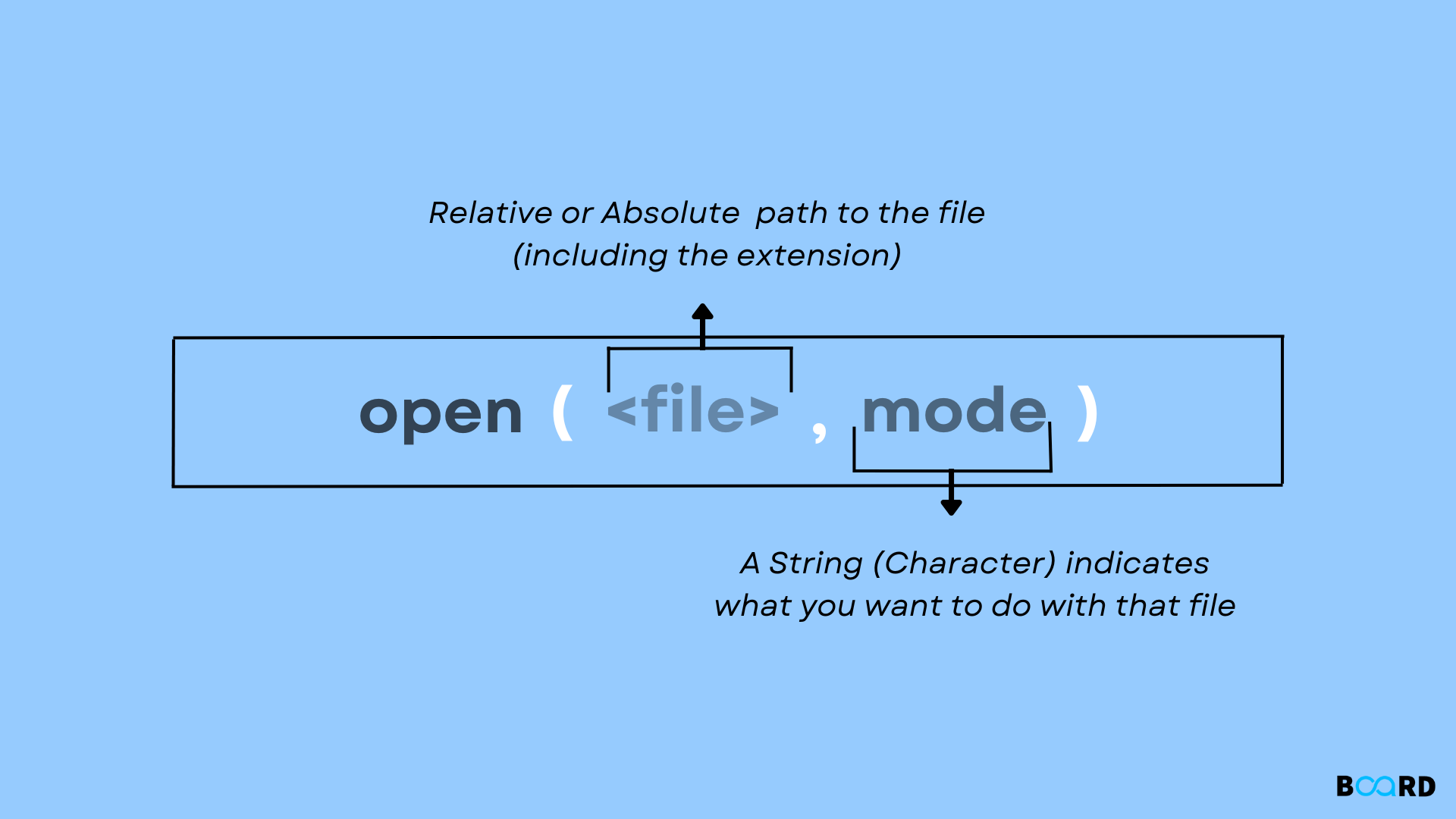
Introduction
Python's standard library includes file handling functions. This is extremely helpful for developers because it eliminates the need to import additional modules for file management.
Python's core file handling functions are open(), close(), write(), read(), seek(), and append() ().
Let's look at the open() method, which allows us to open files in different modes in Python.
All we need to open a file is the directory path where the file is placed. If it's in the same directory, just including the full filename will sufficient.
Opening a file using the open() method
Let's use the open() and read() methods to open OpenFile.txt and read its text contents.
The read() method will read the entire file's contents.
The open() method opens a file in read-only mode by default. To write to a file, we must require that the file be opened in write mode.
Different Modes For open() Method
Let's try writing to the file in the default mode.
We'll leave the read operation alone so we can see where the code ends.
So, what exactly are modes, and how can we incorporate them? When utilising the open() method, the following modes are available.
- r denotes read-only mode.
- r+: Read/write mode. Will not create a new file and will fail to open if the file does not already exist.
- rb: Read-only binary mode for reading photos, videos, and so forth.
- w: Read-only mode. Replaces existing file content. If the supplied filename does not exist, a new file will be created.
- w+: Read/write mode.
- wb: Binary write-only mode for media file writing.
- wb+: Binary read and write mode .
- a: The append mode. It does not replace current content.
- a+: Read and append modes. If the filename does not exist, it will be created.
- ab: Append binary mode for photos, movies, and other media.
- ab+: Binary append and read mode.
Opening Files in Write Mode in Python
In Python, there are several ways to open a file in write mode. You can use one of the following modes to direct how the file handling methods write to a file.
We declare that the file should be opened in write mode by including the 'w' in the first line. However, this operation would also fail because the file is read-only and will not allow us to use the read() method.
The code above will completely clear the text file's contents and instead say "New content."
Any content passed to the write() method will be written by the r+ mode.
With one major exception, the an or a+ mode will do the identical action as the r+ mode.
If the filename supplied does not already exist, the r+ method will not create a new file. If the specified file is not available, a+ mode will create a new file.
Opening Files Using the with clause
To avoid memory leaks when reading files with the open() method, always ensure that the close() method is called. As a developer, you may overlook the close() method, causing your programme to leak file memory due to the open file.
When working with smaller files, there isn't much of an impact on system resources, but it becomes apparent when working with larger files.
In the preceding example, the output would be the same as in the beginning, but we wouldn't have to close the file.
A with block obtains a lock as soon as it is performed and releases the lock when the block is completed.
While staying within the with code block, you can also run other methods on the data. In this situation, I changed the OpenFile.txt file and added some additional wording for clarity.
Conclusion
You should now understand how to open a file in Python and how to handle the many modes for opening a file with the open() method.