Excelling Interviews for Specific Roles and Fields
Java Interview Questions and Answers (2023)
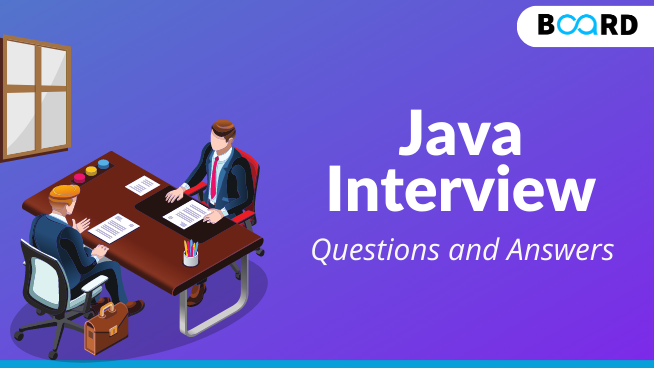
Introduction
When it comes to recalling a perennial language that has made sizeable contributions to software development, Java is the name that comes to everyone's mind. It is one of the most widely-known programming languages used in different verticals like networking, desktop application, web development, system programming, etc. As such, being well-versed in Java will give you an upper hand in tech interviews and help you understand pure object-oriented programming concepts. This article compiles some of the most frequently asked Java interview questions so that you can brush up on your preparation and excel in the interview round.
Java Interview Questions for Freshers
Here are some basic Java interview questions that every fresher and Java developer should know.
1. How will you define the Java programming language?
It is by far the most commonly asked Java interview question for freshers. Java is a class-based, high-level, general-purpose, platform-independent, pure object-oriented programming language. Java resides among the top 7 programming languages. A Java code can run on any platform that supports Java without recompilation. It was created by James Gosling at Sun Microsystems, Inc.
2. Mention any four differences between C++ and Java.
Four differences between C++ and Java are:
3. Mention some key features of Java.
Some key features of Java are:
- Pure object-oriented: Java follows an object-oriented programming paradigm. It allows developers to maintain their code as a combination of different object types. Java also allows developers to incorporate data and behavior. Programmers cannot create a program without a class, hence the term ‘pure’.
- Portable: Java caters to a read-once-write-anywhere strategy. Java allows developers to execute their Java code on any machine. Developers can convert the Java program (.java) into the bytecode (.class) and run it on any system.
- Secure: Java comes under the category of secure programming language because it does not leverage the concept of explicit pointers. Java also renders Bytecode and Exception handling. That makes Java codes more secure.
- Dynamic: Java is a dynamic programming language, meaning it provides the dynamic loading of classes as per demand.
4. What is JVM?
It is another popular Java Interview question. Java Virtual Machine, abbreviated as JVM, is an abstract virtual machine that provides a runtime environment where developers can execute Java bytecode. JVMs are available for many hardware and software platforms. It performs basic operations like:
- Loading the code
- Verifying the code
- Executing the code
- Providing runtime environment for the code
5. What is the expanded form of JRE?
The expanded form of JRE is Java Runtime Environment.
6. Does the Java constructor return any value?
Yes, the Java constructor implicitly returns the current class instance with which it is associated.
Core Java Interview Questions
Here is a set of core Java interview questions frequently asked by many companies that hire Java developers.
1. What is a Class Loader?
A Java class loader is a subsystem of Java Virtual Machine (JVM). It is designed to load class files when a program executes. Class Loader is the first one responsible for loading the executable file.
2. What are the different types of memory allocations or areas available in Java?
Java allows five different forms of memory allocation. These are:
- Stack Memory
- Class Memory
- Program Counter-Memory
- Heap Memory
- Native Method Stack Memory
3. What is a Just-in-time (JIT) Compiler?
The Just-in-time (JIT) compiler is a component of the runtime environment. It enhances the performance of the code execution. It acts as a part of the bytecode having similar functionality, decreasing the compilation time. It improves the code performance at run time by communicating with the Java Virtual Machine (JVM). It then compiles those bytecode sequences into local machine code.
4. How will you explain the statement public static void main(String args[]) to a newbie Java programmer?
The main() function is the entry point of a Java program. Java programmers need to write it as:
public static void main(String args[]).
However, each has a separate meaning and responsibility associated with the main().
- Public: It is an access modifier. Specifying it within the main() allows any class to access this method freely.
- Static: It is a keyword that identifies the main() from within the class. It also determines that programmers can access main() without creating the instance of a class. So, you will notice that a main() method without static will throw an error at the time of compilation. It is because the main() is called by the JVM before creating any other object or invoking any other function.
- Void: This return type defines that the method will not return any value.
- Main: It is the method name that the JVM searches first with a particular signature only. From this method, the main execution takes place.
- String args[]: This means, all the parameters passed over to the main method will be accepted as a string.
5. Give a one-line answer for “what is a singleton class?”
This is an important core Java interview question. In Java, a singleton class is a unique class type that can render only one object (or a class instance) at a time.
6. Can we inherit the constructor in Java?
No.
Java Interview Questions for Experienced Professionals
Here is a set of Java interview questions for experienced professionals.
1. What is the Wrapper class in Java?
Wrapper classes in Java are unique types of classes whose object wraps or comprises primitive data types. Every primitive data type has an associated wrapper class dedicated to it. These are called wrapper classes because these classes have the characteristics to ‘wrap’ the primitive data type into an object of that class. Example: Character, Integer, Float, Long, etc.
2. Mention three different ways of creating an infinite loop in Java.
Infinite loops are exceptional loops that keep executing indefinitely without any break or stop. Programmers need to stop these loops forcefully.
Three different ways of creating infinite loops are:
for (;;) {
// Logic or loop body without any break logic
}
while(true) {
// Logic or loop body without any break logic
}
do{
// Logic or loop body without any break logic
}while(true);
3. What are constructors in Java? What are its types?
Constructors are a set of codes that help initialize a Java object. It uses the same name as the class name with which it is associated. Constructors get invoked when programmers create the object of the class automatically. Java caters to two different types of constructors. These are:
- Default constructor
- Parameterized constructors
4. Define object cloning.
Object cloning helps Java programmers create the exact copy of any Java object. To create this, programmers have to use the clone() method of the Object class. Programmers have to implement java. lang.Cloneable interface by the Java class whose object clone they want to create.
5. Can we perform method overloading on the main() method?
Yes, we can create any number of main() methods within a single Java program with the help of method overloading.
6. Why doesn't Java leverage the concept of pointers?
Java doesn't leverage the concept of pointers because they are unsafe (anyone can access memory through them) and increase program complexity. Since Java wants to render things simply for the programmers, adding the pointers will make it difficult. Java does not require manual control over allocation and deallocation of memory. Instead, it provides automatic memory management through Garbage Collection (GC).
7. What are Java threads?
A thread in Java is a lightweight subprocess followed by a Java program in executing it. It is the smallest processing unit and helps Java developers achieve multiprocessing and multithreading.
8. Is it possible to override a static method?
No, it is not possible to override a static method.
Wrapping up
We hope this compilation of core and basic Java interview questions for freshers and experienced professionals has given you a clear idea of the types of questions you’ll be asked during a Java interview. These Java interview questions also show how robust Java programming is and its sheer scope. It was one of the first, most popular languages that allowed multithreading. Its syntax and features are similar to C and C++, making it easy to understand. Therefore, there are many avenues to widen your career horizons in Java programming. If you want to know more about Java and accelerate your career as a Java developer or software engineer, check out Board Infinity.