Understanding Algorithm: Complexity and Performance
Difference between Hashmap and HashTable
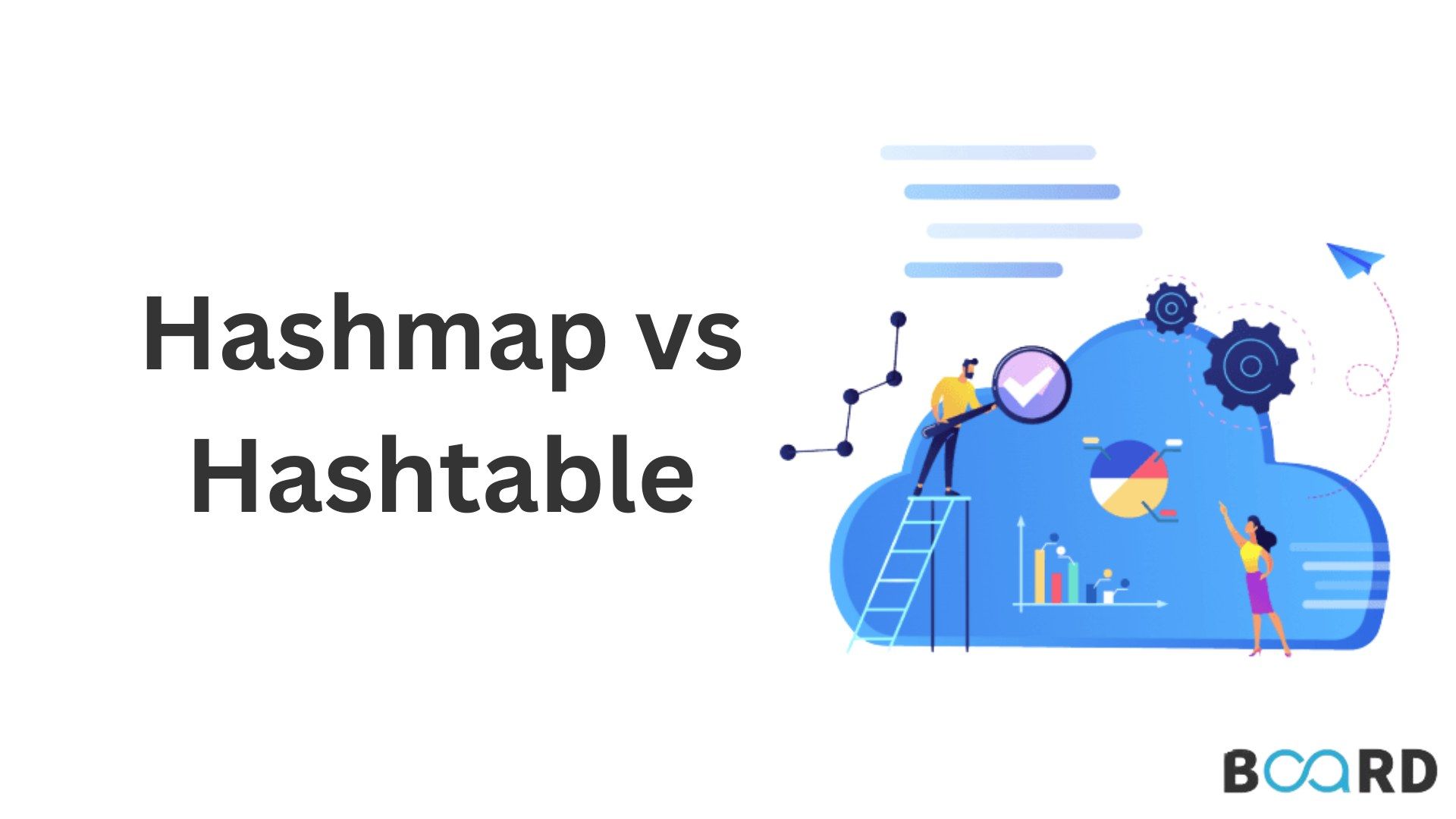
Introduction
In java, Hashmap and Hashtable use a hash table to store key and value pairs. When using either of them, a key is used which is an object and the value that is linked to the key. This key then is hashed. The result of the hashing is a hashcode which is then used as an index at which the value is stored within the table. Now before getting into the difference between the Hashmap and Hashtable, first let us discuss each of them.
HashMap
In Java, the Map interface lets the implementation of the Hashmap class, which permits the storage of the key and value pair, where the keys are unique. When the same key has been inserted the value of the inserted key is replaced with the value of the original key. Operations like updation, deletion, etc are easy using the index. The Hashmap class is found in the following java package.
In java, Hashmap is similar to the legacy Hashtable class, but not synchronized. It permits to also hold null elements, but there should only be a single null key. Since java version 5, the hashmap is denoted as HashMap<K, V>, where K is the key and V is the value. HashMap implements the Map interface. AbstractMap is inherited by HashMap.
HashTable
In Java, the hash table maps keys to values that are implemented by the HashTable class. In it, any object that is not null can be used as a key or as a value. The objects that are used as keys must implement the equals method and hashCode method to store and retrieve objects from a hashtable.
Some Important points
- It is similar to HashMap, but Unlike HashMap it is synchronized.
- HashTable uses a hash table to store key/value pairs.
- HashTable’s initial default capacity is 11 and its load factor capacity is 0.75.
- HashTable gives fail-fast Enumeration.
Differences between HashMap and HashTable in Java
Inside a hash table, HashMap and Hashtable store the key and value pairs in a hash table.
HashMap vs Hashtable
- Hashmap is non-synchronized. HashMap is not a thread-safe and because of this it can not be shared between multiple threads without proper synchronization1 of code on the other hand Hashtable is synchronized and is also thread-safe. So Hashtable can be shared with multiple threads.
- Hashmap permits only a single null key and multiple null values. On the other hand, Hashtable denies any null key or value.
- If thread synchronization is not required, then HashMap is preferred over Hashtable.
Difference Between Hashmap and Hashtable
S No. | HashMap | HashTable |
---|---|---|
1. | In HashMap, not a single method is synchronized. | Every method in Hashtable is synchronized. |
2. | In HashMap, multiple threads can run at the same time. hence it is not thread-safe. | In Hashtable, only one thread is allowed to run at a time. Hence it is thread-safe. |
3. | In it, threads do not wait, hence performance is high. | On the other hand, threads need to wait which makes the performance low. |
4. | HashMap does allow the storage of the null value for both key and values. | HashTable does not allow null values for both key and value because it will give null pointer exceptions. |
5. | Introduced in 1.2 Version. | Introduced in 1.0 Version. |
6. | It is a non-legacy. | It is a legacy |
Java program to demonstrate HashMap and HashTable
Code
Output