Full Stack Development Fundamentals: Skills, Tips, and Resources
Best Resources for Back End Development
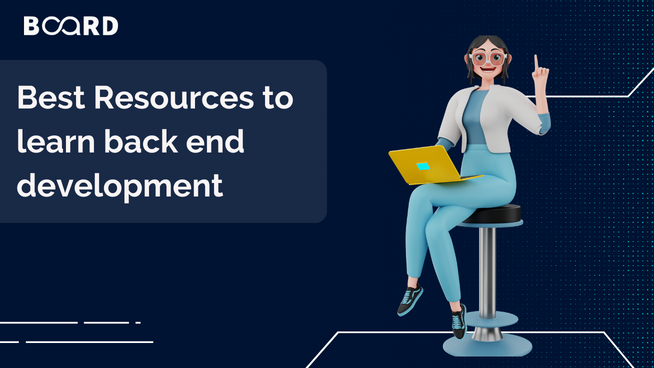
Introduction
Back-end development is the area of website development that deals with server-side code. The development of the user-invisible functions is the responsibility of the back end. While the front end of a website is crucial for the user experience, the back end is just as crucial for the efficient operation of that website. Data, business logic, and the effective retrieval of data from a variety of sources are the main concerns of backend developers. That's not the end of the list. A truly good backend dev is expected to be skilled at a variety of things.
1. Back End Development Books
The books listed below will help you conceptually in the long run:
Programming: Principles and Practice Using C++
Bjarne Stroustrup's book is a great resource for those looking to develop their C++ foundation. Both generic and object-oriented programming are covered in the book. There are numerous exercises available to help clarify the concepts.
Beginning Node.js
This book by Basarat Ali Syed explains the fundamental ideas of NodeJS. Additionally, you will learn how to maintain your data, use the Express framework, and publish web applications online.
The Joy of PHP Programming
This Alan Forbes book is intended for those who want to work as web developers but lack programming knowledge. Everything is covered from scratch in the book, including the fundamentals of HTML, PHP installation, PHP syntax, MySQL, and control structures.
PHP & MySQL: The Missing Manual
This textbook aids programmers in content management, database creation, and user interaction. Understanding database management systems will also help you comprehend how PHP and MySQL are related.
2. Backend Toolkit
Frontend development is primarily influenced by JavaScript, HTML, CSS, and numerous front-end paradigms using these languages, whereas backend development has a much wider range of possibilities. Before moving on to the list of resources, let's define a few key terms.
Languages
The tools that programmers use to create software, apps, and websites. They are employed to generate a set of directives and generate different types of output.
- Examples: Ruby, Java, Python, JavaScript
Libraries
Collections of pre-written code or modules are useful for programmers to use as building blocks for their feature-rich apps. They provide abstraction and are reusable.
- Examples: jQuery, React.js
Frameworks
A group of solutions, instruments, and parts you can access from a single location. They specify the organization of your code and the structure of your project.
- Examples: Ruby on Rails, Angular.js, Django
3. Mastering the Backend
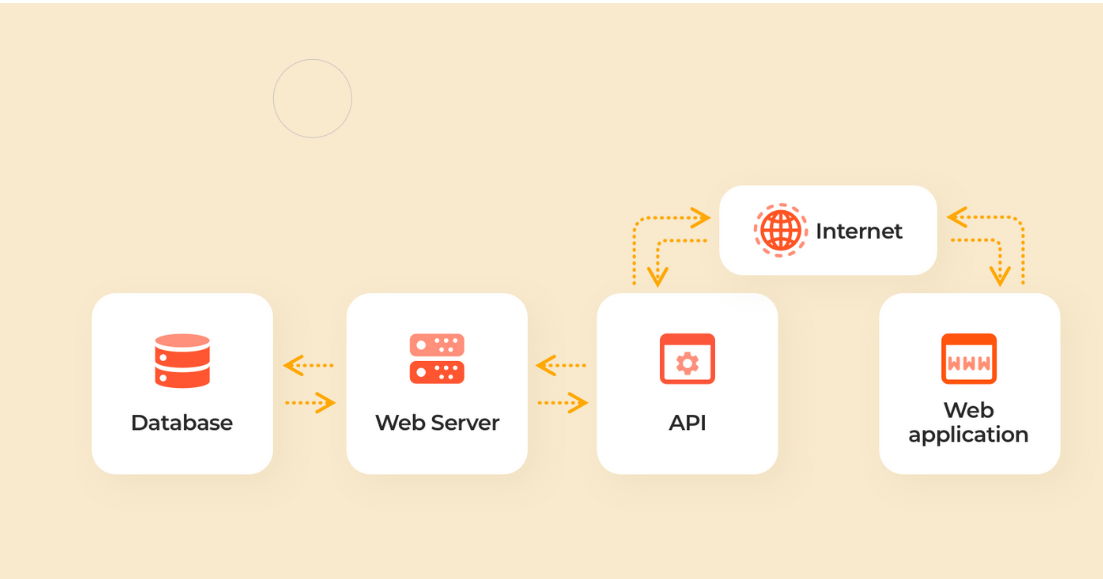
The server-side architecture is broken into four parts:
- Server
- Database
- APIs
- Middleware
You can do a deep dive into backend technologies by reading our How to Become a Backend developer article.
More details about all these backend elements are provided below along with ample resources.
1. Understanding Servers
The server is the engine of the network out of the four parts of your back-end stack, whether it's on-premises or in the cloud. These powerful computers provide the shared resources—such as storing files, confidentiality and cryptography, database systems, mailing, and web applications networks required to function.
Server and Security Resources
The server is the engine of the network out of the four parts of your back-end stack, whether it's on-premises or in the cloud. These powerful computers provide the shared resources—such as storing files, confidentiality and cryptography, database systems, mailing, and web applications networks required to function.
1. Awesome Nodejs - Wonderful Node.js resources and packages from Awesome Nodejs.
2. nodebestpractices - a comprehensive list for creating node apps. essential for large projects.
3. https://owasp.org/www-community/attacks/ - list of malicious attacks and tips on how to evade them
4. https://www.cloudflare.com/learning/ssl/how-does-ssl-work/ - To help you understand Secure Socket Layer and web certificates
5. https://developer.mozilla.org/en-US/docs/Web/HTTP/Cookies - Guide on maintaining best practices when it comes to implementing cookies in your website
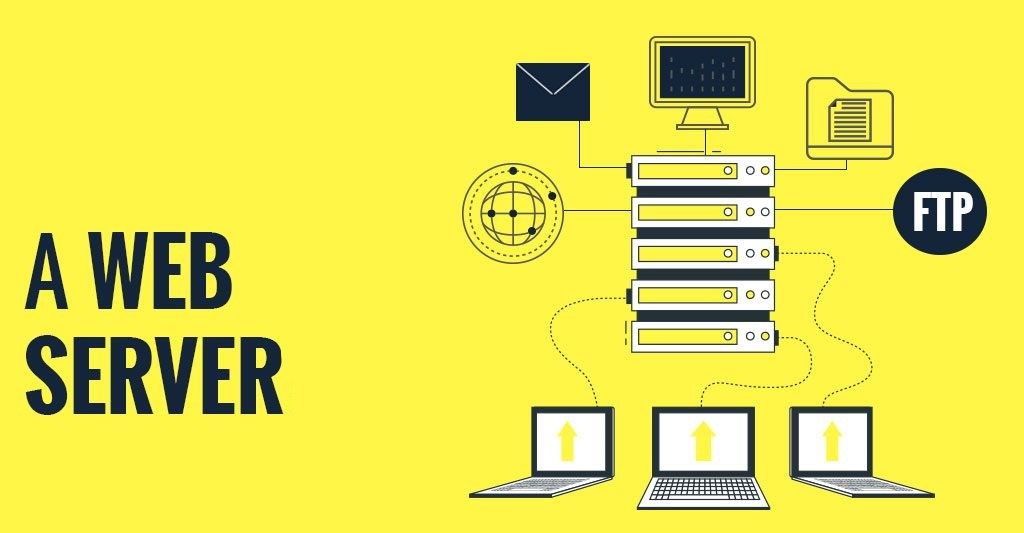
2. Simplifying Databases
In the case of a website, databases serve as the brains that give websites their dynamic nature. When users interact with databases via a website or application, new and edited data can be added. Whether a user is updating their customer information, posting articles to a CMS, or posting images to a Facebook profile, they can make changes to a database from a browser.
Database Tools and Resources:
This is a good time for you to brush up on your DBMS knowledge as well. Check out our 50 DBMS interview questions article.
3. Leveraging APIs
These days, it's impossible to discuss the back-end portion of an application without mentioning APIs (application programming interfaces) as well as the seamless connections they make between apps, software products, database systems, and services. Most network infrastructure architectures are built using APIs, which frequently take the place of more complex programming to enable software communication and data transfer.
Amazing API Resources:
- OpenWeather - You can access current weather information, hourly, weekly and fortnightly forecasts using OpenWeather's straightforward, quick, and free weather API.
- Public APIs - Over 1K Public REST APIs are listed collectively for developers.
- JSONPlaceholder - Free to use fictitious REST API available online for test results and mock-ups.
- Rest Cookbook - Simplified ready-to-implement examples. Learn by doing strategy.
- Quotes REST API
4. Decoupling Middleware
In essence, middleware refers to any server-side software that links an application's front end and back end. It is invisible but necessary, and it must be dependable and consistently perform the tasks that are expected of it.
Additionally, middleware offers services such as system integration and error checking while enabling communication between on-premises and cloud applications. Additionally, effective middleware can power customer engagement, process management, document management, authentication, and many other IT-related functions. An example of an HTTP JavaScript library is Koa.js.
Middle-ware and Cloud Platform Resources
You can get started with Express.js (a great middleware for MERN stack) by checking our blog here.
5. Learning Resource for GIT
Git, a distributed revision control and source code management system with a focus on speed, is the version control system used by GitHub. Here are a few links to reference sheets on bash, SVN migrations, Git commands, and features.
4. Most Productive Tools Used by Backend Developers
Firebase
Firebase offers a comprehensive toolkit for developing, enhancing, and growing your project. Additionally, this mobile platform enables developers to use the toolset to complete a sizeable piece of the app development process that they would otherwise need to construct entirely on their own. As a result, using the Firebase tool saves a lot of time.
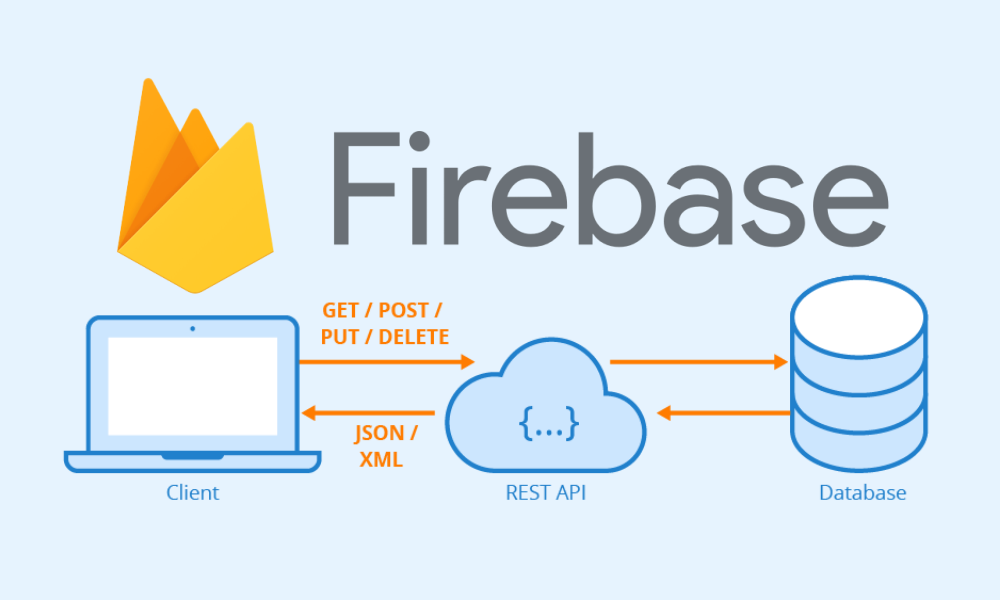
Docker
The important word, agility, is associated with Docker. Docker is used by developers who want their clients to receive features and updates for their apps more quickly. Given that these open-source containers are a significant advancement for cloud development, they are an indispensable tool for developers when laying the foundation for any modern software. Back-end developers deploy Docker and create code with automated infrastructure and configuration, greatly accelerating the deployment process.
Back4App
Back4App is a provider of backend-as-a-service that efficiently propels programs to function. It is a remarkable open-source solution that assists developers in developing complex and scalable mobile and online apps at a quick speed. This solution enables you to smoothly alter and improve your apps in various ways. A free tier is provided by Back4ap.
Heroku
Node.js, Java, Python, Scala, Ruby, PHP, Go, and Clojure are just a few of the eight recognized programming languages supported by Heroku, a cloud platform-based service. Additionally, Heroku offers a fully controlled runtime environment that enables your programs to run within dynos, also known as smart containers.
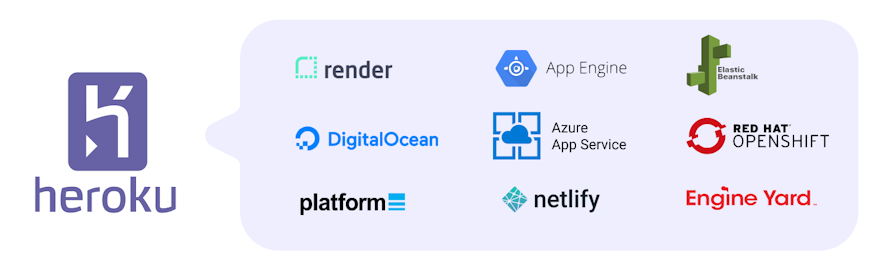
Datadog
Datadog lets you see everything in one place, including your servers, clouds, metrics, apps, and teams. It makes it possible for turnkey integrations to incorporate events and analytics throughout the development stack with ease. Datadog is a cloud-based monitoring-as-a-service program that elucidates modern application concepts for developers, allowing them to assess, debug, and improve application performance
PostmanAPI
Another effective tool that software developers can think about using to speed up and simplify the web development process is Postman API. It enables developers to create, test, and modify APIs rapidly and with much ease.
Jira
A web-based tool called Jira was initially created for tracking issues. It began offering project management services later, in 2012. This tool mostly monitors issues with mobile and web applications. Jira is a project management tool that agile back-end developers use to tailor the workflow for creating apps and deliver them with better assurance. It enables users to arrange and prioritize software development jobs in an enjoyable, rewarding, and flexible manner.
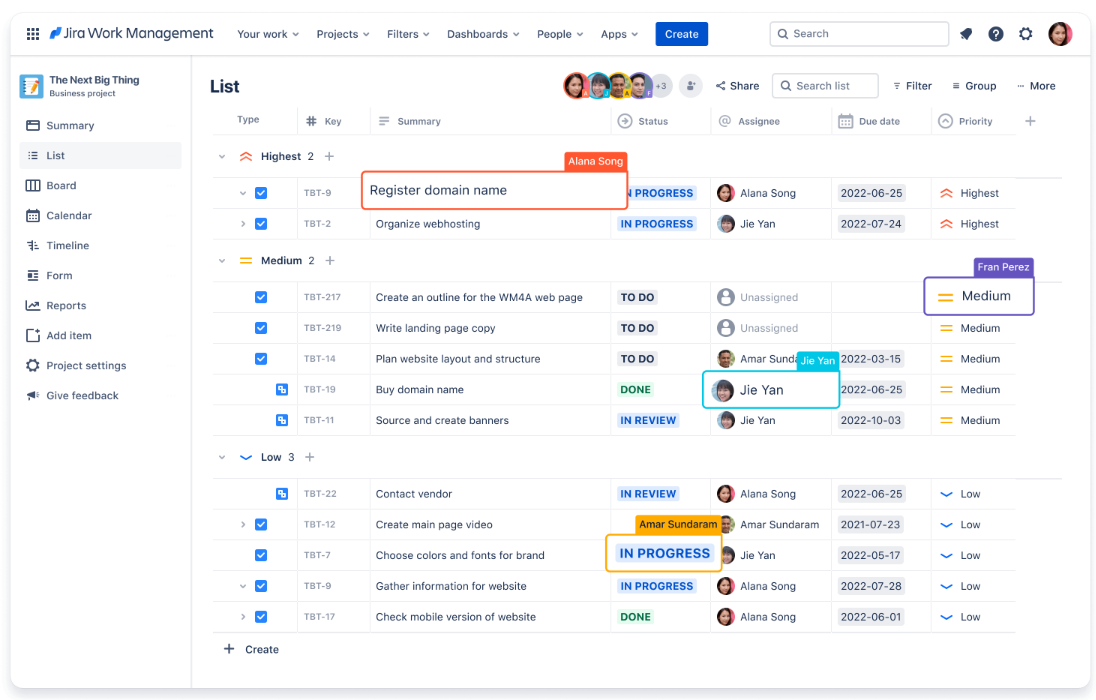
We recommend watching this insightful video about Skills Required for Backend Development as well!
Key Takeaways
From the resources listed above, you are sure to:
- Learn at least one server-side programming language in-depth, like Python, Java, or PHP.
- Learn at least one framework, such as Drupal, Node.js, or Django.
- Get acquainted with web servers along with database management systems.
- Being able to evenly distribute scalable applications.
- Recognize accessibility and security requirements experience working with large teams in a real-world setting using modern version control systems like GIT
Conclusion
Backend development jobs are profitable and exciting, but getting started is challenging. The tasks that a developer could carry out include creating and enhancing application software, planning technical architecture, putting software testing into practice; and troubleshooting code. By making a few wise choices, you may start a prosperous career by developing the necessary abilities to succeed in it.
Given that Java is a crucial part of many different kinds of applications, a wide range of businesses are in need of Java developers.
This Java Backend course provides you with a head start in Java Backend by teaching you Java programming, packages, multi-threading, XML and JDBC, Apache Maven, and more. Gain access to the best content and individualized coaching from professionals in the field. After finishing, you receive a certification in addition to a placement guarantee. Why bother waiting? Join Now!