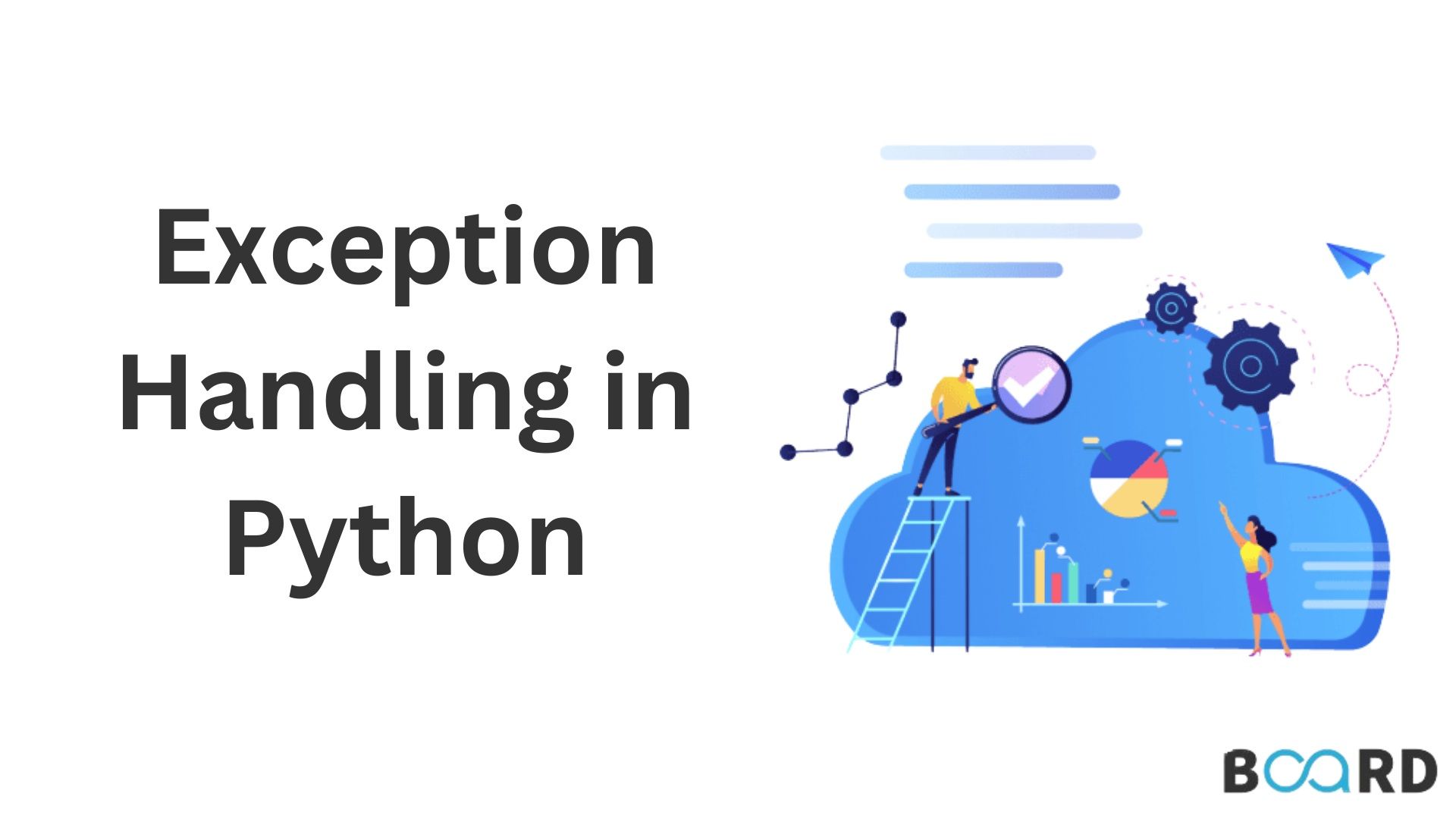
What is an Exception in Python
An event that occurs during the execution of the program and obstructs the regular flow of the program's instructions is an exception. A Python script typically raises an exception when it comes across a situation that it cannot handle. A Python object that represents an error is called an exception.
When a Python script encounters an exception, it must either deal with it right away or quit and stop working.
Code Example of an Exception
Let's take an example code when an exception occurs when we try to divide a number with 0.
Output
Handling an Exception
If your program contains any suspicious code that could cause an exception, you can protect it by enclosing it in a try: block. Include an except: statement after the try: block, then a block of code that solves the issue as elegantly as possible.
Code Example of an Exception Handling:
Let's take an example code where we are handling the above ZerDivisionError Exception.
Output:
Catching and Handling specific Exceptions:
To specify handlers for various exceptions, a try statement may contain more than one except clause. Please be aware that only one handler will be run at a time. The standard syntax for adding particular exceptions is:
Syntax
Example: let's take an example code where we are handling ZeroDivisionError Specifically.
Output
Try with Else Clause
Python also supports the else clause, which must come after all except clauses, in the try-except block. Only when the try clause fails to throw an exception does the code move on to the else block.
Example
Let's take an example code where we are handling the exception with the else clause.
Output
Finally Keyword in Python
The finally keyword is available in Python, and it is always used after the try and except blocks. The final block is always executed after the try block has terminated normally or after the try block has terminated for some other reason.
Example
Let's take an example code where we are handling the exception using Finally Keyword.
Output
Raising Exceptions in Python
We can also raise exceptions in Python for our needs. This can be done using raise keyword.
Example
Let's take an example code where we are raising our custom exception.
Output