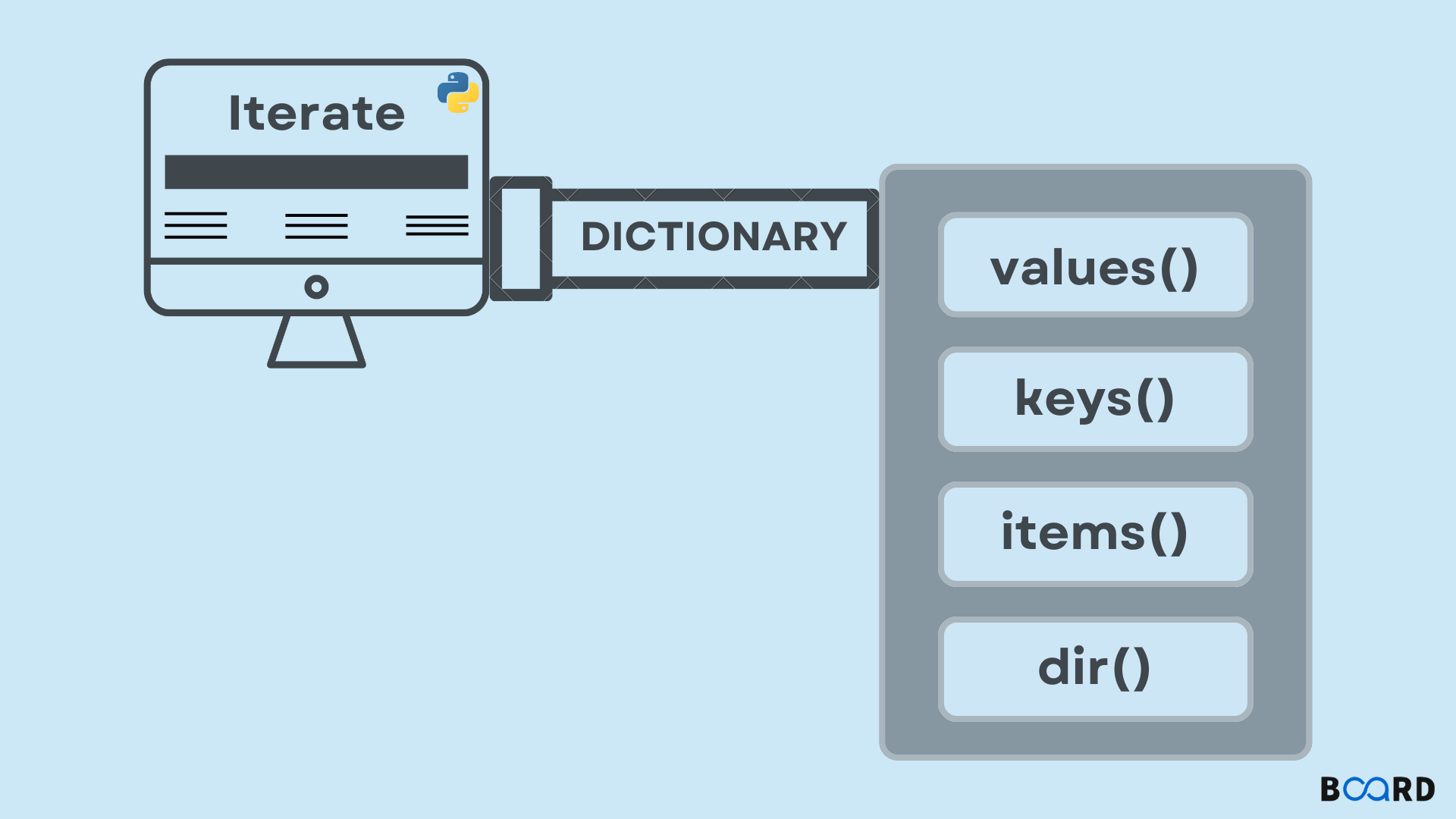
Employing the keys() Method
Python dictionaries include a convenient method called keys that makes it simple to loop through every initialized key in a dictionary ().
Remember that since Python 3, this function returns a view object rather than a list. Like its name implies, a view object is a representation of some data.
This means that while iterating through the data won't be a problem, storing the list of keys requires materialization. Which is simply accomplished by sending the view object provided to a list function Object().
Example :
Output:
Another approach might be:
When a dictionary is used in conjunction with the in keyword, the dictionary calls its __iter__() method. The iterator that this method returns is then used to implicitly iterate across the dictionary's keys.
Making use of the values() Method
The values() method returns a view object just like the keys() method does, but it iterates through values rather than keys :