Mastering ReactJS: Understanding Hooks, Components, and Libraries
React Functional Components: Introduction
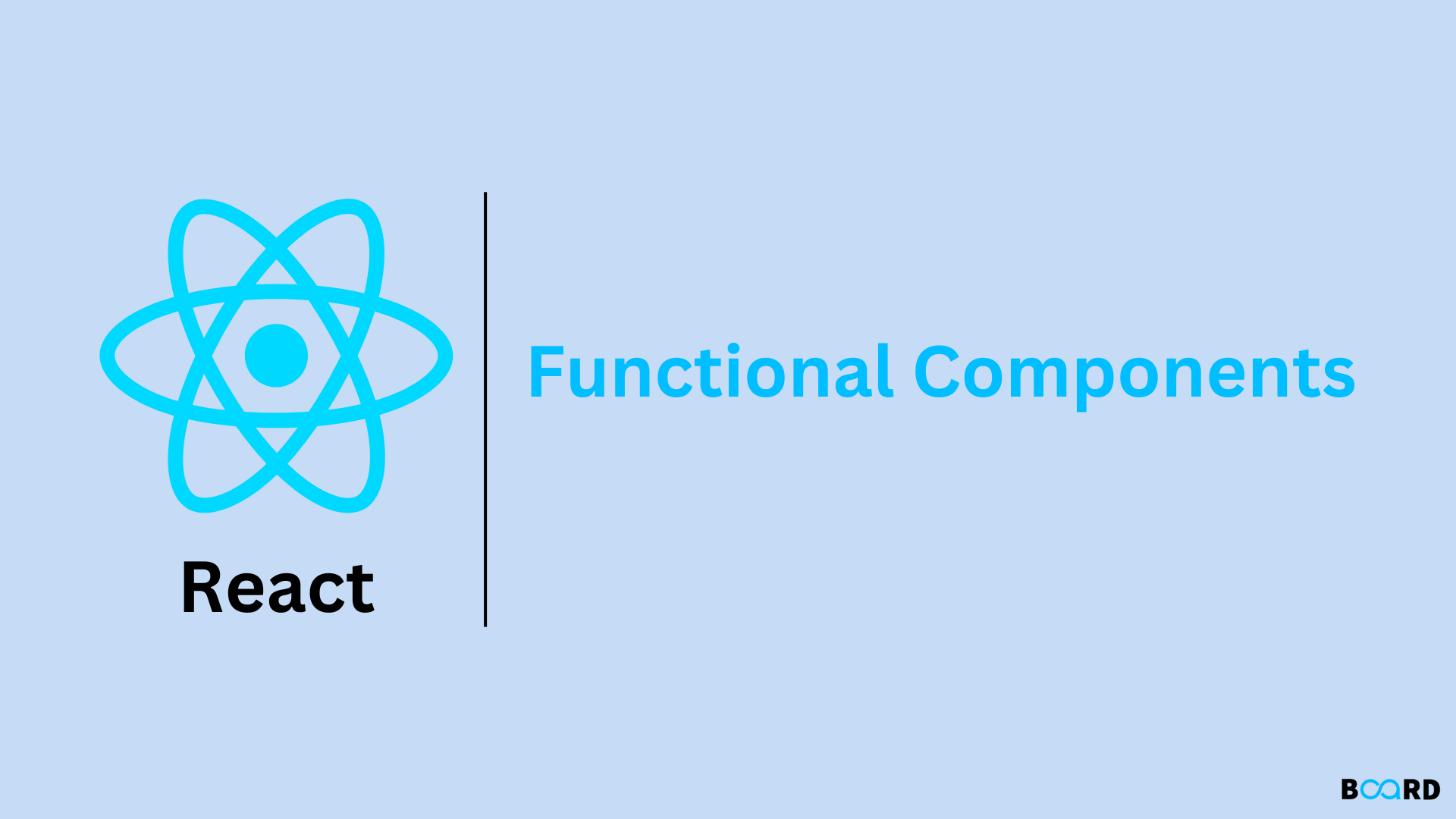
Developers who work in React probably know about React components. Any React application relies on it as its core building block. There is at least one base component in every react application.
This article provides a comprehensive overview of react functional components.
What Are React Components?
A react application consists of react components. As we discussed above, react components are the foundation of any react application. They are reusable and independent components. The react component helps you can break up the entire view into sections. React components define the idea and business logic for each view.
React components can get divided into two types: class-based and functional. A class-based component is "stateful," while a functional component is "stateless.".
Introduction to React Functional Components
React functional components are simply JavaScript functions that can get created using specific function keywords. It is most common for developers to use the Arrow function when creating functional components.
Rendering the view and data to the browser is the primary responsibility of the functional component. In other words, functional components accept data, whereas technically, props are passed as function arguments and exported as valid JSX.
How To Create A Simple Functional Component?
Functional components are pretty simple to define; as already mentioned, they are simply functions that return valid React elements. The first step is to create a boilerplate for react using the following command:
Output:
It is a simple functional component that returns static text.
Note: ES6's arrow function is also available for creating functional components.
A React Functional Component Using Props
A react functional component is a pure javascript function. The function takes an object called props (which stands for properties) and returns JSX formatted data. The following code will help us understand this.
Inside src, create a folder named components. Here we will write all our components and then import them into App.js. It is just a convention used by all react developers. In the components folder, create a file called Employee.js:
Here is an example code of App.js:
Here, we are using props to display employee information. App.js has included the Employee component and passed its information as attributes. We will send this information to the employee component as props, an object that contains a name and age field, and we can use this information wherever we like.
Functional Components and React’s future
The simplicity of functional components is an advantage over class-based components, and with hooks, they offer the same functionality. More and more developers will use functional components in the future since there is no compelling reason to use two different syntaxes.