Mastering ReactJS: Understanding Hooks, Components, and Libraries
Working of Axios in React
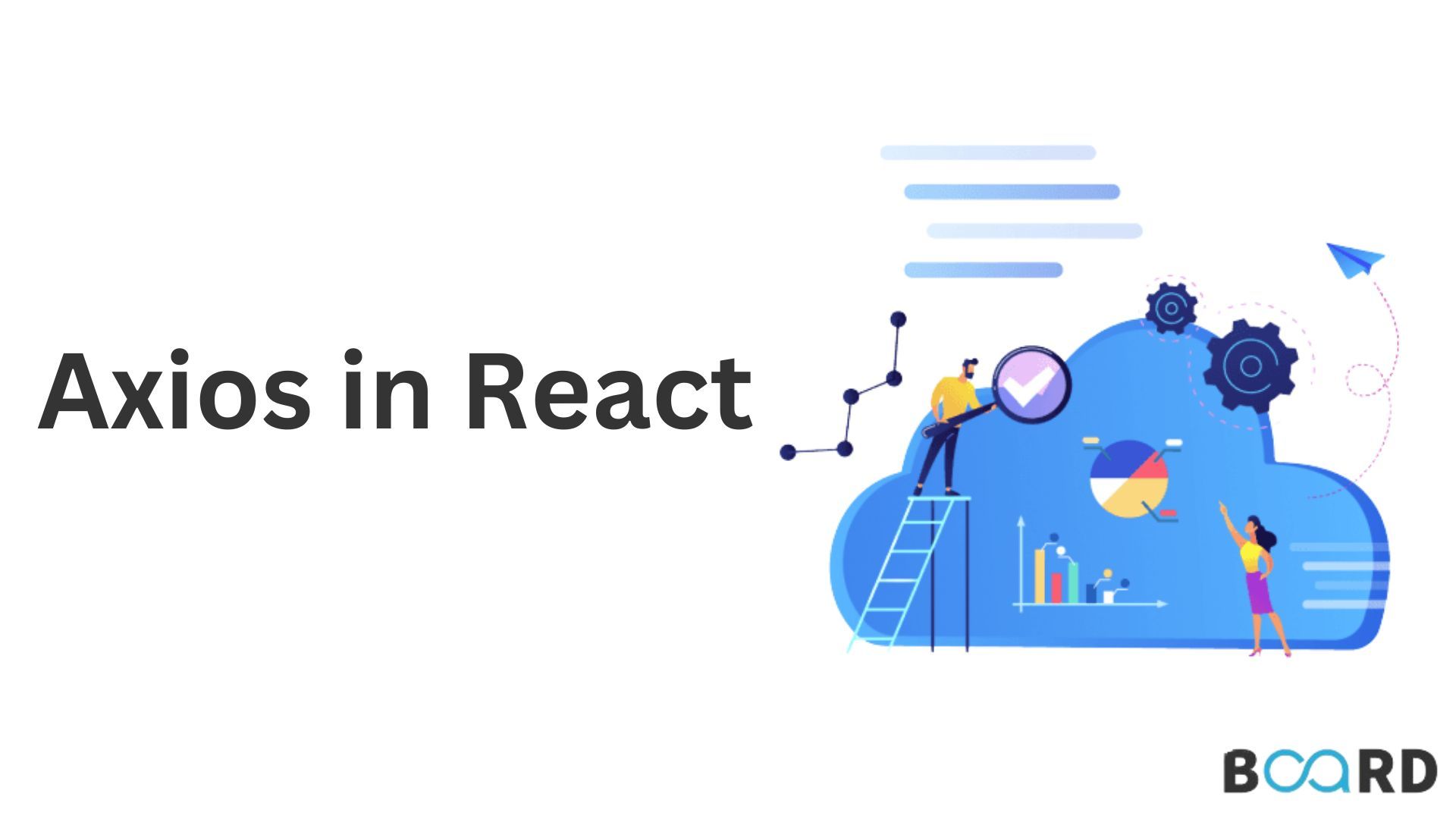
Introduction
Axios is a promise-based HTTP client library. With it, you can send asynchronous HTTP requests to REST endpoints and perform CRUD operations. You can use an external API, such as Google API or GitHub API, or make your own Node.js backend server for this REST endpoint/API.
This article aims to demonstrate how to make Axios API requests in React using the GET, POST, and DELETE methods.
What is Axios?
As part of the backend communication, Axios is used, which supports the native Promise API for JS ES6. Â Essentially, it allows us to request data from an API, receive that data in our React application, and then do something in response. It allows you to make HTTP requests using the browser with Axios, which has over 78k stars on Github.
Why Use Axios in React
When it comes to making these requests, there are several libraries you can pick. So why choose Axios? To make HTTP requests, you should use Axios for the following five reasons:
- JSON data can be handled well by its default settings. There is rarely a need to set headers, unlike alternatives such as the Fetch API. Or convert the body of your request into JSON.
- There are function names in Axios that match any HTTP method. For instance, with .get(), you can perform GET requests.
- With Axios, you can do more with less code. You only need one .then() callback for accessing the requested JSON data.
- Axios comes with better error handling. Axios raises 400 and 500-range errors for you. This is in contrast to the Fetch API, where you have to check the status code and throw an error.
- Axios works well on both servers as well as client-side machines. You can use Axios in a separate environment from the browser if you're writing a Node.js application.
Setting Up Axios with React
React and Axios work very well together. Three things are needed:
- Having an existing React project
- Using npm/yarn to install Axios
- API endpoints for making requests
For existing React projects, install Axios using npm (or another package manager):
By running this command, you will install axios into your directory. You can now perform certain activities related to the Axios library.
GET request with Axios
By using the useEffect() hook, you can use GET requests to get data from an endpoint immediately after the app renders. By attaching the .get() method to the variable, we can make a GET request to our API/endpoint. In the next step, we will use a .then() callback to retrieve all the response data, since the baseURL is already assigned to the variable (client).
POST Request with Axios
Endpoints receive data through POST requests. This method works similarly to GET requests, but when the form is submitted, it will trigger the function. By spreading the previous data and then adding new data, this method sends data into the state from an object:
DELETE Request with Axios
With DELETE, you can delete a specific chunk of data from your endpoint/API. This is how the Delete request will look:
Conclusion
Axios is a promise-based HTTP client for the browser and a node.js library for all server frameworks. Easily put, it makes handling API requests a breeze. No more working with XMLHttpRequest, simply pass in a configuration object and call Axios with whatever API method you need to execute.