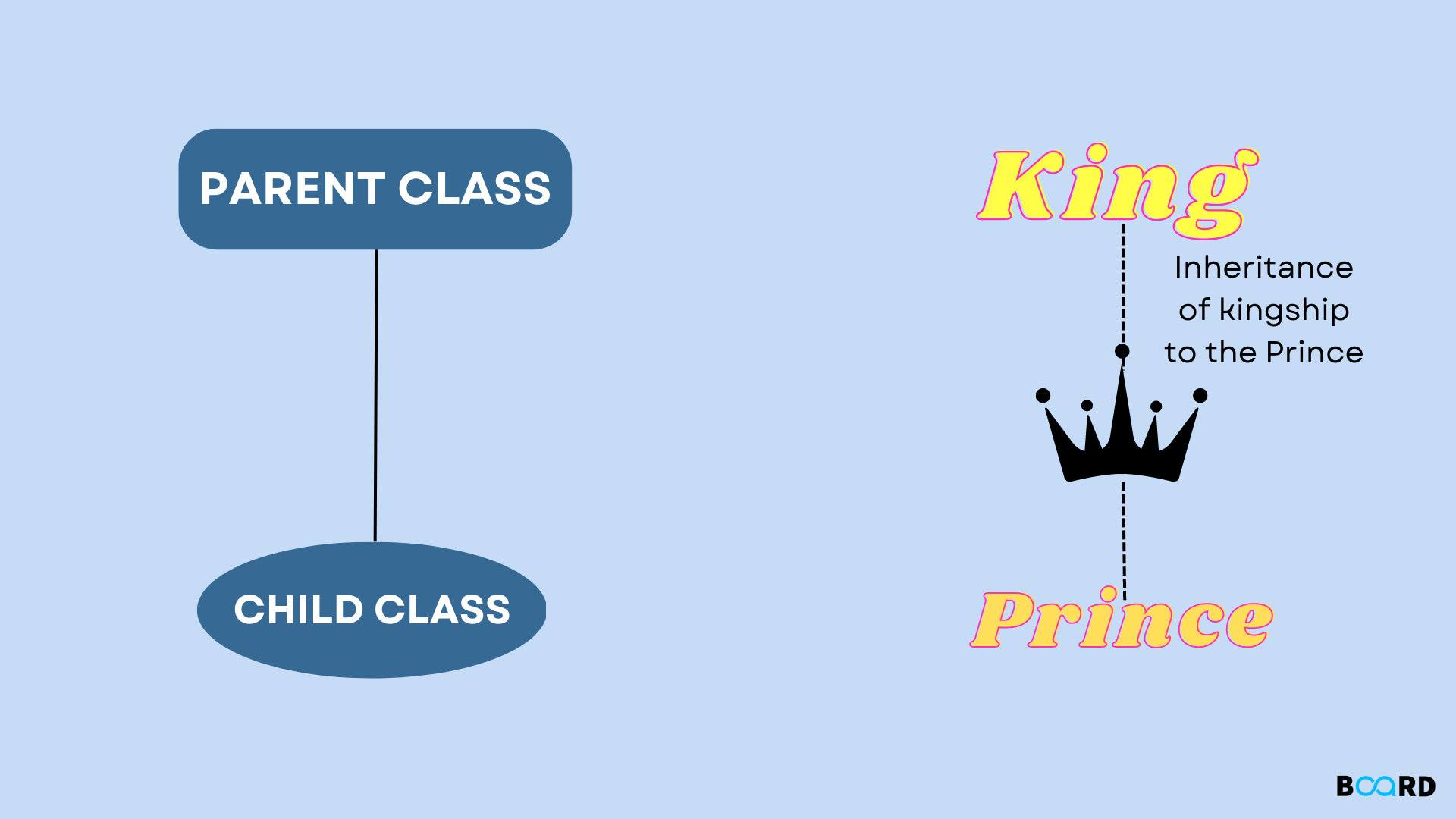
Introduction
Inheritence is a process of obtaining properties and characteristics(variables and methods) of another class. In this hierarchical order, the class which inherits another class is called subclass or child class, and the other class is the parent class.
Inheritance is categorized based on the hierarchy followed and the number of parent classes and subclasses involved.
- It is an excellent representation of relationships in the real world.
- It allows code reuse. It doesn't require us to create the same code repeatedly and again. It also allows us to add options to an existing class without having to modify the existing code.
- It is a transitive nature, meaning that if B is inherited from another class A, all the subclasses belonging to B will inherit directly from class A.
Types of Inheritance
There are five types of inheritances:
- Single Inheritance
- Multiple Inheritance
- Multilevel Inheritance
- Hierarchical Inheritance
- Hybrid Inheritance
Single Inheritance
This type of inheritance enables a subclass or derived class to inherit properties and characteristics of the parent class, this avoids duplication of code and improves code reusability.
Output:
Alright, let’s walk through the above code.
In the above code “Above” is the parent class and “Below” is the child class that inherits the parent class. Implementing inheritance in python is a simple task, we just need to mention the parent class name in the parentheses of the child class. We are creating objects of both parent class and child class, and here comes an interesting point about the inheritance. A child class can access the methods and variables of the parent class, whereas the vice versa is not true.
So in the above code temp1 object can access both fun1 and fun2 methods whereas the temp2 object can access only the fun1 method. Similarly, the same rule applies to variables in the code. And accessing a child class method or variable from a parent class object will throw an error. If the last line in the code is uncommented then it raises an error.
Multiple Inheritance
This inheritance enables a child class to inherit from more than one parent class. This type of inheritance is not supported by java classes, but python does support this kind of inheritance. It has a massive advantage if we have a requirement of gathering multiple characteristics from different classes.
Output:
In the above code, we’ve created two parent classes “A”, “B”. Following the syntax of the inheritance in python, we’ve created a child class, which inherits both classes “A” and “B”. As discussed earlier that a child class can access the methods and variables of the parent class, The child class “C” can access the methods of its parent
Multilevel inheritance
The features that are part of the original class, as well as the class that is derived from it, are passed on to the new class. It is similar to a relationship involving grandparents and children.
Example:
Output:
Hierarchical Inheritance
If multiple derived classes are created from the same base, this kind of Inheritance is known as hierarchical inheritance. In this instance, we have two base classes as a parent (base) class as well as two children (derived) classes.
Example:
Output:
Hybrid inheritance
Hybrid Inheritance is a blend of more than one type of inheritance. The class is derived from the two classes as in the multiple inheritance. However, one of the parent classes is not the base class. It is a derived class. This feature enables the user to utilize the feature of inheritance at its best. This satisfies the requirement of implementing a code that needs multiple inheritances in implementation.