Node.js Essentials: Understanding Express, NPM, and More
How to Read a File in Node.js?
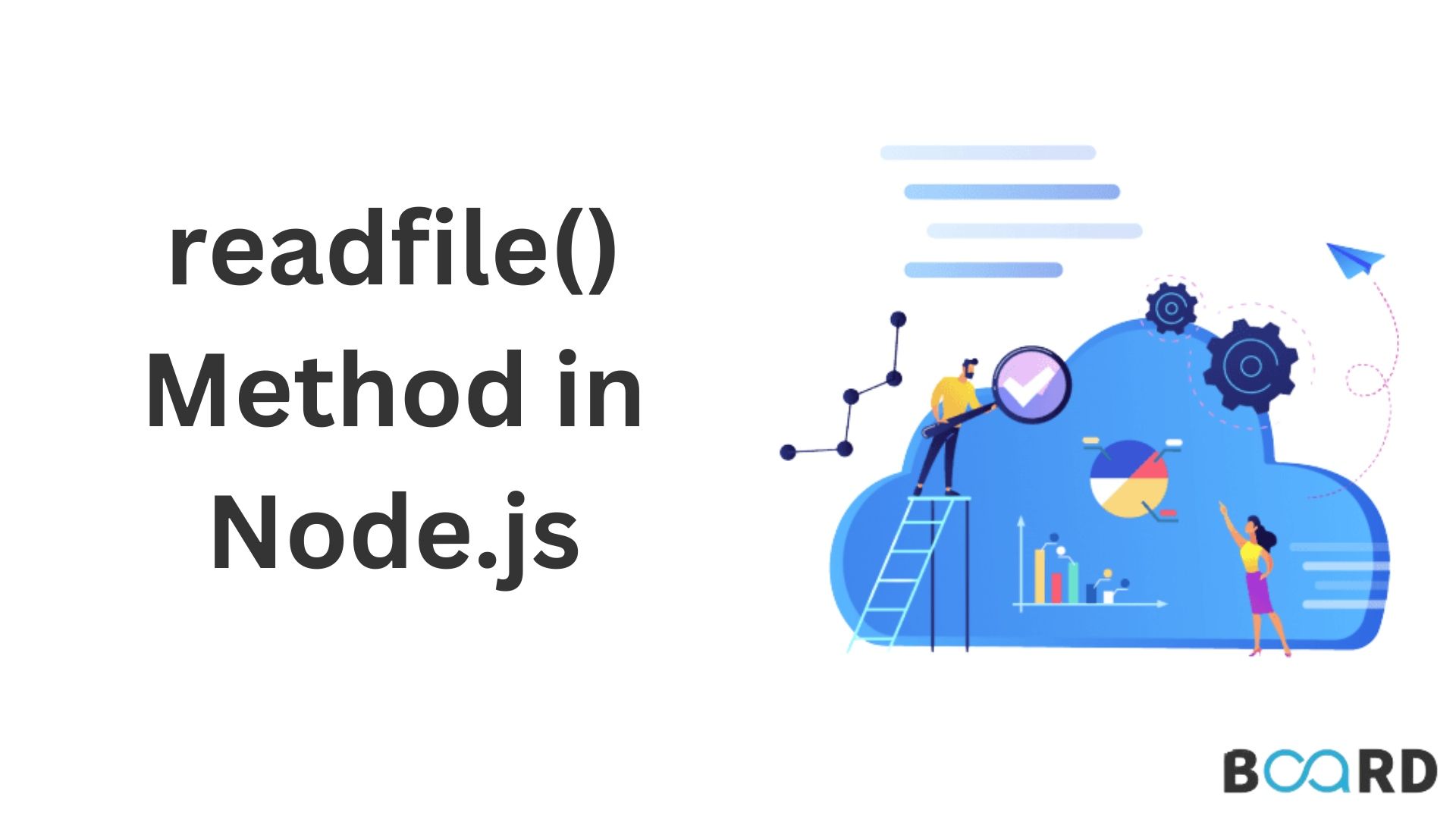
Introduction
In Node.js, the fs (File System) module provides an API for interacting with the file system on your computer. The fs module includes a variety of methods for reading and writing files, including the readFile() method, which allows you to read the contents of a file asynchronously.
To use the readFile() method, you first need to import the fs module at the top of your Node.js script:
Next, you can call the readFile() method and pass it the path to the file you want to read, as well as a callback function that will be executed once the file has been read:
In this example, the readFile() method is called on the fs object and passes the path to the file to be read and a callback function as arguments. The callback function takes two arguments: an error object and the data read from the file. If an error occurs while reading the file, the error object will be non-null and contain details about the error. If the file is read successfully, the data argument will contain the contents of the file.
The readFile() method reads the entire file's contents into memory, so it is unsuitable for reading large files. If you need to read a large file, use the readFileStream() method, which allows you to read the file in chunks and process the data as it is read.
Implementation
Here is an example of how to use the readFileStream() method to read a large file
In this example, the createReadStream() method of the fs module is used to create a readable stream for the file. The data event of the stream is then listened to using the on() method, and a callback function is provided that is called every time a chunk of data is read from the file. The callback function appends the chunk of data to the fileData variable. When the end event is emitted, indicating that the entire file has been read, the fileData variable contains the file's contents.
In addition to reading the contents of a file, the fs module also provides methods for writing to a file, such as the writeFile() method. Here is an example of how to use the writeFile() method to write data to a file:
In this example, the writeFile() method is called on the fs object and passes the path to the file, the data to be written, and a callback function as arguments. The callback function takes an error object as an argument. If an error occurs while writing the file, the error object will be non-null and contain details about the error. If the file is written successfully, the callback function will be called, and a message will be logged to the console.
Conclusion
In summary, the fs module in Node.js provides a variety of methods for interacting with the file system. The readFile() method allows you to read the contents of a file asynchronously, while the readFileStream() method allows you to read a large file in chunks and process the data as it is read. The writeFile() method allows you to write data to a file. Understanding these methods can be useful when working with data in Node.js. In addition to the fs module, third-party libraries like fs-extra and graceful-fs provide additional functionality for working with the file system in Node.js. Overall, the fs module is a powerful tool for reading and writing files in Node.js, and is an essential part of many Node.js applications.