Fundamentals of Operating System
Introduction to Fork Call
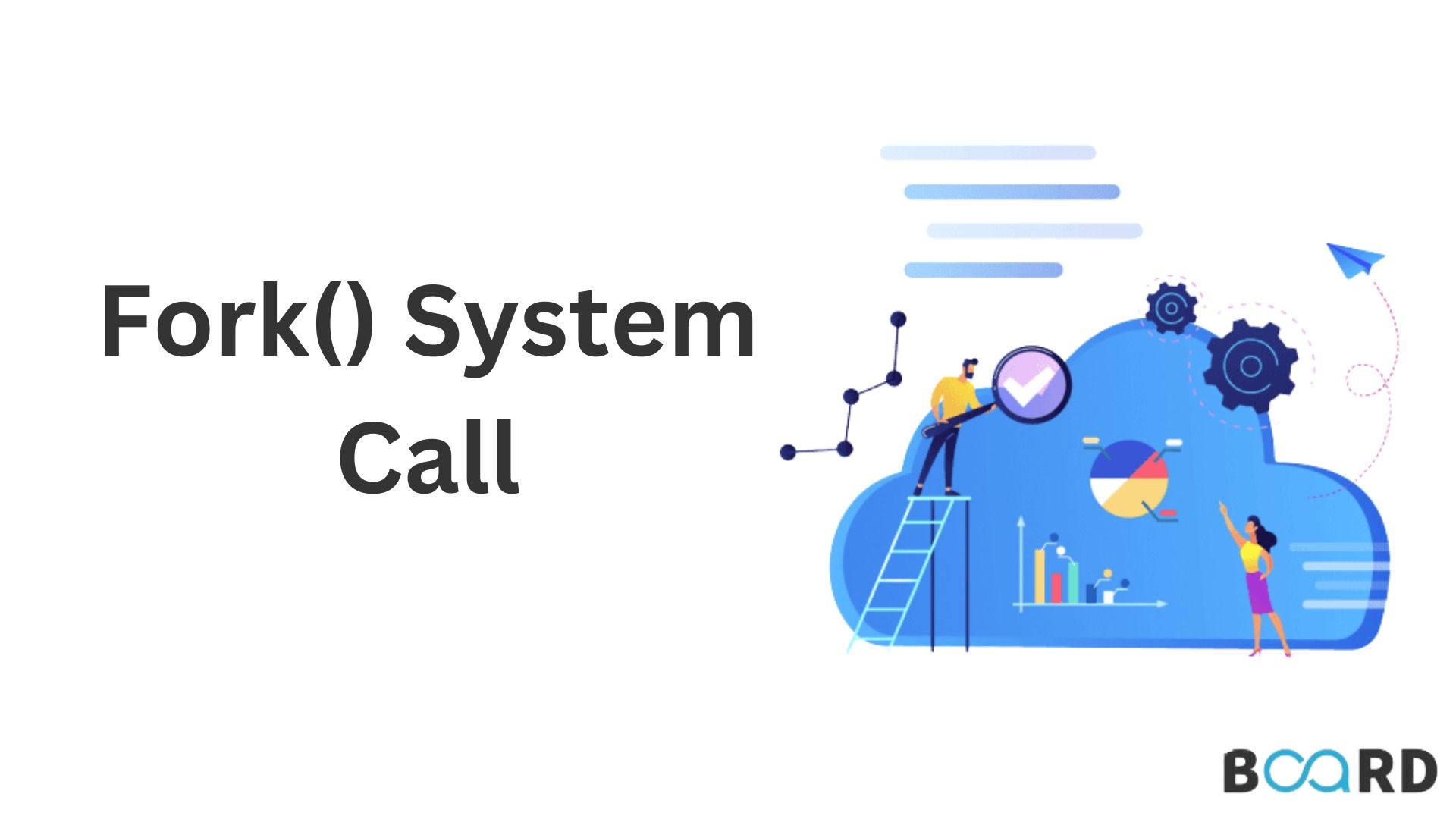
Introduction
The fork system call is specially used to make a new process that is known as a child process that runs simultaneously with the process that invokes the fork() call. Once a new child process is initiated both processes will run the next instruction after the fork() system call. The child process makes use of the same program counter (PC), the same type of CPU registers, and similar open files that are used in the parent process.
The fork system call doesn’t take any parameter and it returns an integer as an output. The following are the values that a fork() function returns:
- Positive value: The value is returned to the parent or caller. The value represents the process ID of the newly created child process.
- Zero: This value is returned to the newly created child process.
- Negative value: This value is returned when the child process is not created successfully.
Let us consider the following programs that will make us understand the fork system call very well
Source Code
Output:
Output Description:
As you can see in the output, “Board Infinity” has been displayed twice.
Now let us try to run the following code this time:
Source Code
Output:
Output Description:
As you can see in the output, “Board Infinity” has been displayed eight times.
From the above examples, we can deduce that the number of time processes are created is equal to 2^N where N is equal to the number of fork() calls made. For example, if there exist 4 fork() calls then the following statements would be executed in 2^4 = 16 processes.
It is important to note that even if a parent and child process both are running the same program then it doesn’t guarantee that they are identical. The operating system is responsible for allocating different data and states for the two processes, and the flow of control for these processes can be very different.
How fork() is different from exec()
The fork() system call makes a new process. The new process that is created using the fork() is just a replica of the current process except for the value which is returned. The exec() system call makes the replacement of the current process with a new program.
Conclusion
In this tutorial, we discussed fork calls. We illustrated how fork() creates new processes using a C++ program. We believe that you must have learned something new from this article.