Mastering Software Engineering: Diagrams, Models, and Testing Techniques
Booth’s Algorithm
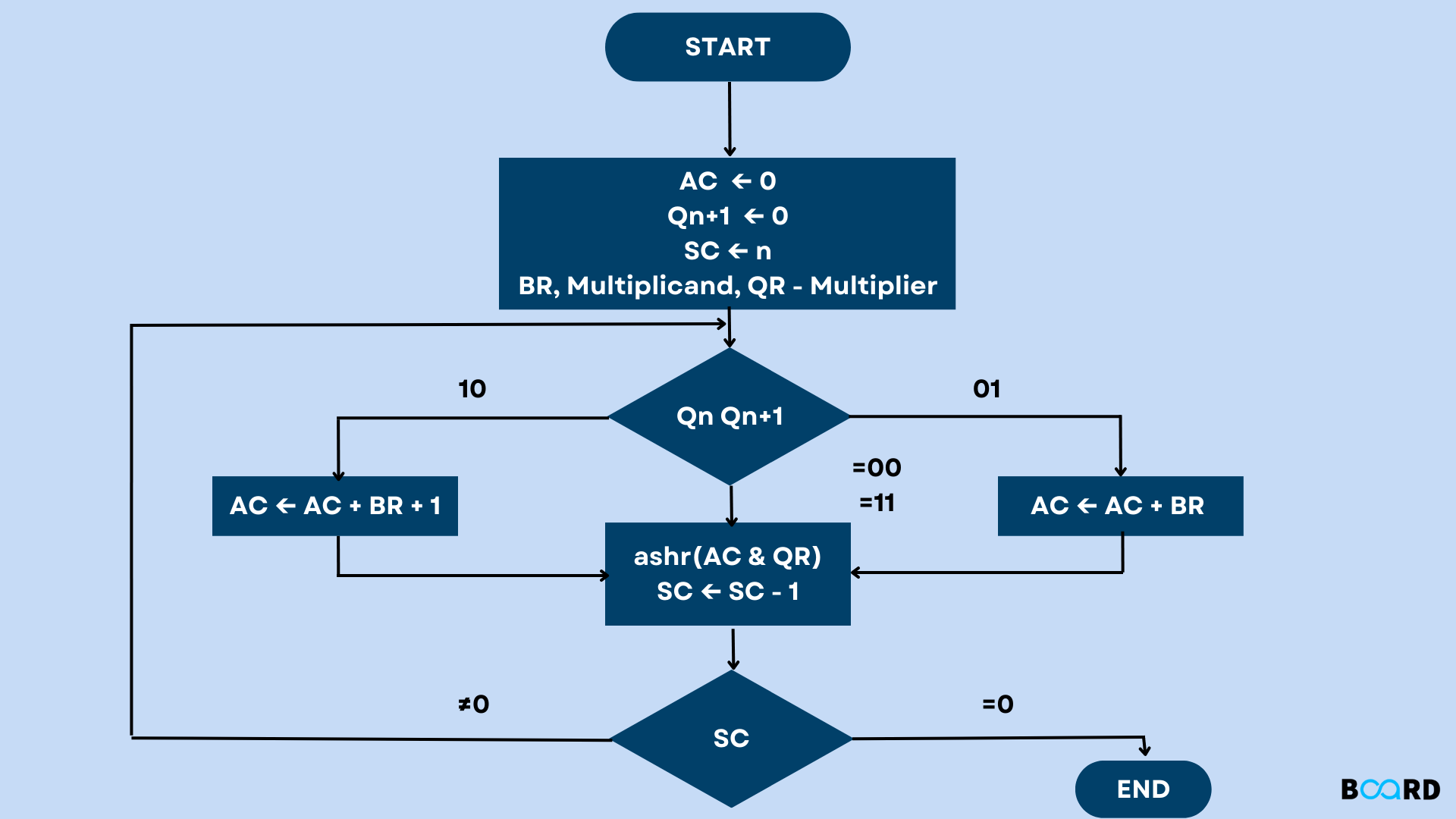
Booth’s algorithm is a method for multiplying two signed binary numbers. It uses a technique called “bit shifting” to quickly multiply the numbers while taking into account their signs. The algorithm works by repeatedly shifting the multiplicand and adding or subtracting it from the partial product based on the value of the multiplier’s bits. This process continues until all bits of the multiplier have been processed, resulting in the final product. Booth’s algorithm is faster than traditional multiplication methods and is often used in computer hardware and software.
Hardware Implementation of Booths Algorithm
Booth’s algorithm is a widely used method for performing binary multiplication, and it has been implemented in hardware in a number of different ways. Some of the key considerations for hardware implementation include efficiency, speed, and accuracy.
One common approach to implementing Booth’s algorithm in hardware is to use a dedicated multiplier unit, which consists of a series of registers and logic gates that are specifically designed for this purpose. The multiplier unit receives the two operands as input, and it performs the necessary shifts and additions to produce the final result.
Another approach is to use a programmable logic device, such as a field-programmable gate array (FPGA). An FPGA can be configured to perform the steps of Booth’s algorithm by programming the appropriate logic gates and interconnections. This approach has the advantage of being highly flexible and adaptable, but it may not be as efficient as a dedicated multiplier unit.
Regardless of the approach used, the hardware implementation of Booth’s algorithm typically follows the same steps as the algorithm itself, including the initial setup of the operands, the calculation of the partial products, and the final addition of the partial products to produce the final result.
Overall, the hardware implementation of Booth’s algorithm is an important component of many digital systems, as it allows for fast and accurate binary multiplication. By following the steps of the algorithm and using specialized hardware or programmable devices, it is possible to achieve reliable and efficient performance.
Flowchart of Booth’s Algorithm
Booth’s algorithm is a method for performing binary multiplication that involves a series of steps and decisions. The following flowchart outlines the basic steps of the algorithm:
- Begin by setting the value of the register “A” to the first operand and the value of the register “Q” to the second operand.
- Check the value of the least significant bit (LSB) of the register “Q.” If the LSB is a zero, skip to step 6. If the LSB is a one, perform the following actions:
- Check the sign of the value in the register “A.” If the sign is positive, add the value in the register “Q” to the register “A.” If the sign is negative, subtract the value in the register “Q” from the register “A.”
- Shift the value in the register “A” left one position.
- Shift the value in the register “Q” right one position.
- Check if the value in the register “Q” is equal to zero. If it is, the algorithm is complete and the value in the register “A” is the result. If the value in the register “Q” is not zero, go back to step 2.
Numerical Example
Multiply -11 (in binary: 1011) by 7 (in binary: 0111)
Thus, the product of -11 and 7 is -184.
Best Case and Worst Case Occurrence
The best case occurrence of Booth’s algorithm occurs when the least significant bit (LSB) of the register “Q” is a zero in every iteration. This means that the algorithm can skip the addition or subtraction step and proceed directly to the shifting steps, which reduces the overall number of steps required to complete the algorithm.
The worst case occurrence of Booth’s algorithm occurs when the LSB of the register “Q” is a one in every iteration. This means that the algorithm must perform the addition or subtraction step in every iteration, which increases the overall number of steps required to complete the algorithm.