Mastering Pandas: Practical Data Handling in Python
Converting List to DataFrames in Pandas
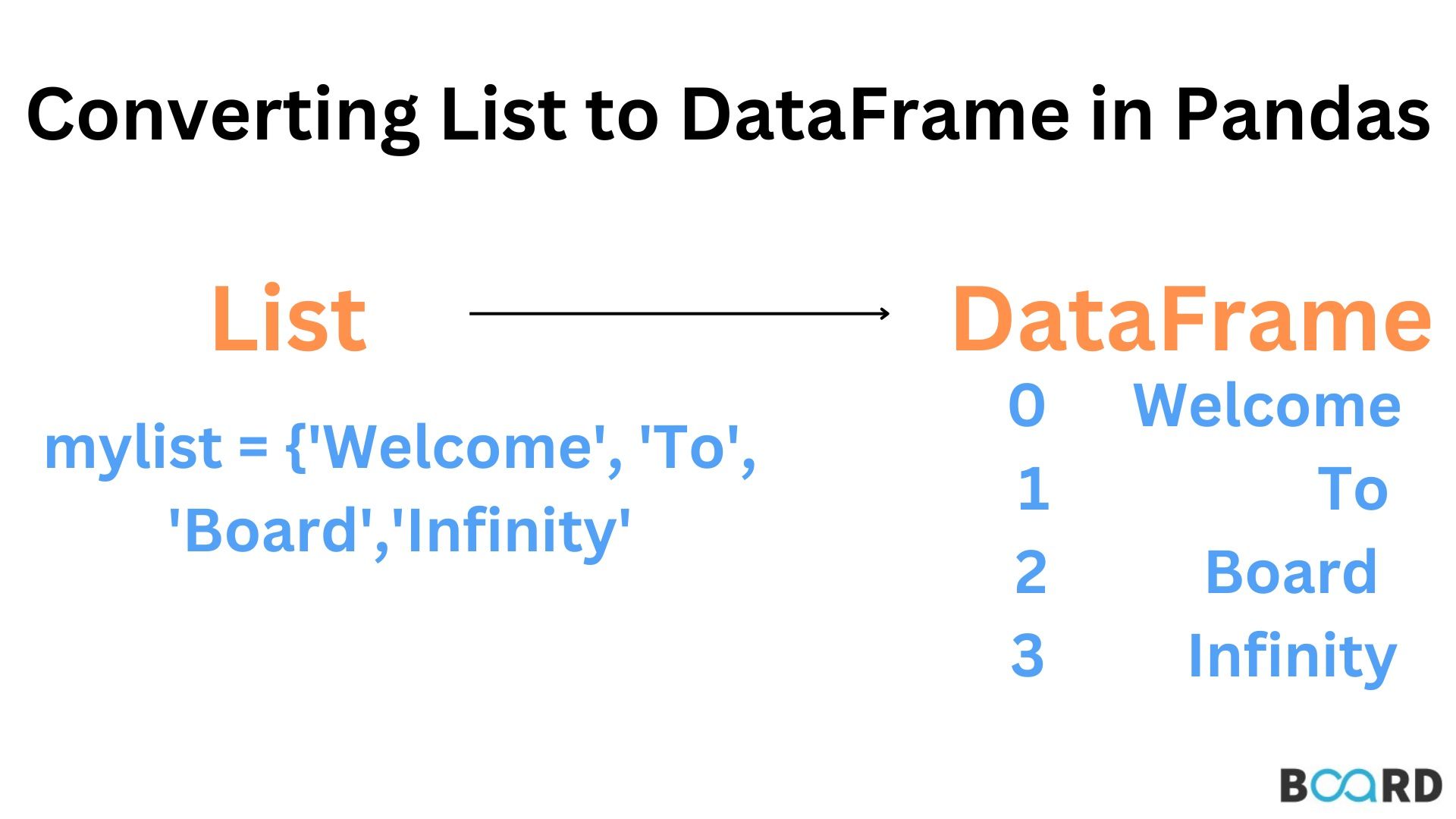
Introduction
Your numpy arrays might need to be transformed into a DataFrame. Or perhaps, when using standard Python, you have a list of values and wish to convert them into a DataFrame using Pandas. List to dataframe can be used in this situation.
It's important to send lists that represent rows when passing multiple lists.
Pandas List To DataFrame Parameters
You only need to care about those arguments since generating a DataFrame from a list utilizes the normal pd.DataFrame() method.
- data = The data that will be used to build your DataFrame. It will be the list(s) you pass in this situation. Every list corresponds to a row in your upcoming DataFrame.
- index = An index that is the same length as the quantity of lists you pass is acceptable. Consider indexes as row labels.
- columns = Names of your columns. This list of columns' length must correspond to the amount of items in your child lists.
The exciting part is now to examine a code example.
Pandas List To DataFrame
A list or list of lists may be used to build a DataFrame. In this situation, all you have to do is pass your data when calling the general pd.DataFrame() function.
We'll give you these three instances:
- From a single list, a DataFrame is created
- Assembling a DataFrame from a number of lists
- Assembling a DataFrame from many lists and naming the columns
- Multiple lists are combined into one DataFrame, with column names and indexes specified.
But let's first make some lists.
Creating a DataFrame from a single list
Let's begin by building a DataFrame from a single list. To do this, I'll call pd.DataFrame and provide data=my list. As you can see, pandas provides a single column DataFrame when I feed it one list. The row inside a single column represents the list values.
Creating a DataFrame from multiple lists
You just need to provide a list of lists into data to generate a DataFrame with numerous rows, which is the most common way to do it.
Assembling a DataFrame from several lists and naming the columns
Let's now provide the column names. Give the column parameter your column names. Check to see if the specified number of columns matches the number of columns in your DataFrame.
Multiple lists are combined into one DataFrame, with column names and indexes specified.
Let's finally make a DataFrame with a certain index. I'll do this by passing the index argument a list of index labels.