Mastering ReactJS: Understanding Hooks, Components, and Libraries
A Quick Guide to React Router DOM
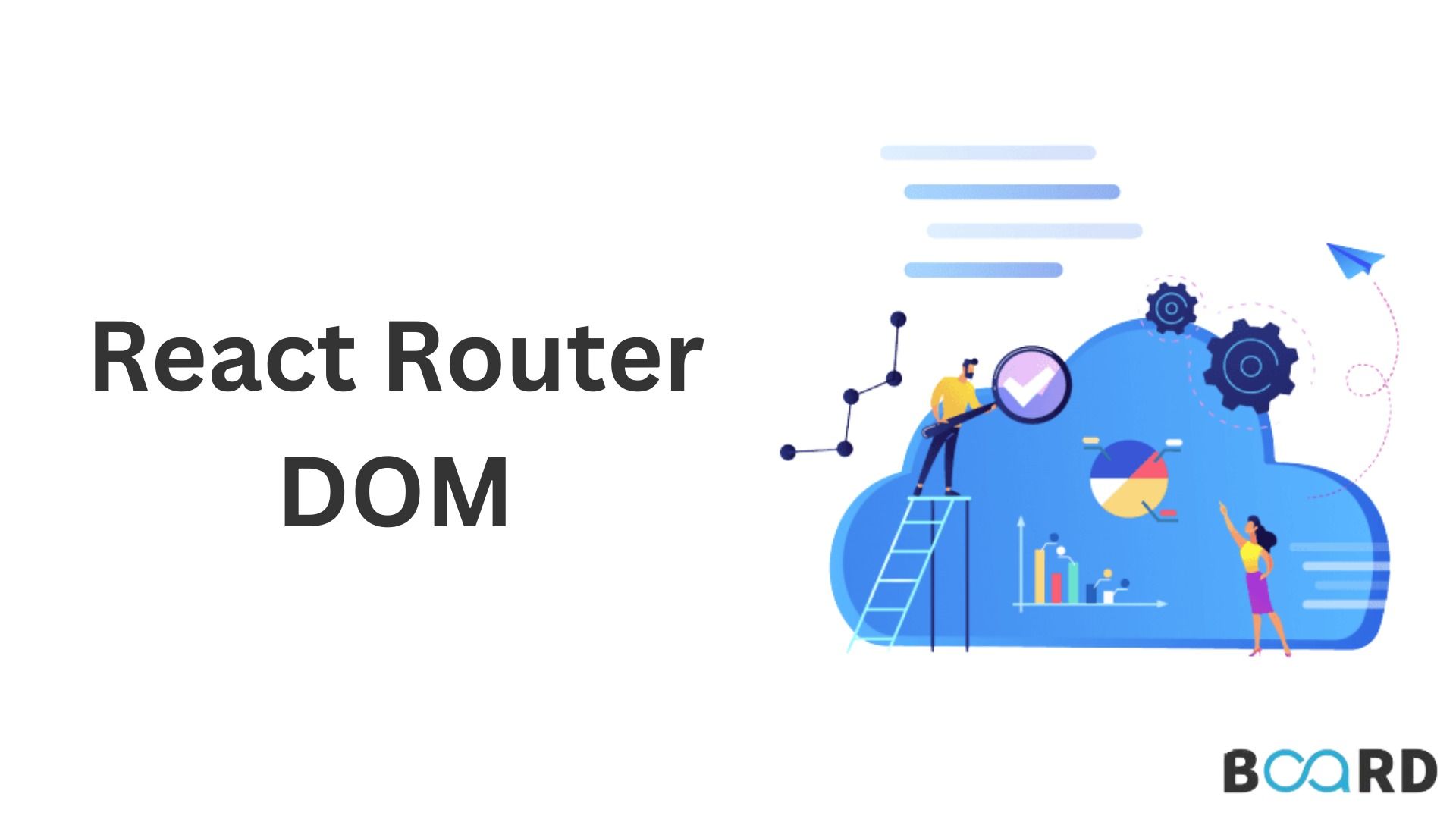
React Router DOM
The process of routing involves the direction of a user to a different page based on their actions. One of the most frequent uses of ReactJS Router is for developing Single Page Web Applications. The application uses React Router to define multiple routes.
React Router is a standard library system that allows users to create routing within React applications using the React Router package. It creates the synchronous URL on the browser with web page data.
The main use of this framework is to develop single-page web applications that maintain application structure and behavior. React Router plays a crucial role in displaying multiple views, In a single page application. A React application cannot show multiple views without React Router.
Many social media websites like Facebook and Instagram render multiple views using React Router.
Quick Start
React Application Setup:
Create a React app using create-react-app and name it boardinfinity.
You can use the following command to create react application
React Router Installation
There are three different routing packages in React. These are:
react-router:
React Router applications rely on it for core routing components and functions.
react-router-native:
It is useful for mobile applications.
react-router-dom:
It is useful for designing Web applications.
Directly installing react-router in your application is not possible. The first step to using react routing is to install react-router-dom modules in your application. Installing react-router dom is as simple as running the following command.
Creating a few pages in the react application will help us learn how to use React Router. Within your src folder, create a folder called pages and add two files named home.js and about.js.
Home.js
About.js
In the app.js file, add all the necessary components. This is what app.js will look like
After saving and running your application it will open new browser window which will look like this.
And our About Us page will look like this
Conclusion
This collection of examples should help you get a feel for how React Router creates navigation. Read on to discover what React Router is made up of!