Node.js Essentials: Understanding Express, NPM, and More
writeFile() in Nodejs
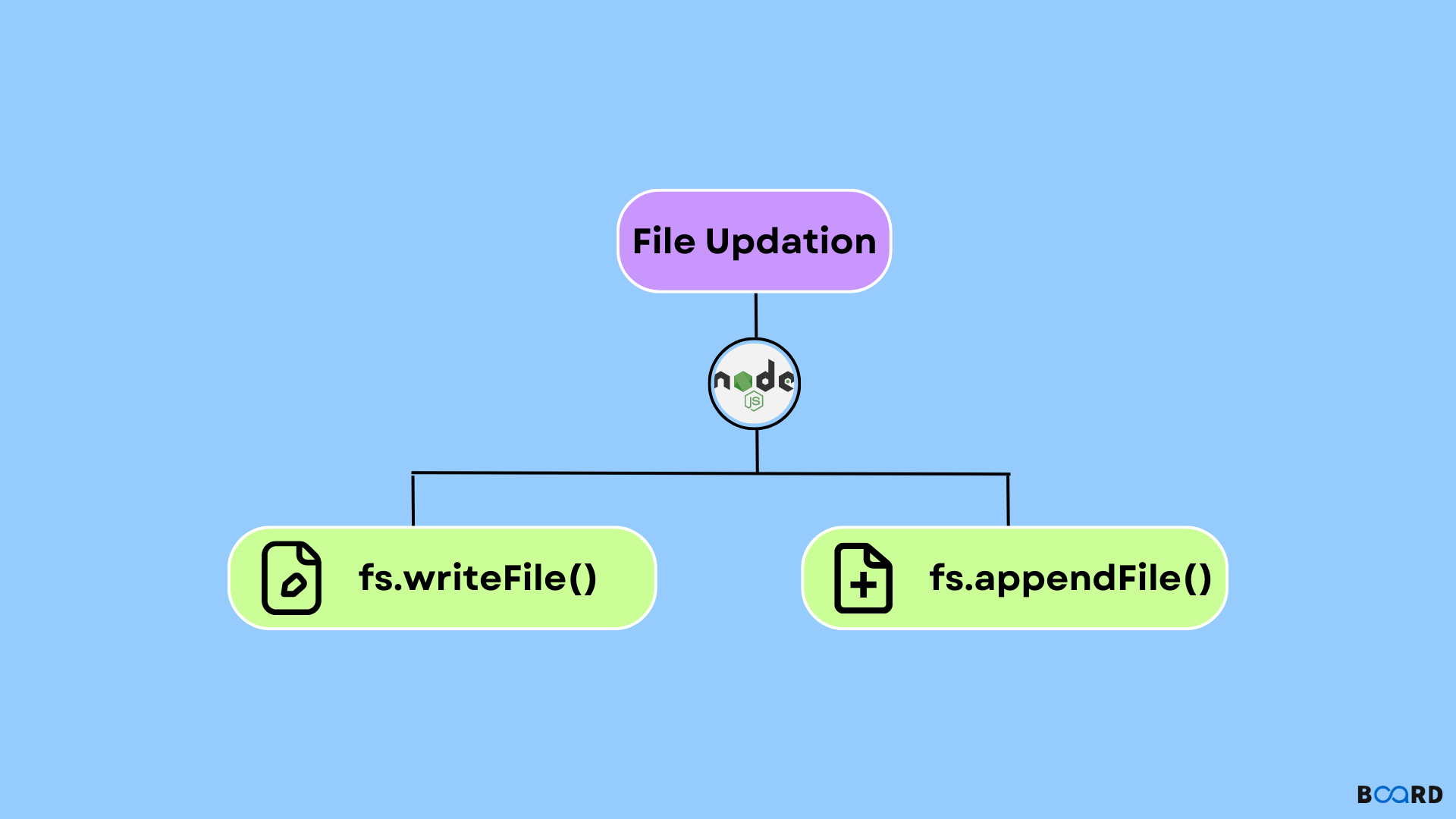
Introduction
In Node.js, the fs (File System) module provides an API for interacting with the file system on your computer. The fs module includes a variety of methods for reading and writing files, including the writeFile() method, which allows you to write data to a file asynchronously.
To use the writeFile() method, you first need to import the fs module at the top of your Node.js script:
const fs = require("fs");
Next, you can call the writeFile() method and pass it the path to the file you want to write, the data you want to write to the file, and a callback function that will be executed once the file has been written:
In this example, the writeFile() method is called on the fs object and passes the path to the file, the data to be written, and a callback function as arguments. The callback function takes an error object as an argument. If an error occurs while writing the file, the error object will be non-null and contain details about the error. If the file is written successfully, the callback function will be called, and a message will be logged to the console.
By default, the writeFile() method will overwrite the file's contents if it already exists. If you want to append data to the end of a file rather than overwriting it, you can use the appendFile() method instead. Here is an example of how to use the appendFile() method to append data to a file:
In this example, the appendFile() method is called on the fs object and passes the path to the file, the data to be written, and a callback function as arguments. The callback function takes an error object as an argument. If an error occurs while writing the file, the error object will be non-null and contain details about the error. If the data is appended to the file successfully, the callback function will be called, and a message will be logged to the console.
The writeFile() and appendFile() methods support writing data in various formats, including strings, buffers, and JSON objects. Here are some examples of how to use the writeFile() and appendFile() methods to write different types of data to a file:
Writing a string to a file
Writing a buffer to a file
Writing a JSON object to a file
In addition to writing data to a file, the fs module also provides methods for reading from a file, such as the readFile() method. Here is an example of how to use the readFile() method to read the contents of a file and then write the data to a different file using the writeFile() method:
In this example, the readFile() method is called on the fs object and passed the path to the input file and a callback function as arguments. The callback function takes an error object and the data read from the file as arguments. If an error occurs while reading the file, the error object will be non-null and contain details about the error.
Conclusion
To summarize, the fs module in Node.js provides a variety of methods for interacting with the file system, including the writeFile() and appendFile() methods for writing data to a file. The writeFile() and appendFile() methods can be used to write various data types to a file, including strings, buffers, and JSON objects. The fs module also includes the readFile() method for reading data