Mastering HTML and CSS: From Fundamentals to Advanced Techniques
How to do Form Validation
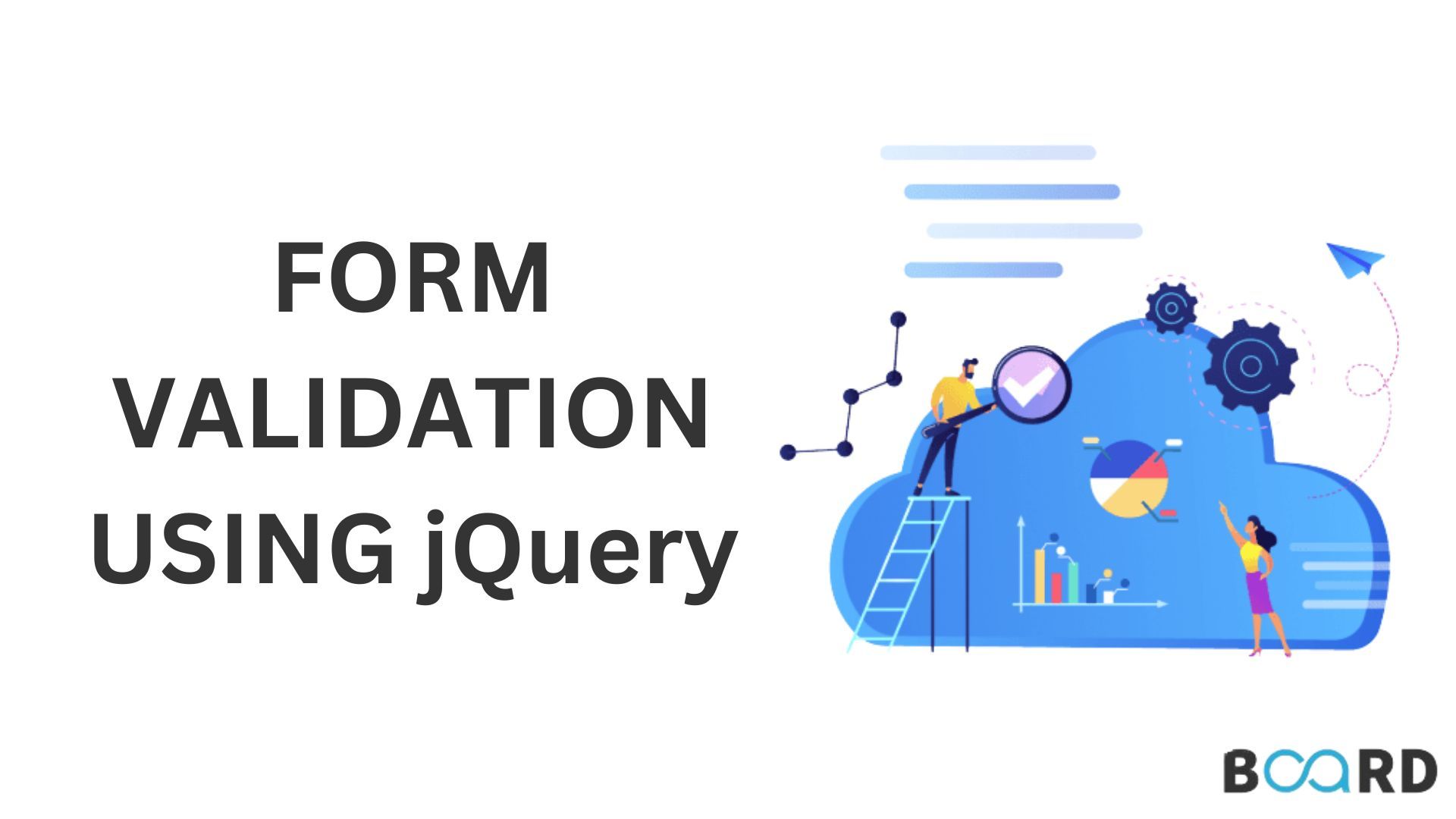
Introduction
In this tutorial, we will take a look at form validation using jQuery. We will start by discussing the different types of validation you can do with it, and then dive into how to validate your own forms. Form validation is one of the most important parts of web development. It helps the user to know if they have provided all the necessary information and also alerts them in case they provide an invalid input, Form validation is a security measure to ensure that the data entered by the user is valid.
jQuery offers a lot of different plugins that enable form validation. These plugins can validate email, text, number, range and many other types of inputs.
The following are some of the most popular jQuery form validation plugins:
1) Validation Plugin 2) jQuery UI 3) Chosen
4) jQuery Validation Plugin
What is jQuery?
jQuery is a JavaScript library that provides us with many useful tools for manipulating HTML elements on the page. These tools allow us to make changes to the DOM (Document Object Model) of the web page without having to worry about cross-browser compatibility issues. In addition, jQuery makes it easier to write less code and do more complex tasks.
The purpose of form validation is to ensure that users enter data in a way that makes sense. There are many different types of validations that can be applied to forms. These include:
- Required Fields - Ensure that a field is filled out before submitting the form.
- Length - Make sure that a user enters a number, letter, or special character.
- Email - Check if a user entered a valid email address.
- Number Range - Make sure that a value falls between two numbers.
- Date Range - Make sure that the date format is correct.
- Regular Expression - Make sure that a string matches a regular expression.
- Custom Rules - Create custom rules for each input field.
First, we will create a simple HTML form with some text fields and submit button. Then, we will add an event handler for the submit button which validates all of the fields in the form before submitting it to the server. Form validation is a way of making sure that a form is filled out correctly before the user submits it. This protects the user from submitting invalid data which can result in errors and delays. Form validation can be done using JavaScript libraries such as jQuery. Some of the most popular validation types are required, email, number, and URL.
The following example shows how to use jQuery to validate a form:
In this section, we will learn how to validate form fields using jQuery.
Form validation for the clientside using jQuery plugin :
Code:
Output
CONCLUSION
Validation is important for the user’s experience with the form. It helps to improve the user interface and saves them from filling in unnecessary fields. The validation process is also essential for web developers as it protects against errors in form submission and data loss. Form validation is a process of checking if the inputted data on a webpage is sufficient to fulfill its intended purpose. Form validation can be done as part of this process or separately, but is generally done at both stages to increase overall compatibility and validity of form submission.