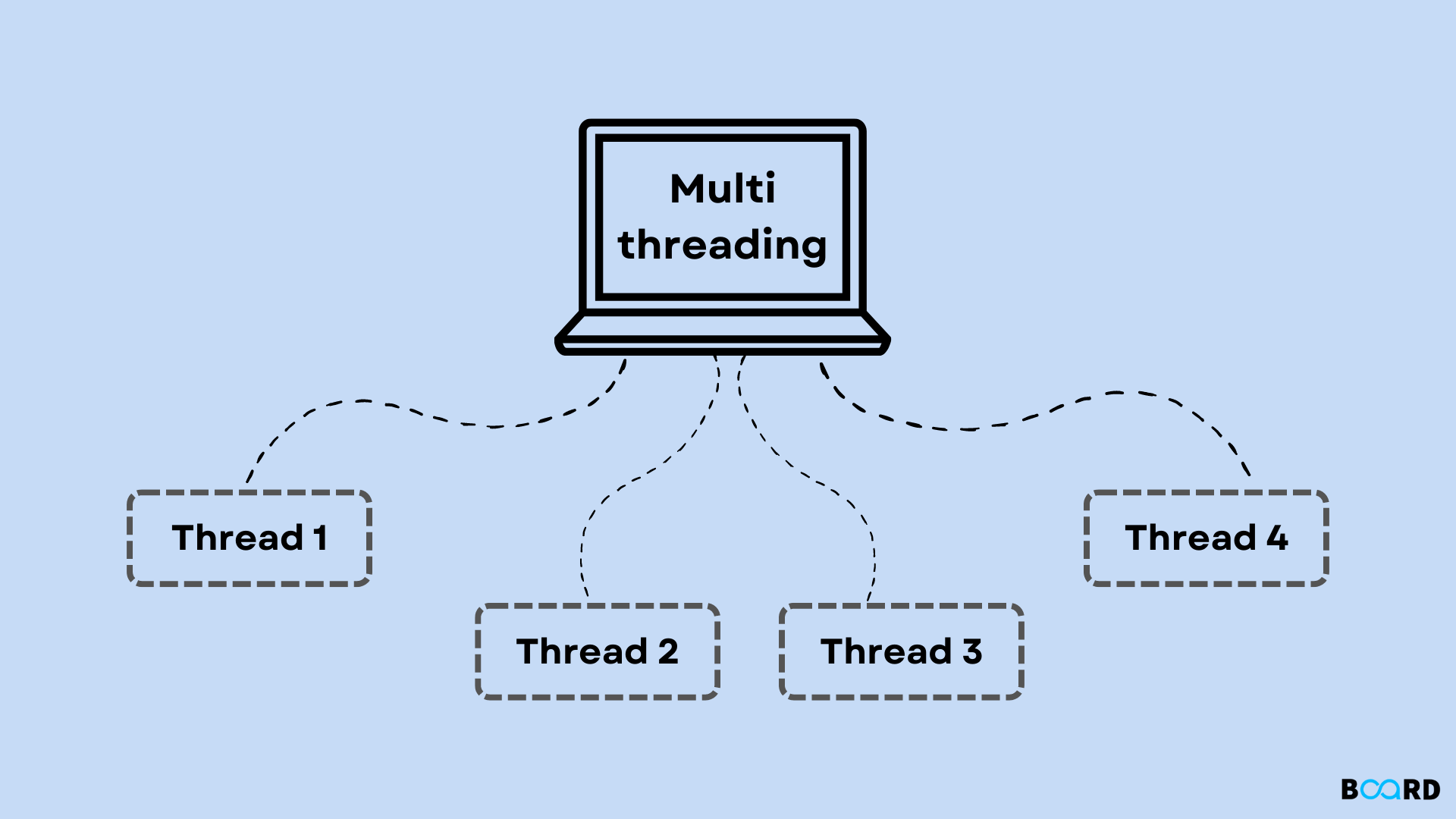
Introduction
Multithreading is the ability of a processor to execute multiple threads parallely. A thread can be defined as an entity within a process scheduled for execution. It is the smallest component of processing that can be performed in an Operating System. When multiple threads are being executed in a process at the same time, we call it using the term multithreading which is simply multitasking.
Thread
In computing, a process can be defined as an instance of a computer program that is being executed, a process can also be known as an active program that is under execution. It has three basic components :
- An executable program
- The associated data needed by the program(variables, workspace, buffers, etc.)
- The execution context of the program
A thread is the smallest unit of a process executed independently or scheduled by the operating system. In the computer system, an OS achieves multitasking by dividing the process into threads. A thread contains all the information in a Thread Control Block(TCB):
- Thread Identifier: Unique id is assigned to every new thread.
- Stack pointer: It indicates(points to) the thread’s stack in the process. The stack contains the local variables under the thread’s scope.
- Program counter: a register that stores the instruction address currently being executed by a thread.
- Thread state: The state of the thread can be running, ready, waiting, starting, or done.
- Thread’s register set: It is the set of registers that are assigned to the thread for computations.
- Parent process Pointer: A pointer to the Process control block (PCB) of the process that the thread prevails on.
Python Multithreading
Multithreading is a threading technique in Python programming to run multiple threads simultaneously by rapidly switching between threads with the help of the CPU (context switching). Multithreading aims to perform multiple tasks simultaneously, increasing performance and speed and improving the application's rendering.
Example code
Benefits of Multithreading in Python
- It ensures the effective utilization of computer system resources.
- Multithreaded applications are more responsive.
- It shares resources and its state with sub-threads which makes it more economical.
- It makes the multiprocessor architecture more effective due to parallelism.
- It reduces the time required by executing multiple threads at the same time.
- The system would be needless of too much memory to store multiple threads.
Conclusion
It is a very useful technique for time-saving and performance improvement of an application. Therefore multithreading enables more than one user at a time also it manages multiple requests from the same user.