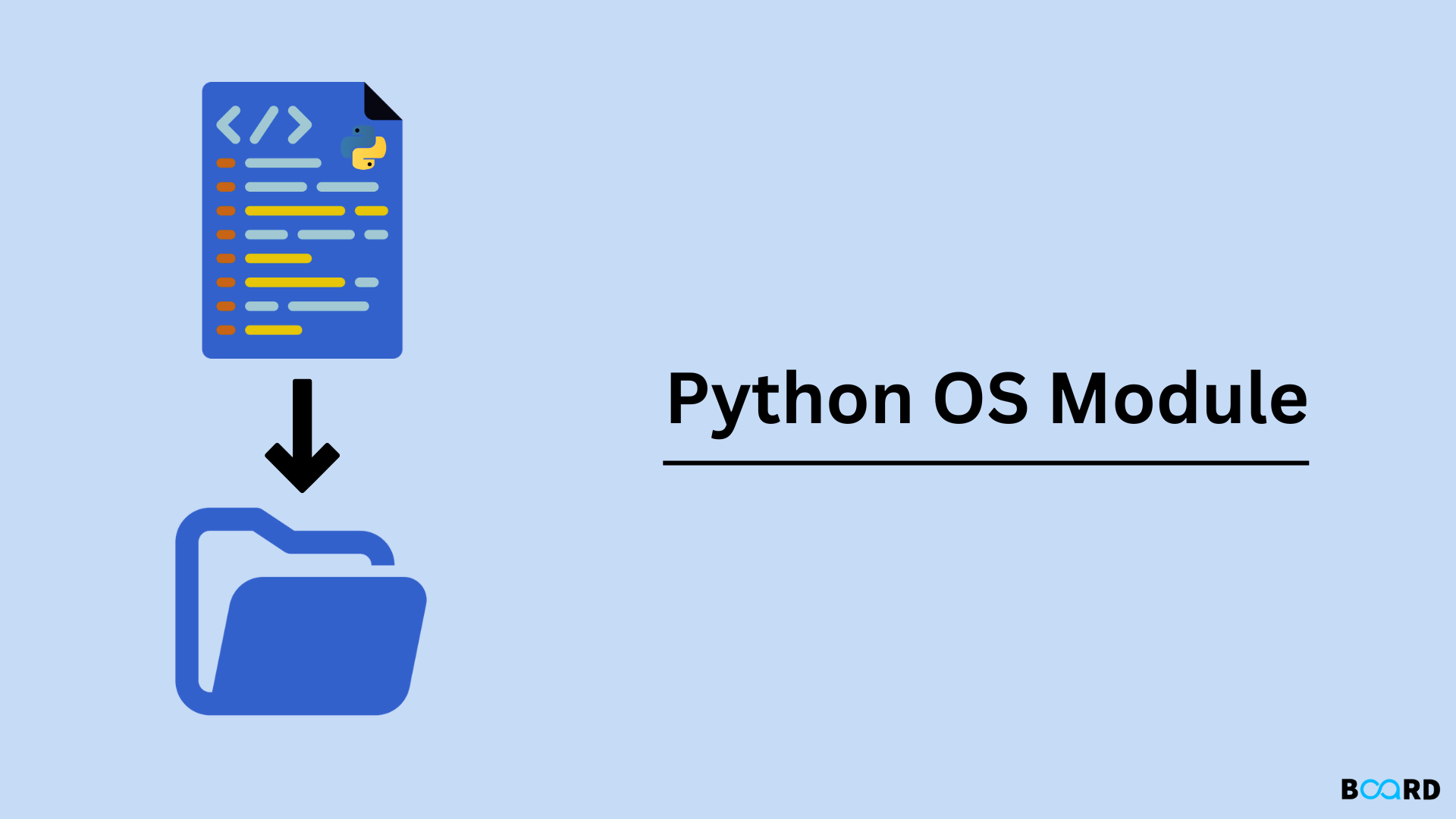
Introduction
Python's OS module offers tools for communicating with the operating system. OS is included in the basic utility modules for Python. A portable method of exploiting operating system-specific functionality is offered by this module. There are numerous functions to deal with the file system in the os and os.path modules.
We can do several operations using the OS module.
Handling the Current Working Directory
Think of the Python operating directory, or Current Working Directory (CWD), as a folder. Python assumes that the file starts in the CWD whenever it is called only by name, so a name-only reference will only work if the file is in Python's CWD.
Getting the Current Working Directory using OS
We can use os.getcwd() method for getting the current working directory:
Example:
Output:
Changing the Current Working Directory using OS
Using the os.chdir() method, the current working directory (CWD) can be changed. This technique modifies the CWD to follow a predetermined path. A new directory path is the only argument that is required.
Example:
Output:
Creating a Directory
We can create a new Directory using OS module, for that, we can use os.mkdir() or os.mkdirs() function
- os.mkdir()
- os.makedirs()
Creating a Directory using os.mkdir()
To create a directory named path with the specified numeric mode, use Python's os.mkdir() method. If the directory that is to be created already exists, this method raises a FileExistsError.
Syntax of os.mkdir():
Parameters of os.mkdir():
os.mkdir() methods uses the below parameters:
- path: An object that represents a file system path. A string or an object made up of bytes that represents a path is a path-like object.
- mode (optional): An Integer value indicating the mode of the newly created directory. If this parameter is omitted, Oo777 is used as the default value.
- dir fd (optional): A file descriptor referring to a directory. This parameter's default value is None.
dir fd is not used if the specified path is absolute.
Return Value of os.mkdir():
os.mkdir() method does not return any value.
Now, lets see how we can use os.mkdir() to create a directory in Python.
Example 1: Use of os.mkdir() method to create directory/file
Output:
Example 2: Errors while using os.mkdir() method
We can get errors while trying to create a directory that has already been created in the system.
Output:
Creating a Directory using os.makedirs()
Python's os.makedirs() method is used to recursively create directories. This means that if any intermediate-level directories are missing when creating a leaf directory, the os.makedirs() method will create them all.
Syntax of os.makedirs():
Parameters of os.makedirs():
os.makedirs() methods uses the below parameters:
- path: An object that resembles a file system path. A string or an object made up of bytes that represents a path is a path-like object.
- mode (optional): An Integer value indicating the directory's mode. If this parameter is omitted, Oo777 is used as the default value.
- Exist ok (Optional): For this parameter, the value False is the default. If its value is False, an OSError is raised if the target directory already exists; otherwise, it is not.
Return Value of os.makedirs():
os.makedirs() method does not return any value.
Now, lets see how we can use os.makedirs() to create a directory in Python.
Example 1: Use of os.makedirs() method to create directory
Output:
Listing out Files and Directories with Python
To get a list of all the files and directories in the given directory, use Python's os.listdir() method. The list of files and directories in the current working directory will be returned if no directory is specified.
Output: