Mastering Pandas: Practical Data Handling in Python
How to Get Column Names in Pandas?
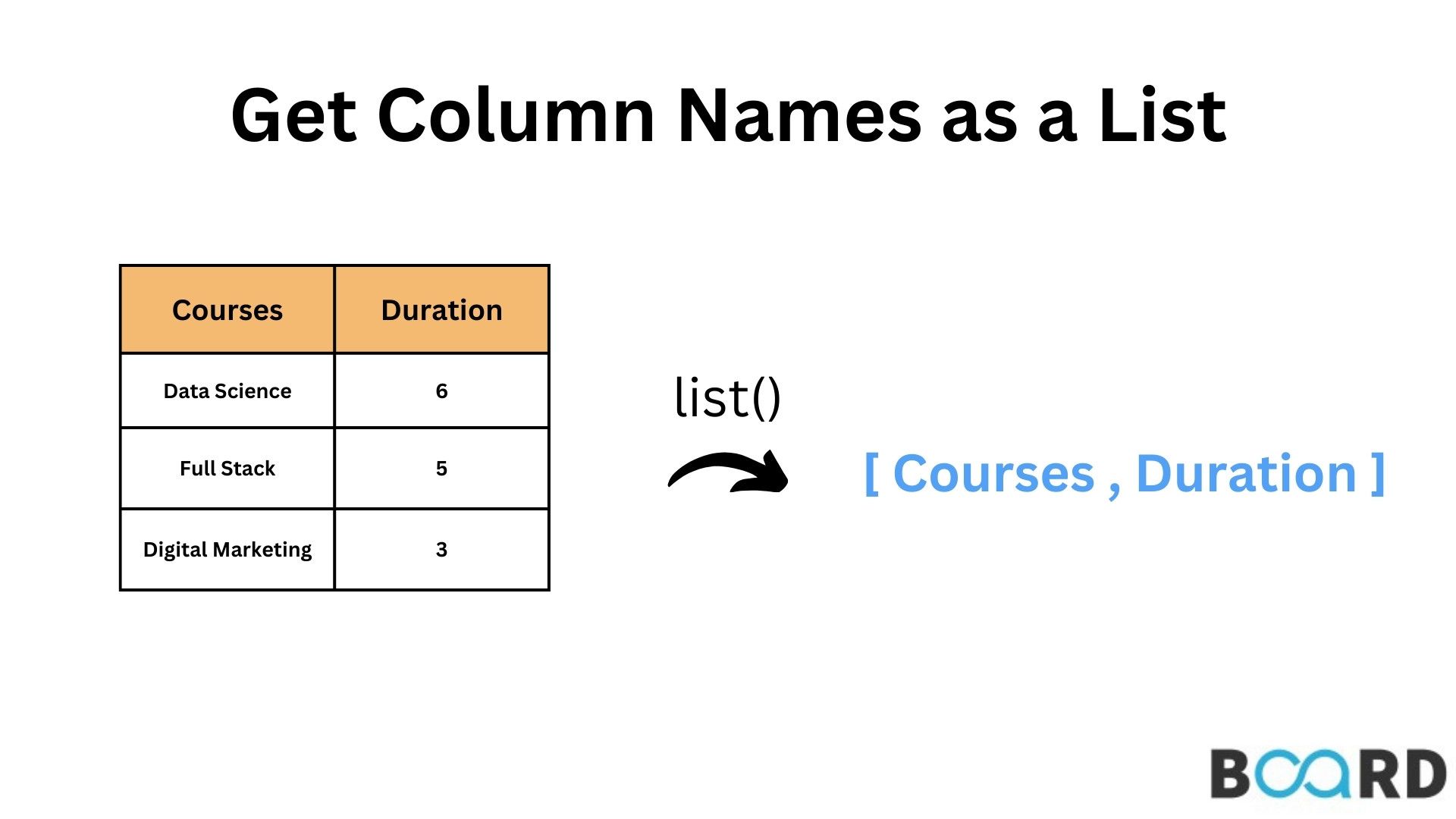
Introduction
In this article, we will learn how to get the column name from a given dataset in pandas. We have previously learned that Pandas is a Python library used for working with data sets. It offers tools for data exploration, cleaning, analysis, and manipulation.
How to get or print Pandas DataFrame Column Names?
DataFrame.columns.values method can be used to obtain the Pandas DataFrame Column Names, and to-list can be used to obtain the names as a list (). Each column in a Pandas DataFrame has a label or name that indicates the kind of value the column contains or represents. Obtaining the column names is helpful if you wish to change the values of all columns or have programmatic access to all columns by name. I'll describe various approaches to extracting column names from Pandas DataFrame headers in this article along with examples.
Use DataFrame.columns.values.tolist() to retrieve a list of columns from the DataFrame header. The statement's many parts are individually explained below.
- .columns returns an Index object with column names. This preserves the order of column names.
- .columns.values returns an array and this has a helper function .tolist() that returns a list of column names.
The examples that follow show you how to quickly retrieve column names from a pandas DataFrame. To output it to the terminal, simply use the print() statement.
Create a Pandas DataFrame from Dict with a few rows and with columns names Courses, Fee, Duration, and Discount.
pandas Get Column Names
With the help of the Python list() function, you can obtain the column names from a Pandas DataFrame. Once you have the data, you can print it using the print() statement. This statement's df.columns attribute produces an Index object, a fundamental object that contains axis labels, so let me briefly explain what is happening. In our example, the property Index.values of the Index object gives column names in an array of data.
Keep in mind that df.columns maintains the columns' original arrangement. Either one can be used to turn an array of column names into a list.
Use list or toList() on an array object (array object).
Yields below output.
You can also use df.columns.values.tolist() to get the DataFrame column names.
Get Column Names in Sorting order
Use the sorted(df) function to obtain a list of column names in sorted order. column names are returned by this function in alphabetical order.
Yields below output. Notice the difference of output from above.
Access All Column Names by Iterating
You can perform the iteration over all columns and application of a function as shown below.
Yields below output.
Example of pandas Get Columns Names
Conclusion
In this article, you learned how to get or print the column names using the functions df.columns, list(df), and df.keys. You also learned how to get the names of all the integer columns and how to get the column names sorted, among other things.