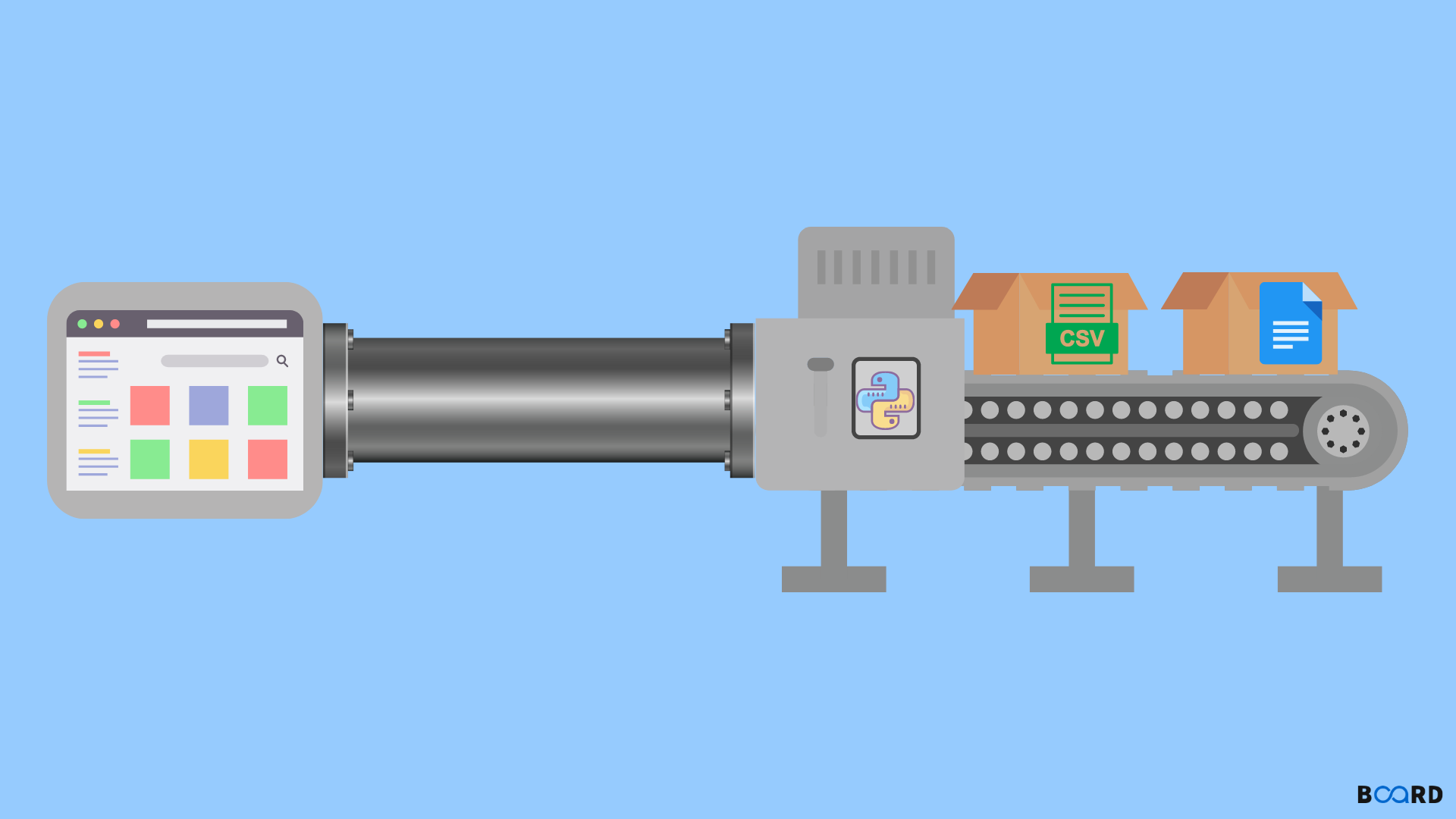
Introduction
Web scratching is the most common way of gathering and parsing crude information from the Internet, and the Python people group has concocted some powerful web-scratching instruments.
The Web has the best wellspring of data on earth. Many disciplines, like information science, business knowledge, and analytical revealing, can benefit enormously from gathering and investigating information from sites.
Scratch and Parse Text From Sites
Gathering information from sites utilizing a computerized cycle is known as "web scratching." A few sites expressly disallow clients from scratching their information with automated devices like the ones that you'll make in this instructional exercise. Sites do this for two potential reasons:
- The site has a valid justification for safeguarding its information. For example, Google Guides only allows you to demand such a small number of results excessively fast.
- Making many rehashed solicitations to a site's server might go through transfer speed, dialing back the site for different clients, and possibly overburdening the server to such an extent that the site quits answering thoroughly.
Before involving your Python abilities for web scratching, you should continuously check your objective site's good use strategy to check whether getting to the site with computerized devices is an infringement of its terms of purpose. Legitimately, web scratching against the desires of a site is a very hazy situation.
Put Together Your Most Important Web Scrubber
The Python standard library includes a helpful pack for web tinkering called urllib, which contains tools for interacting with URLs. particularly the urllib. The limit urlopen(), which you can use to open a URL inside of a program, is consolidated by the sales module.
Enter the following code in the stuff's bright window to import urlopen():
The page that will load has the following URL:
Passing the URL to urlopen() will open the page:
An HTTPResponse object is returned by urlopen():
To remove the HTML from the page, first, use the HTTPResponse thing's.read() method, which returns a series of bytes. Then, employing UTF-8, interpret() to convert the bytes into a string
Right now you can print the HTML to see the things on the site page:
Output:
You used urllib to access the webpage as you would in a browser. However, you copied the source code as text rather than graphing the content. Now that you have the HTML as text, there are various methods to extract information from it.
Text from HTML Can Be Extracted Using String Methods
Using string methods is one method for obtaining data from HTML code on a web page. For instance, you could use. Find () to look for the element in the HTML text and then a string slice to get the title.
You can obtain the index of the opening substring because.find() delivers the index of the first instance of a substring.
By supplying the string "" to. find(), and you don't want the tag's index:
The title can also be obtained by slicing the HTML string:
HTML in the real world can be far more intricate and unpredictable than it is on the Aphrodite profile page. Here is another scrapable profile page with slightly messier HTML:
Utilizing the same technique as in the preceding example, try extracting the title from this new URL:
The title includes a small amount of HTML. How come that?
There is a slight discrepancy between the HTML for the /profiles/Poseidon page and the /profiles/aphrodite page. The initial tag.
These issues can develop in a variety of unanticipated ways. You need a more dependable method of text extraction from HTML.