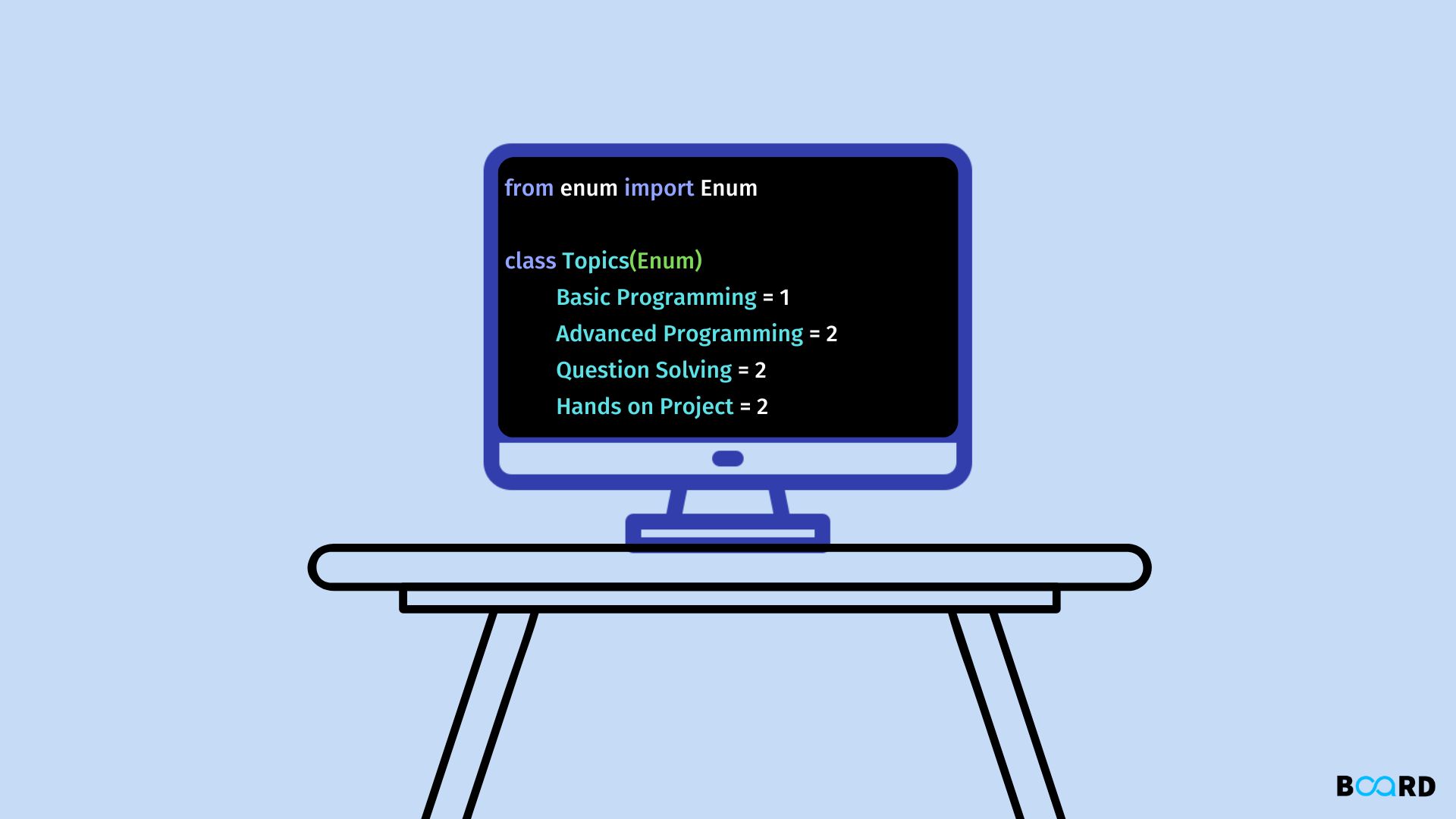
Introduction
A data collection object is transformed into an enumerate object using Python's Enumerate method. It gives back an object with a counter serving as the key for each value. This facilitates access to collection items and enables us to keep track of how frequently we have used them. A suite of utilities called Enumerate enables you to iterate through a set of data once for each item. Each item is coupled with a number that represents how many times the loop has run as the function iterates across the object.
We'll just cover two of the four data types available in Python for storing information collections in this example. Dictionary and list data types are ordered, therefore they often don't need to go through the enumerator function (with the exception of complicated programming, like machine learning). Sets and tuples, on the other hand, are unordered and hence more likely to be enumerated.
Python Enumerate Example
Accessing and counting objects in a data collection is made simpler by the Python enumerate function. In this section, we'll examine instances of the enumerate function using the set and tuple data types. Python has four major data collection types. We'll examine these two different but useful examples of the enumerate function in the following section. Let's quickly review the two data types we'll be using and how they differ from one another.
- Tuple: Arranged and Unalterable. Allows for multiple members.
- Set: Unindexed, unordered, and immutable (things may be added or deleted but not modified). Exactly one member alone.
Enumerate Python Sets
Python sets are collections of unsorted data whose elements are immutable, meaning they cannot be altered after being added. Since sets are by definition unordered, the order in which their components are accessed varies, and since they lack an index value, they cannot be targeted by it. Each item in the set is given a counter by the enumerate function as a key. This can aid in giving an unordered collection structure. In order to interact with the set in a trustworthy manner, we have greater control by enumerating it. This is helpful with sets since the elements within them lack a specific index location.
The targeting of an index, which is incompatible with sets, causes the code above to throw an error stating a set is not subscriptable. The counter may be used as an index to locate our item, though, if we enumerate the set first.
Although the order in which the items are returned may differ, the code above would print the following result to the console.
It's crucial to notice that this does not change the value of snekSet; publishing it to the console will instead display the same set that we declared, most likely in a different order.
You will need to change the results' data type in order to maintain the enumerate function's impact on a set. Let's take a look at an illustration of how to use the list to accomplish that.
We first construct the set, then we use the set to execute the enumerate function and save the results to a new variable. The outcome of the enumeration is then given to the list and stored as a new list object. This new setlist object's contents would be organized as a list of key-value pairs.
The problem is that it results in a complicated data structure, and as sets are unpredictable, caution should be used while using them. Let's examine tuples as a different use of the enumerate method.
Enumerate Python Tuples
To obtain key/value pairs from tuples, the enumerate function can be used; this method offers considerably greater assurance about the return order of the items. Tuples are ordered, which means that the elements within them are in the order in which they were inserted. This is the rationale behind it. In contrast to a set, a tuple can target the third item by using the bracket notation and index 2.
Comparing counting sets to counting Python tuples, the former is easier. Let's examine an example of enumerating over tuples in Python to see how much more predictable and consistent the outcomes are.
The result of the loop will be written to the console after each iteration, and it will include a list of the snekTuple's produced keys and values.
This example is somewhat redundant because tuples are sorted and may be specifically targeted using indexes, it is crucial to highlight. This is not to suggest that there aren't valid reasons or uses for this; enumerating over a dictionary of sets is an excellent example. To better grasp the dictionary's contents, the sets within it might be listed off.