Essentials of Back-End Development: From APIs to Databases
Client Side and Server Side Scripting: Explanation
Fundamentals of Express.js
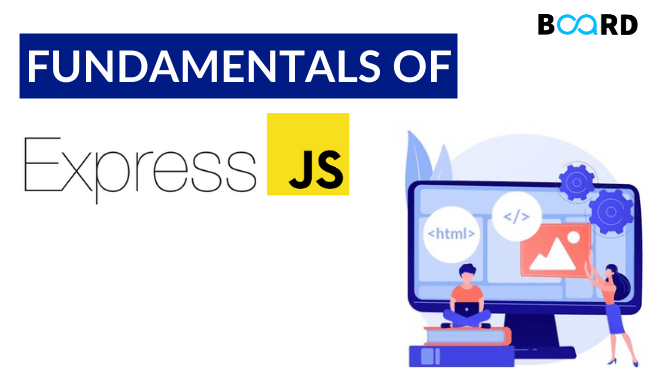
What is Express.js?
The idea behind Express.js is to provide robust tooling for HTTP servers and thus make it a great solution for single page web-applications, websites, or public HTTP APIs.
- Express is a Node.js framework that is minimal, flexible and provides a robust set of features for both mobile and web applications.
- This framework is built on the top of Node.js and it provides a minimal interface with most of the tools required to build any web application.
- It also adds flexibility to any application by providing a plethora of modules available on npm which we can directly embed into Express as needed.
- The primary use of this framework is to provide server-side logic for mobile
Express is responsible for handling the backend part in MEAN, MERN as well as MEVN stack applications. They are open-source JavaScript software stack that is heavily used for building dynamic websites and web applications in the market.
MEAN stands for MongoDB, Express.js, Angular.js and Node.js. MERN stands for MongoDB, Express.js, React.js and Node.js. MEVN stands for MongoDB, Express.js, Vue.js and Node.js.
Features of Express.js
1. Express increases the pace of full-stack application development.
2. Express.js can also be used to create a single page, multi-page and hybrid applications (both web and mobile).
3. It has the Model-View-Controller (MVC) architecture.
4. It can work with Pug, Mustache, EJS and other various templating engines.
5. It also facilitates working with databases such as MongoDB, Redis, and MySQL by making the integration process effortless.
6. It also defines the error-handling middleware.
7. By providing a thin layer of fundamental web application features, Express does not obscure the existent Node.js features.
8. It eases the configuration and customization steps for the application.
9. Since, Express provides a myriad of HTTP utility methods and middleware, creating a robust API is quicker and easier.
Installing Express
Before installing Express.js in the system, we have to ensure that Node.js is already installed. If not, then in such a case, we have to first install Node.js and then proceed with the Express installation process. Once we installed Node.js, the next step is to install Express.
To install Express.js, the first step is to create a project directory and create a package.json file which will hold the project dependencies. The following command can be used:
npm init
Now, we can install Express in the system. It can be installed globally by using the following command:
npm install - g express
In case, if we want to install it locally into the project directory, we can use the below command:
npm install express –save
Some Fundamental Concepts
REST APIs
REST stands for Representational State Transfer. It is referred to as an architectural style and approach for communications purposes that is commonly used in most web services development. It is basically an application program interface (API) that uses the various HTTP requests to GET, PUT, POST and DELETE the data in various applications.
Express.js is developed on the middleware module of Node.js known as connect, and it automatically becomes the ideal choice for a server. This is because of the fact that Express simplifies the process of creating APIs to a large extent. It also helps in exposing APIs for the server to further communicate as a client with the server application. In this case, the connect module communicates with Node.js with the help of HTTP module. Therefore, if we need to work with any of the connect based middleware modules, then we can easily integrate with Express.js.
Scaffolding
When we work with various REST APIs, we have to work with multiple files such as HTML, CSS, JPEGs, etc., for accomplishing any specific task. But managing so many files becomes very difficult for developers. In order to counter such issues, Express provides the developers with an easy solution known as Scaffolding. It is the process of creating and maintaining a proper structure for various full-stack web applications and thus helps in managing the files in proper directories saving us from manually arranging the files and directories.
Thus, it can be said that Scaffolding really helps in creating the skeleton for our express applications and helps us directly jump into the application development. It provides multiple Scaffolding tools like Yeoman and Express-Generator.
Using express-generator
We have to install express-generator before using it in our system.
npm install -g express-generator
After installing express-generator, we can use it to create our project skeleton.
express APP_NAME
Routing and HTTP Requests
Routing is considered the process of determining a specific behaviour of an application. It defines how an application should respond to a client request to a particular route, path, or URI along with a particular HTTP Request method. Any particular route may contain more than one handler functions, which gets executed when the user browses for a specific route.
app.METHOD(PATH, HANDLER)
There are different HTTP methods that can be supplied within any request. These methods are used to specify the user operations. They are:
- GET Method: It helps in requesting the representation of a specific resource by the client.
- POST Method: It helps in requesting the server to accept the enclosed data within the request as a new object.
- PUT Method: It helps in requesting the server to accept the enclosed data within the request as a new object as an altercation to the existing object.
- DELETE Method: It helps in requesting the server to delete a particular resource from the destination.
Example :
var express = require('express');
const app = express();
app.use(express.json());
app.get('/topic', function (req, res) {
console.log("GET");
res.send('<h1>Welcome to GET request!</h1>');
})
app.post('/addTopic', function (req, res) {
console.log("POST");
res.send('<h1>Topic Added by POST Request!</h1>');
})
app.delete('/delete', function (req, res) {
console.log("DELETE");
res.send('<h1>Topic Deleted by DELETE Request!</h1>');
})
Error Handling
The mechanism for catching and processing the errors which might occur during the program execution and disrupt the normal flow is known as Error Handling in Express.js. With the help of middleware, it takes care of errors. It is divided into various categories based on the number of arguments the middleware function takes in. The “error handling middleware” function takes in some specific arguments like, err, req, res and next.
Summary
- In this article, we learnt about Express.js framework built over Node.js and its various features.
- We also understood why Express is very popular among various full-stack web applications and why it is mostly used in MERN and MEAN stack applications.
- We also saw several fundamental concepts in Express like Routing and HTTP Requests, REST APIs, Scaffolding and Error Handling.
If you're someone who is interested in learning about full-stack web development, check out Board Infinity's Full Stack Development Course with certification. Gain expertise with the help of 1:1 mentoring from top industry experts and launch your dream career!