Mastering Software Engineering: Diagrams, Models, and Testing Techniques
Introduction to Caesar Cipher Algorithm
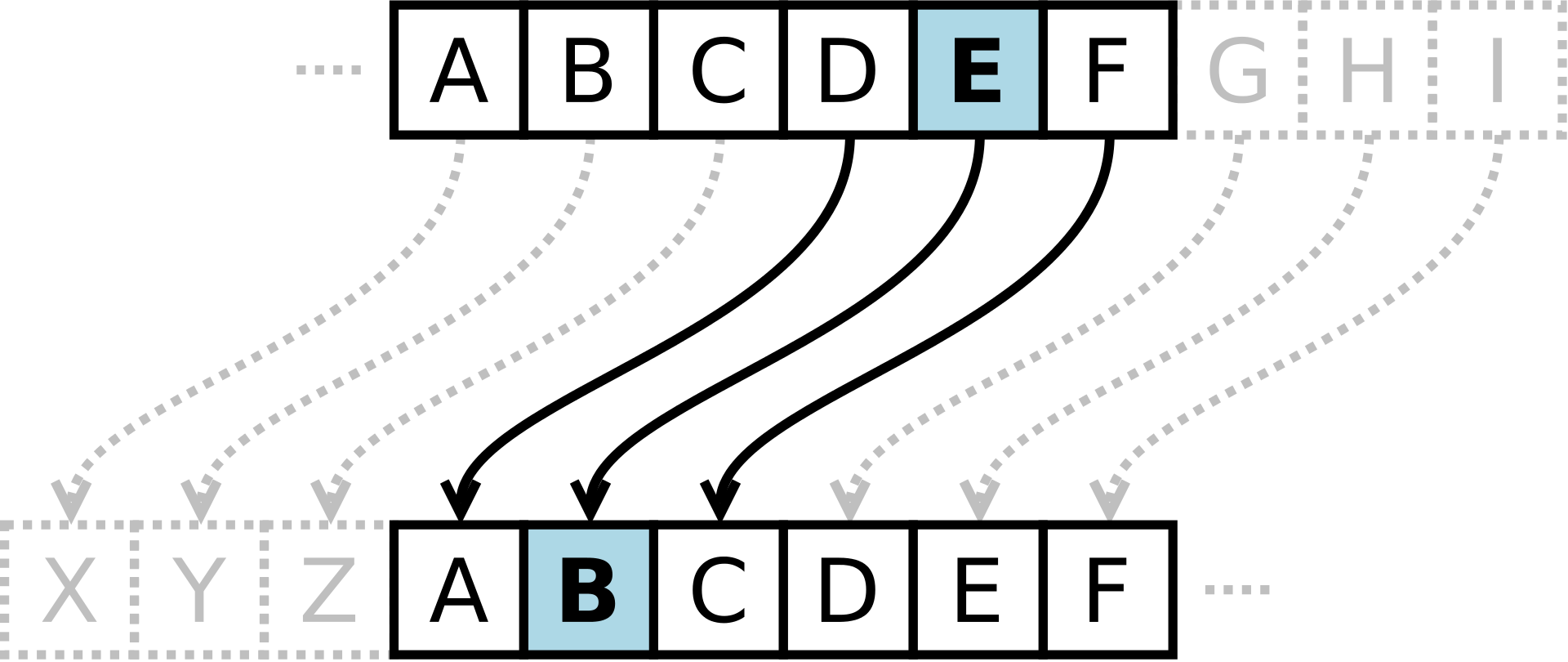
Introduction
In this article, we'll talk about the Caesar Cipher Algorithm and create a programme that uses it. One of the most well-known and straightforward encryption methods is the Caesar Cipher. Each letter in the plaintext is replaced by a letter that is located a certain number of places farther down the alphabet in this form of substitution cypher. With a left shift of 3, for instance, D would become A, E would become B, and so on. At the end, C++ code is also given for complete coverage.
Understanding Cyphers
A cypher (or cypher) in cryptography is a set of predetermined procedures that may be used as a technique to achieve encryption or decryption. Encipherment is a different, less often used word. Information is transformed into a code or cypher when it is encoded or enciphered. In everyday speech, the terms "cypher" and "code" are interchangeable since they both refer to a series of operations that encrypt a message; nevertheless, in cryptography, especially classical cryptography, the ideas are different.
While cyphers often replace the same amount of characters as are entered, codes typically replace varying length strings of characters in the output. There are exceptions, and certain cypher systems may emit a small number of characters more or less than they did when they were entered.
Encryption
INPUT:
- key (key) [ideally integer, between 0-25]
- message (s) [string or text message]
OUTPUT:
- Encrypted message (t)
CODE
Decryption
INPUT:
- key (key) [ideally integer, between 0-25]
- message (s) [string or text message]
OUTPUT:
- decrypted message (t)
CODE
Algorithm Complexity
Time complexity: O(N) -> N is the length of the given text Auxiliary space: O(N)
Advantages of Caesar Cipher
- Applying it is pretty simple.
- The simplest form of cryptography is this one.
- Its whole operation uses a single short key.
- It is ideal for a system if it doesn't employ sophisticated coding methods.
- It simply needs a little amount of CPU power.
Hope this article gives you a clear understanding of the Caesar Cipher Algorithm.