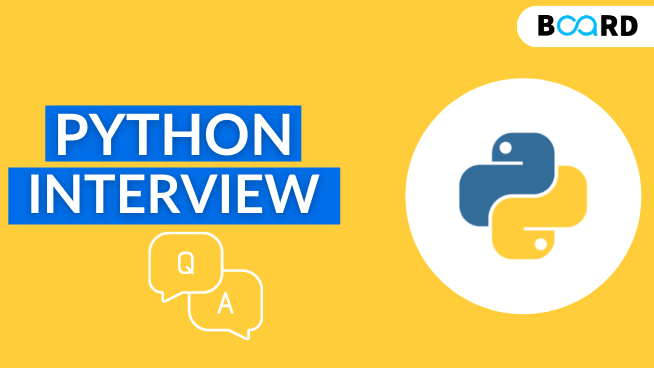
Python is one of the most popular programming languages nowadays, it's a hot choice for both advanced users and beginners because it finds its use in almost every domain, from Web-development to Data Science.
So, if you are attending a python interview, the interviewer will throw some conceptual, classic python interview questions.
Python Interview Question and Answers
To address all the doubts like what kind of python questions are asked in the industry, what should be a good answer to them, how to answer, etc., we have created this list which contains top python interview questions along with their answers.
1. What is an Interpreted Language?
Interpreted languages are those programming languages that execute line by line. For example, python, JavaScript, PHP, etc. Program written in an interpreted language runs directly from the source code, which means an extra compilation step is not required.
2. What is PEP8 and why is it Important?
PEP stands for Python Enhancement Proposal. It is a set of rules that specify how to format python code for maximum readability.
It is important because it contains design guidelines for python code i.e. when someone is writing code at the enterprise level or contributing to open-source, one needs to follow those guidelines strictly.
3. What is the Difference Between Lists and Tuples?
Lists in python are mutable i.e. they can be edited, whereas tuples are immutable i.e. they can’t be edited. Lists are comparatively slower than tuples but it also offers more functionality like inserting, deleting, modifying, etc.
4. What is the __init__ method?
__init__ is a constructor method that is automatically called to allocate memory whenever an object/instance of the class is created. Its task of constructors is to initialize (assign values) to the data members of the class when an object of the class is created.
5. What is Self in Python?
Self is a keyword used to define an object or instance of a class. It helps to distinguish between attributes and methods of the class and its local variables. It is used to access the variable associated with the current instance.
For example -
class Employee:
def __init__(self, name, empID):
self.name=name
self.empID=empID
6. How can you Randomize List items in place?
We can import shuffle from a random module, then we just pass the list name as an argument in the shuffle. It will randomize list items.
7. What are Python Namespaces?
Namespaces in python ensure that object names in the program are unique so that they can be used without any conflict. At the backend python implements namespaces with a dictionary where ‘name as key’ is mapped to ‘object as value’.
8. What is the Lambda Function in Python?
Lambda is an inline and anonymous single expression function that can have any number of parameters. It is used to make new function objects and to return them at the runtime.
Here is the example of the lambda function:
sum = lambda a, b : a + b
print(sum(10, 5))
It will print 15.
9. What is Docstring in Python?
It is also known as document string which is used to document a specific code block. It should describe what is the functionality of the function or method.
10. What is Pickling and Unpickling?
Pickling is a process in which the pickle module converts any python object to string representation and dumps it into a file using the dump function.
Whereas the unpickling process is just the opposite i.e. it retrieves the original python object from a stored string representation.
11. What is the usage of help() and dir() functions in Python?
- help() function is used to display docstring and it also displays the help related to modules, attributes, keywords, etc.
- dir() function returns a list of attributes and methods associated with any object.
12. What is the Difference Between Iterators and Generators?
- Iterators are used to convert objects to an iterator using the iter() function. It is implemented using a class, and no local variables are required to iterate over an object.
- Generators are usually used in the loop to generate an iterator by returning all values in the loop without affecting the iteration of the loop. It uses the ‘yield’ keyword instead of returning it in a defined function.
We can say that every generator is an iterator, whereas every iterator is not a generator.
13. What is the Difference Between Arrays and Lists in Python?
Lists:
- They can contain elements of different data types.
- No need to import any module for declaration.
- Can’t handle arithmetic operations.
- They require larger memory hence preferred for shorter sequences of data only.
Arrays:
- They can contain elements of the same data types only.
- Need to import array modules to use them in code.
- Can directly handle arithmetic operations.
- Comparatively memory efficient than lists hence they are used to store long sequences of data.
14. What is a Dictionary in Python?
Dictionary is a data type as well as a data structure that is used to store key-value pairs. It is analogous to the Map data structure used in Java and C++.
For example:
dictionary={
‘Apple’:1,
‘Banana’:2,
………………..
………………..
‘Xyz fruit’: 100
}
And if we want to value associated with a banana then, we would write print(dictionary[‘banana’])
15. What is the Difference Between Deep and Shallow Copy?
Shallow Copy:
- It copies references of objects to the original memory address.
- It reflects changes made to new or copied objects, in the original object.
- It is comparatively faster than the deep copy.
Deep Copy:
- It stores copies of an object's value.
- It doesn’t reflect changes made to new or copied objects, in the original object.
- It is comparatively slower.
Wrapping Up
In this blog, we have discussed types of python interview questions and answers that are most likely to be asked in a python interview. We sincerely hope that this blog gives you a quick glimpse of the python questions, and now you are confident to crack any Python interview.
If you have a keen interest in Python and want to upskill yourself to stand out of the crowd, check out the Python Programming Certification Course, where you will gain expert knowledge in Python irrespective of whether you took this course as a hobby or as a portfolio builder. In the end, you will earn a Python programming course certification.