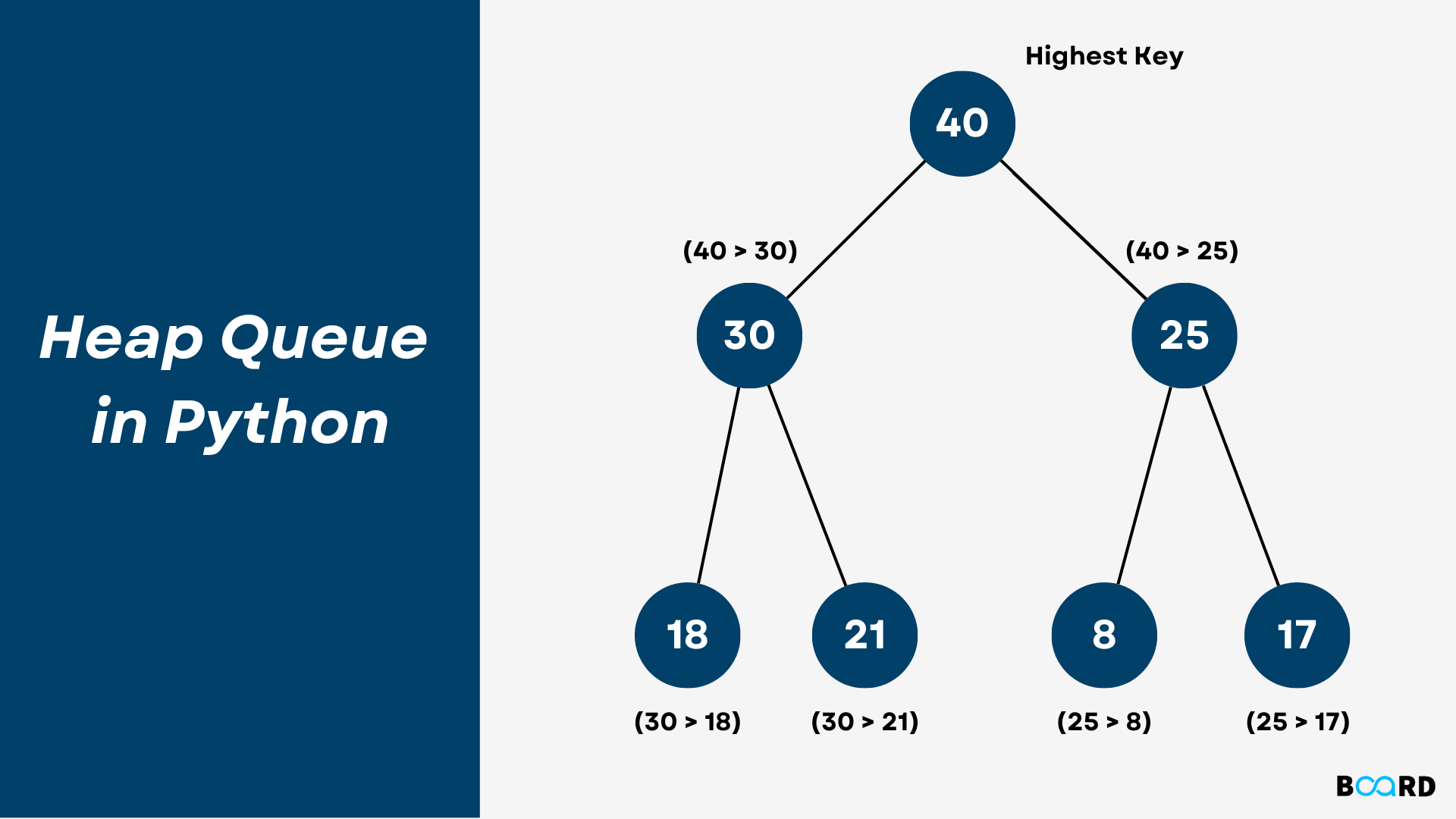
Introduction
Heap is a data structure, that is mainly used to represent a priority queue. In Python, it is available by importing the heapq module. Heapq has a property that every time the smallest heap element is popped (min-heap). Each time when an element is pushed or popped the heap structure is maintained. Also when we use heap[0], it returns the smallest element. In this article, we will see different operations on the heap in python.
Creating a simple heap
Heapify(iterable) is a function that converts an iterable list into a heaping order.
Code
Output
write your code here: Coding Playground
Appending and Popping Items Efficiently
- heappush(heap, ele): heappush is a function that takes an argument which the element and inserts that element into the heap. The heap order is adjusted and the heap structure is maintained.
- heappop(heap): heappop is a function that removes the smallest element in the heap and also returns it. This heap order is again adjusted and the structure is maintained.
Code
Output
write your code here: Coding Playground
Appending and Popping simultaneously
- heappushpop(heap, ele):- This function combines the functionality push and pop operation inside the statement, thus increasing the efficiency. Heap order is maintained after this operation.
- heapreplace(heap, ele):- This function also does the same functionality but is different from the above function. In this function, the element is first popped and then the element is pushed. that means the value larger than the pushed element can be returned. This function returns the element that is smallest ignoring the pushed element as opposed to heappushpop().
Code
Output
write your code here: Coding Playground
Find the largest and smallest elements from Heap in Python
- nlargest(k, iterable, key = fun): This method is used when we need to return the k largest elements from the iterable specified and also satisfy the key if mentioned.
- nsmallest(k, iterable, key = fun): This method is used when we need to return the k smallest elements from the iterable specified and also satisfy the key if mentioned.
Code
Output
write your code here: Coding Playground