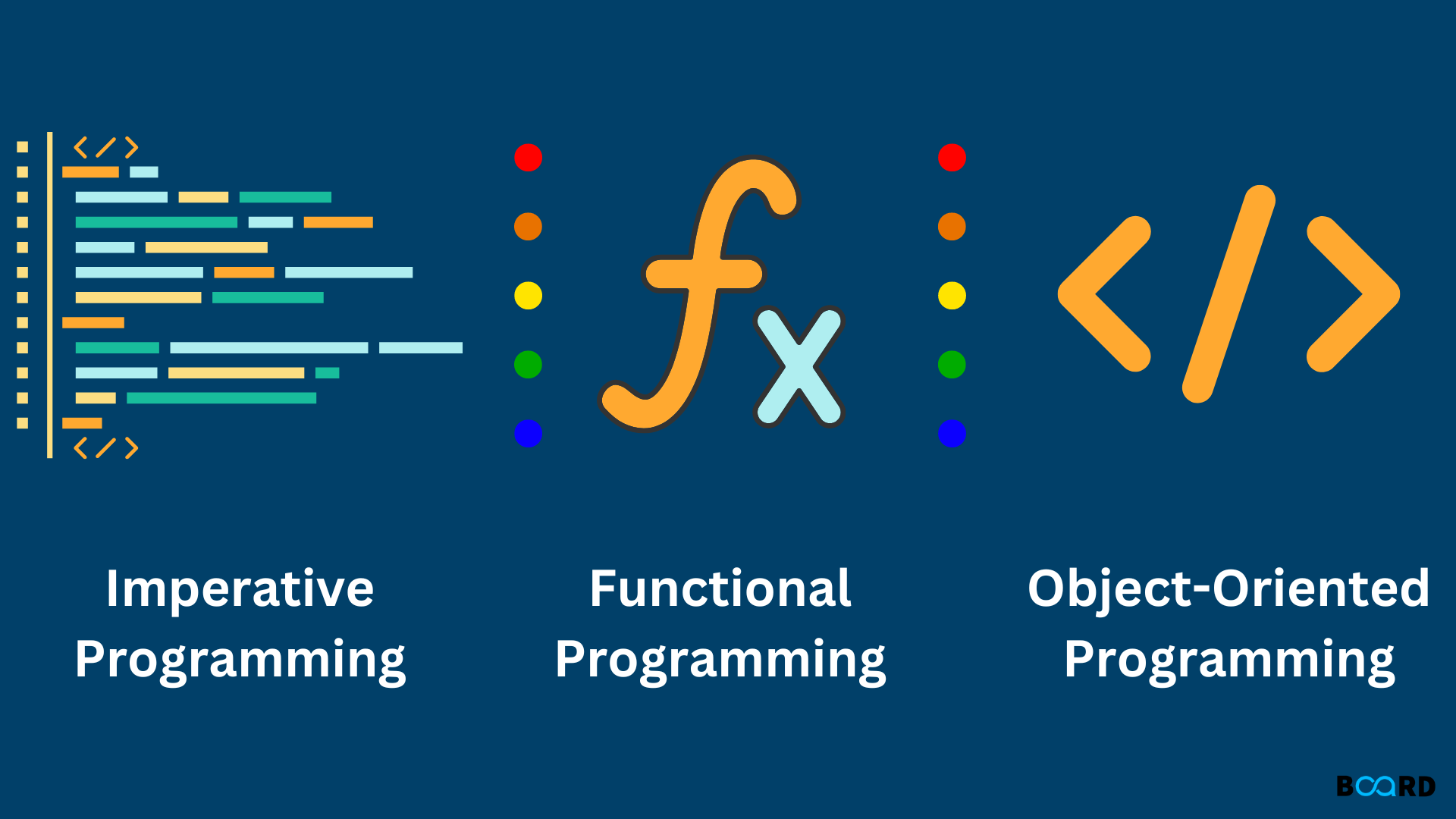
Introduction
A programming paradigm is a method, a strategy, or a technique of writing programmes in a particular programming language to address a problem.
You might be wondering what method or style we're referring to. It is a method for constructing programmes and objects in object-oriented programming, for resolving issues using a sequence of functions in functional programming, or for building logic using a sequence of symbols in logic programming. A programming language has a special feature or uses a particular methodology that makes it the ideal choice to address a particular type of issue if it supports or comes under a particular paradigm.
For instance:
By obfuscating crucial data and associating methods with the entities, object-oriented programming is best suited for developing real-world entities. C++, Java, Python, etc., as examples.
The best method for generating logic from a complicated symbol structure is logical programming. Prolog, F-logic, etc., as examples.
Because different programming problems require various approaches, a good programming language should support a variety of paradigms. A programming language may not be sufficient or lack necessary features if it only supports one or two paradigms.
The majority of contemporary languages allow many paradigms, including C++, C#, Python, Go, etc.
Programming Methodologies
There are several categories in which programming paradigms can be divided, but only two are very important:
- The paradigm of imperative programming
- The paradigm of declarative programming
The paradigm of imperative programming
The imperative programming paradigm, which is the earliest, adheres to the most fundamental principle of programming. These paradigms include modifying the program's present state as the programme is being run step by step. An imperative programme comprises of commands that the computer executes in order to alter the program's state.
The following list includes some of the programming languages that support the imperative paradigm:
C ,C++,Java ,PHP ,Ruby ,Scala
Pascal Code illustration:
The example code below is written in C++, a language that supports imperative programming. Analyze how the program's state changes and how the goal is reached in very few steps.
The Von Neumann architecture statements are followed by the paradigm, which consists of a number of statements and variables that calculate and store the outcome. Object-oriented programming, procedural programming, and other approaches are examples of imperative programming.
Three general subcategories of the imperative are as follows:
- The paradigm of procedural programming
It is identical to imperative programming, but it also includes a procedure call that enables code reuse. And at the time, this functionality represented an incredible development. C++, Java, as examples.
- Programming that is object-oriented (OOP)
This is used to work with objects and classes that represent real-world entities. The class is the object's blueprint, which you can duplicate as many times as you like. These classes include several attributes and operations that are duplicated in objects. C++, Python, etc., as examples.
- Processing in parallel
A programme is processed using this method of programming by being divided into several processors. To speed up the problem-solving process, the system has many processors.
Declarative paradigm for programming
A declarative programming paradigm is one in which the programmer states a result's property without concentrating on how to get there (imperative programming works on how to attain the task by manipulating the state of the program). The method used in this programming is to build some objects or programme pieces. This type of programming places more emphasis on what needs to be done than on how it should be done. It just outlines what the programme must perform; it makes no mention of the work process leading up to the result.
Declarative programming is used in programming languages for logical programming, functional programming, regular expressions, and database queries.
Declarative programming paradigms are supported by a number of languages, including:
Prolog ,javascript ,Scala,Lisp, SQL ,XQuery ,Clojure
Code Example:
The SQL code used in the example below is a language code that supports declarative programming. Look at how it discusses choosing a particular book without emphasizing how to do it.
Declarative programming can be further divided into three main groups:
- The paradigm of logic programming
In logic programming, words are constructed logically, and symbols are used to build expressions. There are numerous models in artificial intelligence and machine learning that employ these programmes.
The way the programmes are run is quite similar to a mathematical statement. It focuses mostly on developing reasoning.
- The notion of functional programming
Programming in which a programme is built by defining and utilising functions is known as functional programming. Functional programming uses functions to map and convert one variable to another value rather than a series of statements.
- A database programming methodology
The foundation of this programming is data inquiry, modification, movement, etc. SQL is the most well-known programming language that can do this.