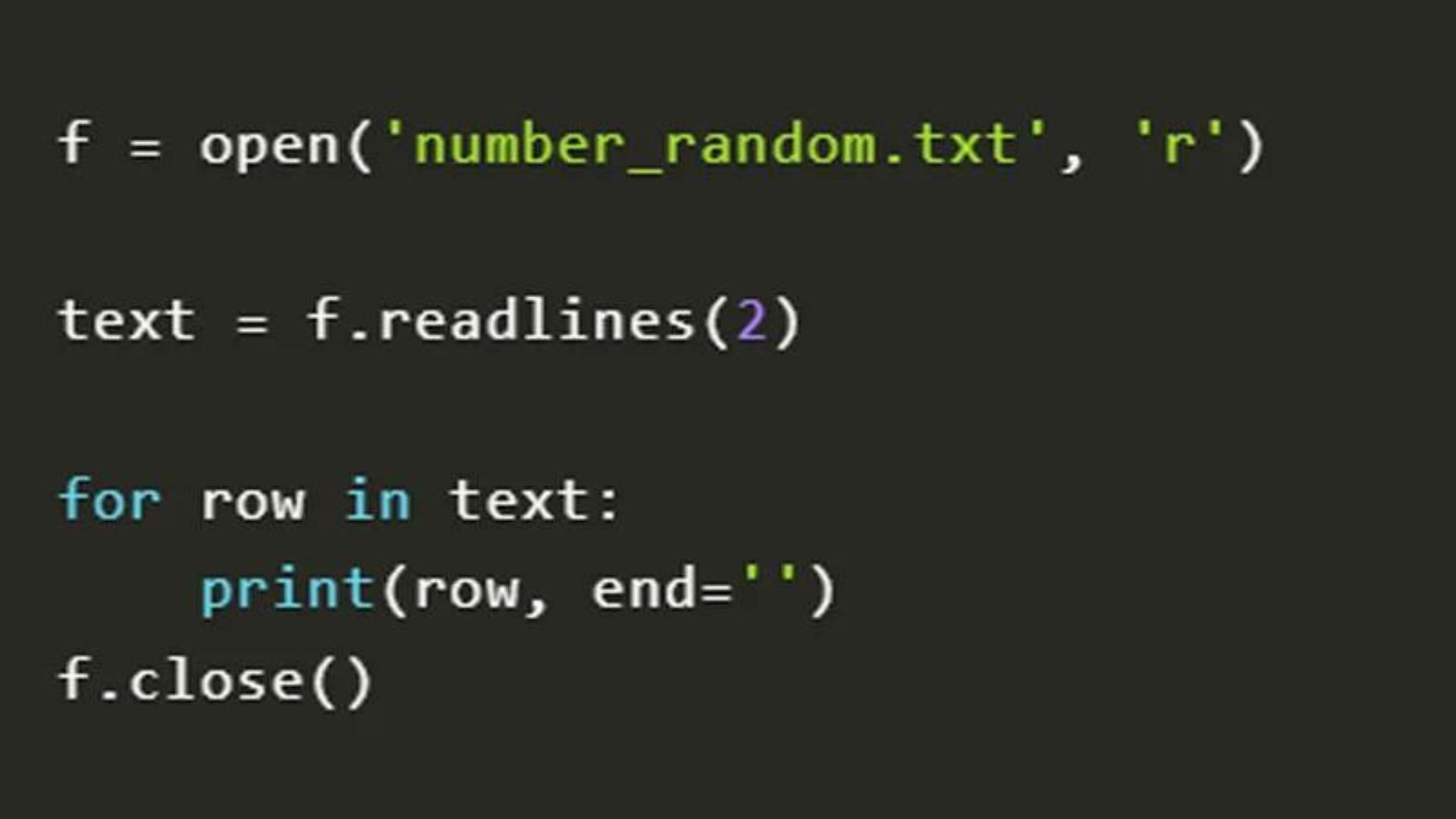
Introduction
You will learn the fundamentals of what readlines() is and performs in this post. Additionally, we will look at the hint parameter's impact on readlines (),IOError associated within readlines, code examples for file reading, etc. Python's readlines() method allows us to read every line at once and returns the results as a string element. Readlines() will be the go-to function whenever we need to extract the text from any file.
Syntax
The syntax for readlines is
Each line is returned as a string item in a list by the method readlines(), which reads all lines simultaneously. We may go over each member of the list using readlines(), and then use the split() function to remove characters. Since this approach reads the contents of the full file into memory before separating them into separate lines, it may be used with small files.
Parameters
- The readlines() method has a single hint parameter, which is reserved for exceptional circumstances and is not often used.
- No more lines will be returned if, while using hint, more bytes are returned than the hint number. If the value is set to default, all lines will be returned (-1).
Return Values
- Let's imagine you have a file called "myfile.txt" on your PC. The text file's entire contents will be returned by the readlines() function.
- This method returns a list that contains every line in the file.
Exceptions
- One of the few Python methods with a low number of runtime exceptions is readlines().
- The IOError is the sole exception that individuals occasionally encounter. Input/output error is referred to as IOError.
- It occurs when the file, file path, or OS activity that we are referring to doesn't exist.
- Both insufficient permissions and a missing file are covered by IOError.
The IOError for readlines() appears like this:
Example 1: Reading file lines using the readlines() function
The readlines() function will be used to print the lines that were written from the file in this example after we attempt to write into an empty file.
Code
Conclusion
Learning Python is quite basic and straightforward. It enables you to read, write, and display data from a variety of formats, such as text, Word documents, or CSV files. The function readlines() was created by Python to read data from a certain file all the way through and show any Python data on the output terminal. In this article, we looked at how Python's readlines() method operates. To show the lines of a text file in one row, we started with a very basic example. After that, using the strip() method, we created a way to show each line independently.