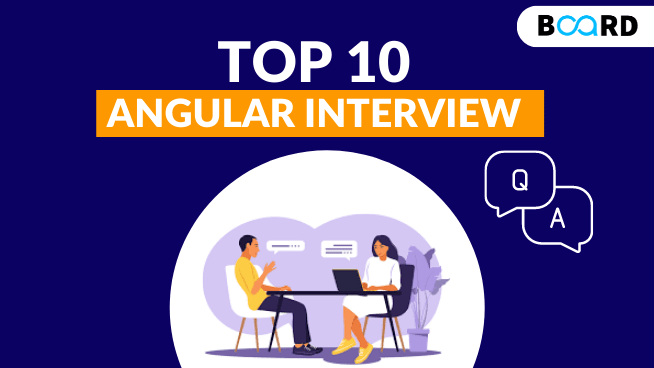
1. What do you understand by Angular JS?
To put it simply, Angular JS is an open source JavaScript framework which is used to create dynamic, single web page applications. It allows us to create rich and cross-platform web applications with fewer lines of code. It runs on plain JavaScript and HTML hence there is no need for any other dependencies to make the framework work.
2. What are the Key Features of Angular JS?
Some key features of Angular JS are:
- MVC Architecture: The Model View Controller architecture is the main feature of Angular JS. Angular JS automatically combines all the three elements together and there is no need for extra code to connect them.
- Data Binding: Angular JS has 2-way data binding feature.
- Directives: Angular JS has a set of inbuilt attributes called directives. You can also create directives of your own using Angular JS.
- Filters: The filter feature of Angular JS allows you to change data into any format like currency, date, time, JSON string, etc.
- Services: Anguler JS services are functions that contain some business logic and are used to carry out specific tasks.
3. What are the Different ways to Communicate between Modules using Angular JS?
The most common ways of communicating between modules using Angular JS functionality are:
- By using services
- By using events
- By assigning models on $rootScope
- Communicating directly between controllers using $parent, $$childHead, $$nextSibling, etc.
- Directly between controllers using forms of inheritance like ControllerAs
4. How are Directives are Instantiated? Explain in Brief.
- The $compile() function is executed first. It returns the preLink and postLink functions. This functions executed for every directive starting from the parent to the child and then grandchild.
- The controller and preLink functions are also executed for every directive. The order of execution is from the parent to the child and then grandchild.
- The order of execution of the postLink function is in reverse order. First it is executed for the grandchild, then for the child and then for the parent directive.
5. What is Data Binding in Angular JS and what is the difference between One-Way and Two-Way Binding?
Data Binding is simply the automatic binding of data between the view and the model components of MVC architecture. Angular JS uses the two way data binding technique.
In One-way data binding the data is bonded from the model to the view without updating the HTML template. The scope of variable in HTML is set to the first value that its model is assigned to. In order to change the values in HTML template, separate custom code is written which updates the view every time a data binding is done from model to view.
In Two-way data binding, data is binded from mode to view and vice-versa. The HTML template is updated automatically and no custom code is required.
6. What are the different Directive scopes in Angular JS?
There are three types of directive scopes in Angular JS.
- Parent Scope: Parent scope is the default scope. Any changes you make in your directive that comes from the parent scope, will also reflect in the parent scope.
- Child Scope: It is a nested scope which inherits properties from the parent scope. If any function or properties on the child scope are not connected to the parent scope directive then a new child scope is created.
- Isolated scope: This scope is only used for private and internal use and so it does not inherit properties from the parent scope. It is a reusable scope and is used when we develop self-contained directives.
7. Which means of Communication between Modules are Easy to Test?
Communication between modules by using services is easier to test. As services are injected, you can either use real service or a mock service in a test.
Events are also easy to test. If you want to test events on $rootScope then it must be injected into the test.
You can test $rootScope against the existence of some arbitrary. However, sharing data through $rootScope is not considered a good practice.
For testing direct communication between controllers, the expected results should probably be mocked. Otherwise, controllers would need to be manually instantiated to have the right context.
8. Explain the Digest Cycle in Angular JS?
The digest cycle is a part of the data binding process in Angular JS. In each digest cycle the old and new versions of the scope model are compared. That is, all the new scope model values are compared against the old values and the process is called dirty checking. If any changes are detected, watches set on the new model are triggered and a new digest cycle starts executing. This cycle repeats until all the models are stable.
If you want to trigger a digest cycle manually you can make use of the $apply() function.
9. What do you understand by SPA in Angular JS?
SPA stands for Single Page Application. Such web applications load a single HTML page and update it dynamically as the user interacts with the application. The pages are loaded from the server by creating a single shell page or master page and loading the web page into the master page.
SPA can be implemented in Angular JS by using Angular routes.
10. What are the Advantages of using Angular JS?
- Angular JS makes use of MVC architecture
- It uses two-way data binding
- It provides a desktop like end user experience
- Developing in Angular JS reduces a lot of time and length of code as developers do not have to register callbacks manually or write DOM manipulation tasks again and again
- Angular JS makes testing code easier as it separates DOM manipulation from app logic.
- It allows mutual client-server communication
- It also supports simulations