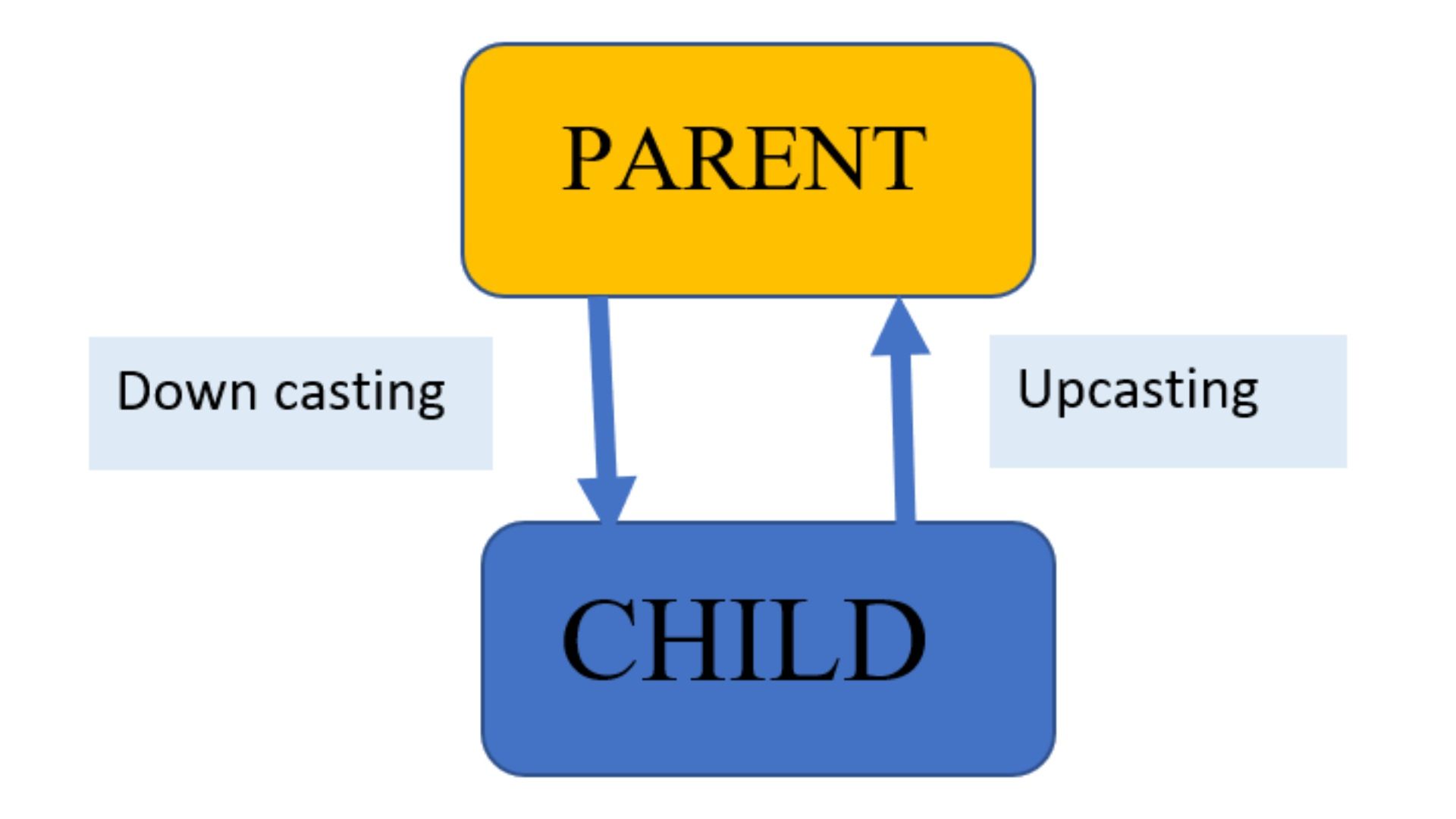
Introduction
The act of converting from one type to another is known as type casting. Type casting is a feature that permits the form or type of a variable or object to be converted into another sort of object. The various data types in java are char, int, short, long, float, double, etc. Similar to variables, objects can be type cast as well. There are only two sorts of objects, namely parent objects and child objects. Typecasting of objects, then, fundamentally refers to the conversion of one kind of objects, such as a child or parent, to another. Typecasting comes in two forms. As follows:
- Upcasting
- Downcasting
Upcasting
A form of object typecasting called upcasting involves typecasting a child object to a parent class object. We may simply access the variables and methods of the parent class in the child class by utilizing upcasting. Here, we just access a portion of the procedure and variables. Only a few specific child class variables and methods are accessed. Widening and generalization are other names for upcasting. Look at the example below:
Downcasting
Although it is not possible to give a parent class reference object to a child class in Java, if downcasting is used, there won't be any compile-time errors. The "ClassCastException" is thrown when we attempt to run it. The question is, why is downcasting permitted by the compiler if it is not feasible in Java? In some Java contexts, downcasting is an option. The parent class in this case refers to the subclass object. Here is a downcasting example :
CODE
write your code here: Coding Playground
OUTPUT
Why do we need Upcasting and Downcasting?
Internally, upcasting is performed, and as a result, an object may only access certain members (overridden methods) of the parent class and certain members of the child class.
Downcasting is an external process that allows a child object to inherit the parent object's attributes.
Upcasting Vs Downcasting
There are several very distinct distinctions between upcasting and downcasting. The following tips briefly highlight a few of them:
- A child object is typecast to the parent object during upcasting. On the other hand, when that relates to downcasting, the reference to the parent class object ultimately passes on to the child class.
- Downcasting can only be done directly, but upcasting can be done both explicitly and implicitly.
- People can access the variables and methods of the parent class in the child class in the case of upcasting, but not the other way around. But with downcasting, both classes' variables and methods are readily available. Accessibility to just specific child class methods is permitted by upcasting.