Get a list as input from the user
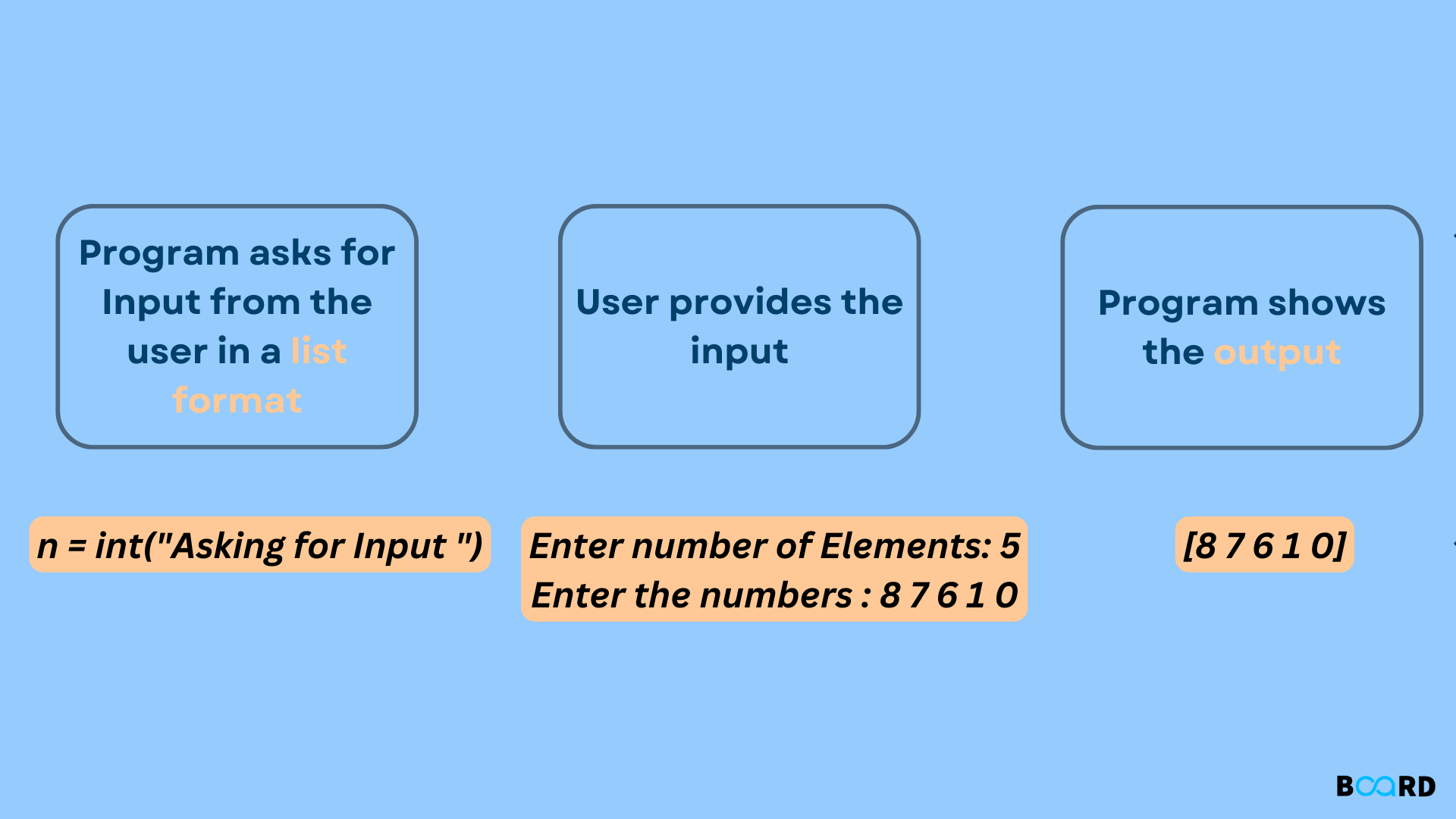
Introduction
Now in day-to-day programming, we need to take numbers or strings as input. Now in Python, we can take input from the user by using the input keyword. Let us see how can we input a list from the user.
Example
Taking list as input from the user
Lists: In Python, just like java and c++, Lists are just like dynamic arrays. A list is a collection or pack of items. Lists are enclosed in square brackets i.e. [] and all its elements are separated by commas.
Code
Output
Taking list as input from the user with handling exceptions
Exceptions: In Python, when a cod is correct syntactically but gives an error while running, then exceptions are raised. Now, this error or the exception does not stop the execution of the program, the program still executes, but the exception will change the flow of the program.
Code
Output
Taking a list as input from the user using the map
map(): In python, the map is a function. This method returns a map object of the results after applying this method to every item of iterable such as a list, tuple etc. This map object is an iterator.
Code
Output
Taking list of lists as input
Code
Output
Taking input by using List Comprehension and Typecasting
list comprehension: In python, list comprehension is used to execute some expression while iterating over each element of a list. It contains brackets that contain the expression, and that expression is executed for each of the elements of the list.
Code
Output