Understanding Algorithm: Complexity and Performance
What is Hashing in Data Structure?
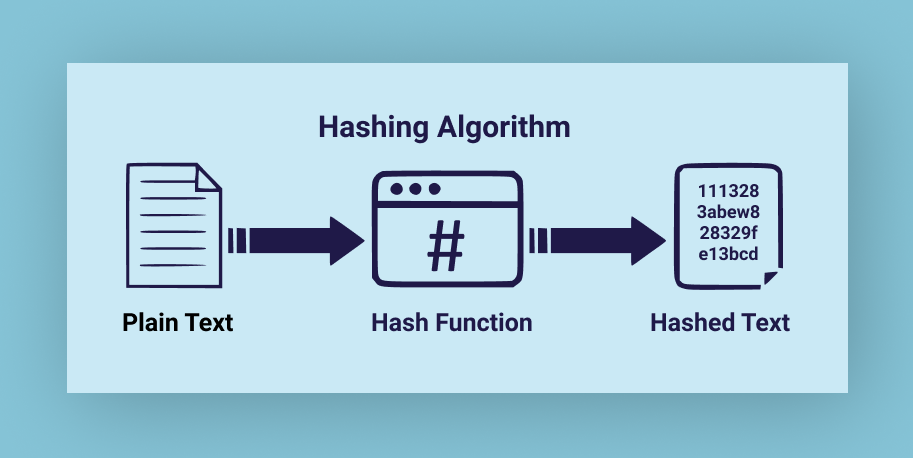
Introduction
An essential data structure called hashing was created to address the issue of quickly identifying and saving data in an array. With many examples, this blog will help you grasp the hash technique, hash tables, and terminology used with this data structure.
Hash Tables
An abstract data type is implemented in computer programming via an associative array, which is a structure called a hash table (ADT). A programmer simply has to be aware of the operations that may be carried out on an abstract data type; they are not required to understand the implementation specifics of the data type (such as how the data is kept in memory). In order to find the needed value from an array of buckets or slots, a hash table utilizes a hash function to construct an index into each one. Map-like data structures are implemented using hash tables. Modern computers heavily rely on hash tables to achieve many things, like dictionaries (as in Python), associative arrays (as in PHP), java hash tables, etc. Hash tables are often implemented as an array of values ordered by their keys in programming languages. The data is organized in memory, which speeds up lookup and insert/delete operations. Example:-
- A library has an endless supply of books. Each book is given a special number by the librarian. This distinctive number aids in locating the books on the bookcase.
Hash Function
A data structure's hash function converts data of any size to data of a specific size. It returns a tiny integer number (often referred to as a hash value), hash sums, and hash codes. The data structure uses several pretty intriguing hashing methods, like:
hash = hashfunc(key)
index = hash % array_size
The data structure's hash function has the following three characteristics:
- Free from Collision
- Hidden
- Non Reversible or guessable
Collision Resolution
In hashing, collisions are resolved using collision resolution algorithms. Chaining or open addressing are two collision resolution strategies. In chaining, the previous element is kept in place while the new element is inserted into the next empty space. Although it is a straightforward collision resolution technique, it performs poorly. In open addressing, the old element is swapped out for the new element, which is then designated as a collision.
Hashing Keys
These are different kinds of hashing in a data structure; the static hashing allows the user to search up words in the finalized dictionary, which cannot be altered. The dynamic dictionary, on the other hand, enables the data to be relocated according to demand as the data may be added or withdrawn. The foundation of the records is likewise altered by the outcome.
Public Key
It is a key that is accessible to others. It is frequently used for things like sending bitcoins and safeguarding online sessions. Since two keys are needed, the security remains uncompromised.
Private Key
It is a method of data encryption or decryption. For instance, let's say I email a password-protected encrypted file to a friend. The file would be decrypted by entering the passcode because my friend knows it. Private keys are effective since just one key is needed.
SSH Public Key
SSH uses both a public and a private key. SSH is a set of keys that may be used to authenticate and decode a communication sent from a distance. Both the distant servers and the stakeholders have access to the public key.
Applications of Hashing
Computer science uses hashing operations for a variety of purposes, including document fingerprinting and cryptography. A hashing function's principal objective is to convert enormous input volumes into outputs of a predetermined length. Hashing is a technique used in cryptography to make sure that a communication or document has not been tampered with. The hash value is also changed whenever the file or message is changed in any manner (even by a single character). It is therefore almost impossible to create a document or message with a given hash value.