Mastering HTML and CSS: From Fundamentals to Advanced Techniques
Understanding PUT and PATCH
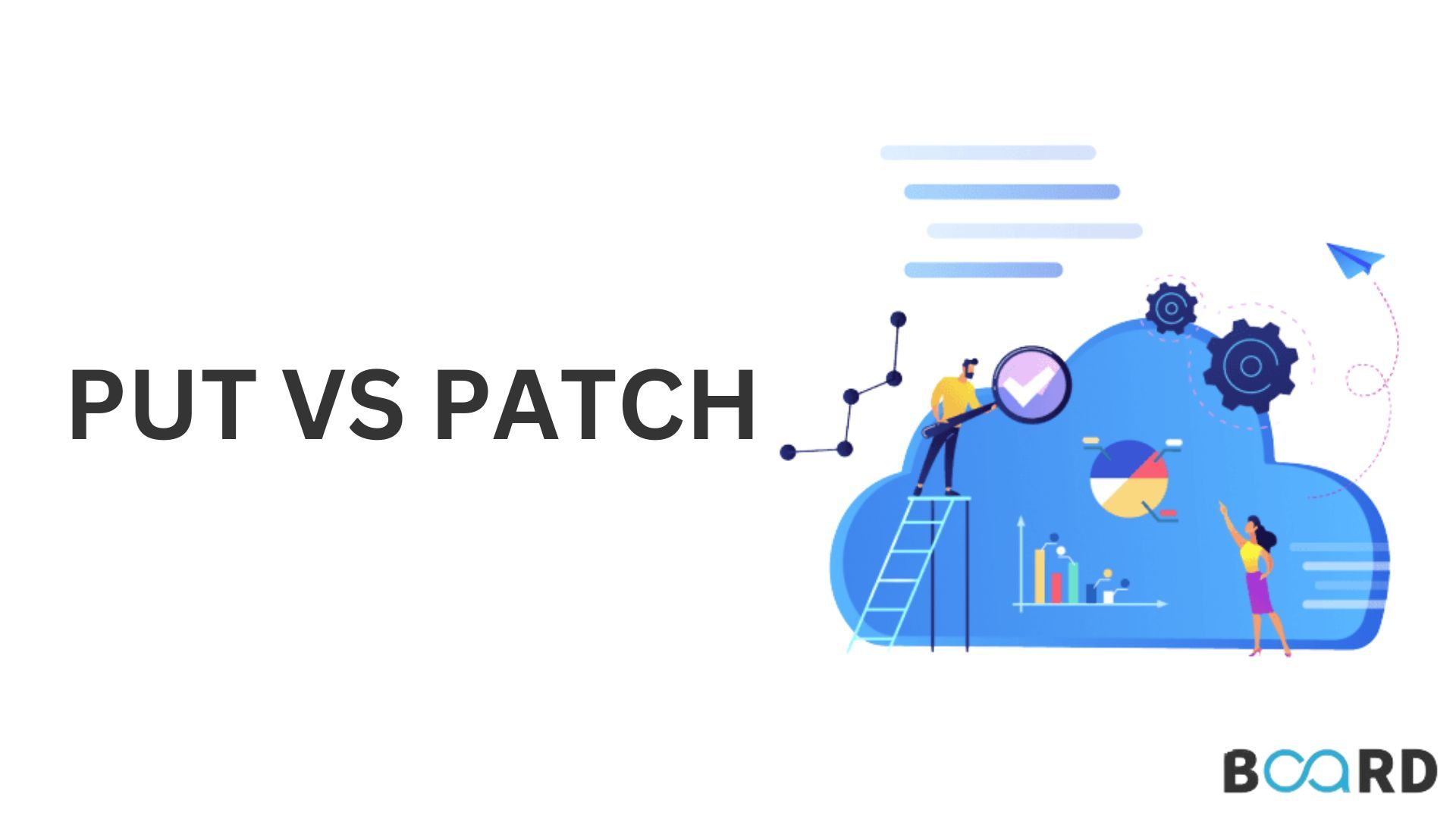
PUT HTTP Request
The HTTP PUT method is used to modify the data in a resource. The resource being modified is specified by the URL and can be either a file or an element of an XML document. The request body contains the updated data to be uploaded. The response body returns the status code of the operation and includes an entity containing the updated content.
PUT is a method of modifying resources where the client sends data that updates the entire resource.
Example
When you want to update the Student's name and email, you have to send all the parameters of the Student including those not to be updated in the request body, otherwise, it will simply replace the entire resource with the name and email.
CODE
OUTPUT
PATCH HTTP Request
The HTTP PATCH method allows you to change the state of a file or page. This means you can use it to update the content of a web page, which is useful if you need to fix an error or add new features. You can use this method to make small changes, such as updating a title tag on one page.
To perform an HTTP PATCH request, you send a request with an entity body that contains all the data you want to change in the target resource. The server will then apply any changes and return the updated resource, which can lead to duplicate content issues if multiple resources have changed at once. For example, if you have multiple articles on your website for different topics and you want all of them to link back directly to each other through their titles within those articles, then you could use an HTTP PATCH request to update each article's title tag so that they all link back through the same title tags (e.g., "The Best Way To Build A House"). But if there are any links that go from one article to another, then those links will simply be broken once everything has been updated (e.g., "The Best Way To Build A House").
PATCH does partial updates e.g. Fields that need to be updated by the client, only that field is updated without modifying the other field.
EXAMPLE
CODE
Output
CONCLUSION
PUT and PATCH requests are very similar to each other, but they're also different. Let's take a look at the difference between these two request types.
PUT is used to update a resource on a server. It has no body, and it uses the HTTP method of PUT. If you want to create or update an object in a database, you'll use PUT. If you want to add or remove one or more rows from a table in a database, you'll use PUT.
PATCH is used to update one or more pieces of data in an existing resource on the server. It has no body, and it uses the HTTP method of PUT. The resource being updated can be anything—for example, an entire webpage, part of an existing webpage, or even just one piece of text in your web page.
The distinction between these two requests is that PATCH doesn't change any structure (it only updates part of it), whereas PUT does (it changes the whole thing).