Mastering Software Engineering: Diagrams, Models, and Testing Techniques
SHA-1 Hash Algorithm
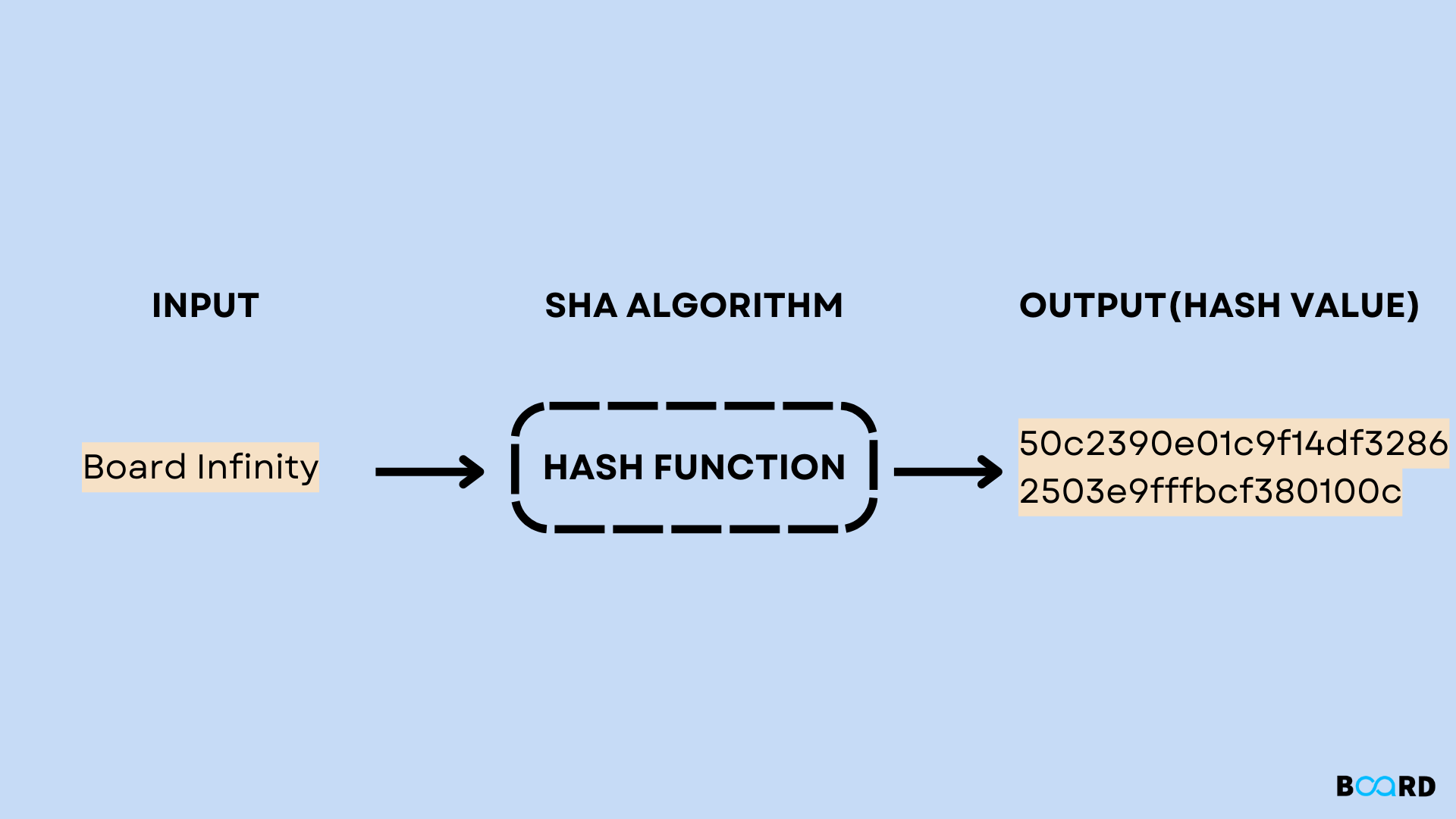
Introduction
SHA-1 or Secure Hash Algorithm is a cryptographic hash function that takes a string as an input and generates a hash value of 160bit or 20 bytes. The hash value is generally called a message digest. This message digest is generally altered into a 40-decimal long hexadecimal value. Many tech giants like Google, Microsoft, and Apple is now considered insecure by US Federal Agencies since 2005. For calculating the hash value i.e, Message Digest java uses a MessageDigestClass which is present under the package of java.util.package. These algorithms are initialized in a static method called getInstance(). After selecting the algorithm the message digest value is calculated and the results are returned as a byte array.
BigInteger class is used, to convert the resultant byte array into its signum representation. This representation is then converted into a hexadecimal format to get the expected MessageDigest.
Implementation
Below is the implementation of SHA -1 in java
The output obtained is as follows:
Conclusion
SHA-1 or Secure Hash Algorithm which generates the crtpographic hash value for the given input. This hash value is called message digest and it is 20 bytes long.