamentals: Learning the Language of the Web
What are Sets in Javascript?
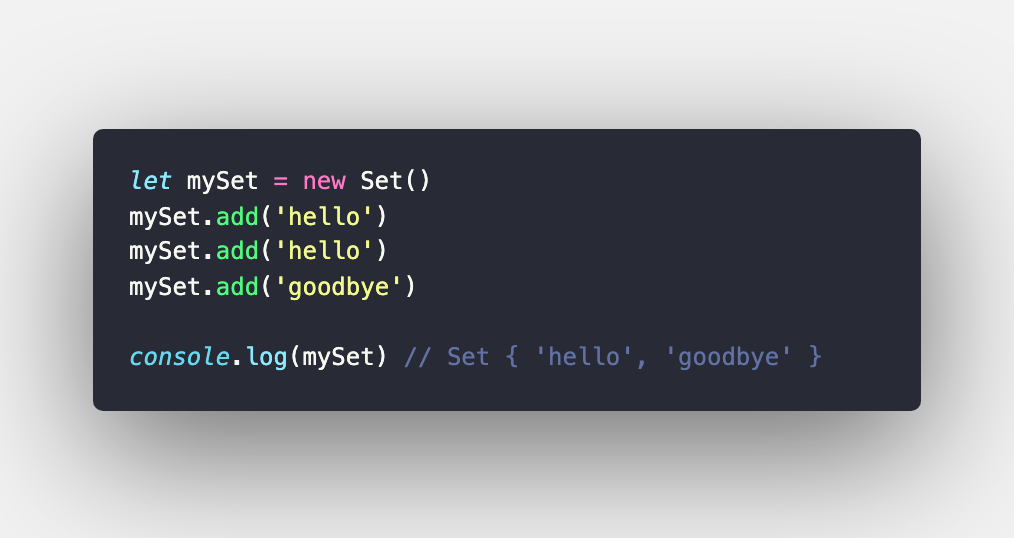
Overview
A set is an object in ES6 that enables a programmer to create a collection of distinct values of any data type. We can add as many items in a set as we wish to, but it will automatically discard any duplicates. In this article, we shall learn in-depth about Set in JavaScript, its uses, how it can be used, and many other cool things!
Scope
In this article, we shall learn about:
- How to create a Set in JavaScript.
- Some useful methods that Set provides.
- What is WeakSet in JavaScript?
How to create a set?
The following code block shows how to construct a set object:
We can also pass an iterable object as an argument to the SET() constructor to initialize our set object with the elements of the argument object. Have a look at the code block ahead:
Output
Set methods and properties
size
The size property of Set holds the number of items in the Set object. This is how we use it:
Output
add
It is a method that allows us to add elements to a set object. Use it as demonstrated in the following code block:
Output
We can serially chain multiple add calls as follows:
has
has() is a method that returns a boolean value, which determines whether or not a certain value is present in the set object. Pass the item to be checked the presence of, as an argument to the has() call. Look at the code block ahead to have a better understanding of how has() works;
Output
delete
It is a method used for removing an element from a set object.
Output
The method “delete” returns true implying that the element has been successfully removed from the set object.
clear
Use clear() method to empty a set object.
Output
entries
In JavaScript, we are also provided with the keys(), values(), and entries() functions. Though, the keys and values in a javaScript set object are the same.
Output
WeakSets
A WeakSet in JavaScript is similar to a normal Set. The only difference is that a WeakSet may contain only objects. The objects stored in a WeakSet object are automatically garbage-collected, and thus a WeakSet object does not have the property “size”. Since one can’t iterate the elements of a WeakSet, it is rarely used in practice. Check out the code snippet below:
Output