Mastering ReactJS: Understanding Hooks, Components, and Libraries
Working with React Router
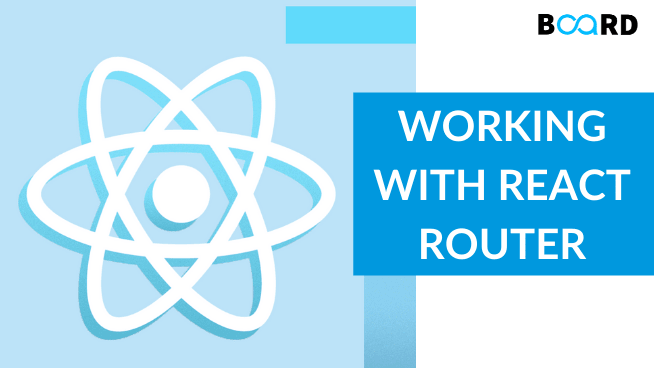
1. What is React Router?
React Router is considered the standard library for routing in React which allows the navigation among views of various components in any React Application, enables changing the browser URL, and keeps the UI in sync with the URL.
In order to understand how the React router operates, let us create a simple application to React. The application will contain three components, namely, the home component, the about component, and the contact component. We will be using React Router to navigate between these components.
2. Installing React Router
React Router can be installed via npm ****in the React application. Follow the below steps to install Router in any React application:
- Step 1: Create a react application by the following command.
npx create-react-app demo-router
- Step 2: Use cd to go to the project directory i.e., demo-router.
- Step 3: To install the React Router use the following command:
npm install react-router-dom –save
3. Components of React Router
The major components of the React Router are:
- BrowserRouter: BrowserRouter is actually a router implementation that uses the HTML5 history API i.e., pushState, replaceState and the popstate event in order to keep the UI in sync with the URL. It is the parent component that is used to store all of the other components.
- Route: Route is basically the conditionally shown component that renders some UI when its path matches the current URL.
- Link: The Link component is used to create links to different routes and implement navigation around the application and it works as an HTML anchor tag.
- Switch: The Switch component is used to render only the first route that matches the location rather than rendering all the matching routes. Although there is no defying functionality of the SWITCH tag in our example application as none of the LINK paths are ever going to coincide. But let’s say we have a route, then all the Route tags are going to be processed which starts with ‘/’. This is when we need the SWITCH statement to process only one of the statements.
4. Using React Router
To use the React Router, let us first create a few components in the react application. In our project directory, let us create a folder named component inside the src folder and now add three more files named home.js, about.js and contact.js to the component folder.
After creating these files, let us add some code to our components:
Home Component
import React from 'react';
function Home (){
return <h1>Welcome to the Demo Routing App!</h1>
}
export default Home;
About Component
import React from 'react';
function About () {
return <div>
<h2>It shows a demo!</h2>
</div>
}
export default About;
Contact Component
import React from 'react';
function Contact (){
return <div>
<h2>Phone : 98XXXXXXX22</h2>
</div>
}
export default Contact;
Now that we have created our components, let us include React Router components in the application in the following manner:
- BrowserRouter: Add BrowserRouter aliased as Router to the app.js file in order to wrap all the other components. BrowserRouter acts as a parent component and can have only a single child.
class App extends Component {
render() {
return (
<Router>
<div className="App">
</div>
</Router>
);
}
}
- Link: Let us now create links to our components. The link component uses the to prop to describe the location where the links should navigate to.
<div className="App">
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About Us</Link>
</li>
<li>
<Link to="/contact">Contact Us</Link>
</li>
</ul>
</div>
Now, let us run the application on the local host and click on the links that we created. We will notice the URL changing according to the value in the props of the Link component.
- Route: The route component will now help us to establish the link between the component’s UI and the URL. To include routes to the application, let us add the code shown below to the app.js.
<Route exact path='/' component={Home}></Route>
<Route exact path='/about' component={About}></Route>
<Route exact path='/contact' component={Contact}></Route>
- Switch: In order to render a single component, wrap all the routes inside the Switch Component. Switch groups together several routes, iterates over them and finds the first one that matches the path. Thereby, the corresponding component to the path is rendered.
<Switch>
<Route exact path='/' component={Home}></Route>
<Route exact path='/about' component={About}></Route>
<Route exact path='/contact' component={Contact}></Route>
</Switch>
5. Summary
- In this article, we learnt the concept of Routing in ReactJS along with its various components like Browser Router, Link and others.
- We also learnt how to install React Router in any React application.
- We also understood the actual working with React Router by creating a demo application and including all the components.