Mastering ReactJS: Understanding Hooks, Components, and Libraries
Functional Components and Class Components in ReactJS
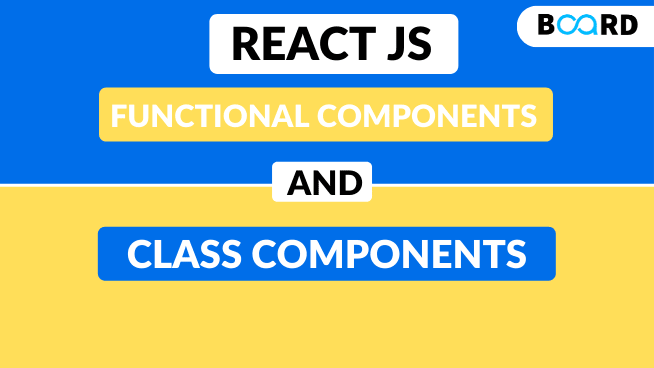
What is a Component?
Rather than building the whole UI under one single file, we can and should divide all the sections into smaller independent pieces. These are referred to as components in ReactJS.
- A Component can be described as one of the fundamental building blocks of React.
- The various applications developed using React are made up of pieces called components.
- Components facilitate the process of developing UI.
- A UI can be broken down into multiple individual pieces known as components and we can work on them independently as required.
- Then we can merge all the individual components to make a parent component which will act as the final UI.
Each component renders some JSX code and it defines which HTML code React should render to the real DOM in the end. JSX is not HTML but it looks a lot like it. In React we mainly have two types of components: Functional Components and Class Components.
Functional Components
The first and most recommended type of component in React is a functional component. A functional component is basically a JavaScript/ES6 function that returns a React element (JSX). According to React's official documentation, the function below is a valid functional component:
function Welcome(props) {
return <h1>Hello World, {props.name}</h1>;
}
Alternatively, the users can also create a functional component with the arrow function definition:
const Welcome = (props) => {
return <h1>Hello World, {props.name}</h1>;
}
The above function is a valid React component because it accepts a single “props” (which stands for properties) object argument with data and returns a React element.
To be able to use a component later, the users need to first export it so that they can import it somewhere else:
function Welcome(props) {
return <h1>Hello World, {props.name}</h1>;
}
export default Welcome;
After importing it, the users can call the component as shown in this example:
import Welcome from './Welcome';
function App() {
return (
<div className="App">
<Welcome />
</div>);
}
So a Functional Component in React can be summarized as:
- It is a JavaScript/ES6 function.
- It must return a React element (JSX).
- It always starts with a capital letter according to the naming convention.
- It takes props as a parameter if necessary.
Class Components
The second type of React Components is the Class component. Class components are ES6 classes that return JSX. Below, we can see our same Welcome function, but this time as a class component:
class Welcome extends React.Component {
render() {
return <h1>Hello, {this.props.name}</h1>;
}
}
Different from functional components, class components must have an additional render( ) method for returning JSX.
Why Are Class Components Needed?
Class Components are mandatory while working with "state". In the older versions of React (version < 16.8), it was not possible to use state inside functional components.
Therefore, we needed functional components for rendering UI only, whereas we would use class components for data management and some additional operations (like life-cycle methods). This has changed considerably with the introduction of React Hooks, and now we can also use states in functional components as well.
A Class Component can be summarized as follows:
- It is an ES6 class and will be a component once it ‘extends’ a React component.
- It takes Props (in the constructor) if needed.
- It must have a render( ) ****method for returning JSX.
Functional Components vs Class Components
- A functional component is considered as a plain JavaScript function that accepts props as an argument and returns a React element. On the other hand, a class component requires the users to extend from React. Component and create a render function that returns a React element.
- There is no render method used in functional components, whereas, the class components must have the render() method returning HTML.
- React lifecycle methods like componentDidMount, cannot be used in functional components. On the contrary, React lifecycle methods can be used inside class components like componentDidMount.
- Class Components are also known as Stateless components as they simply accept data and display them in some form, and they are mainly responsible for rendering UI. On the other hand, Functional Components are also known as Stateful components as they implement logic and state.
Summary
- In this article, we explored the concepts of Functional Components and Class Components in ReactJS along with their examples.
- We saw the various scenarios which may demand the use of these components.
- We also explored the various differences between these two concepts.
- We should select one of them according to the situation and based on the type of application we want to develop.