Mastering Pandas: Practical Data Handling in Python
Things to do with OpenCV
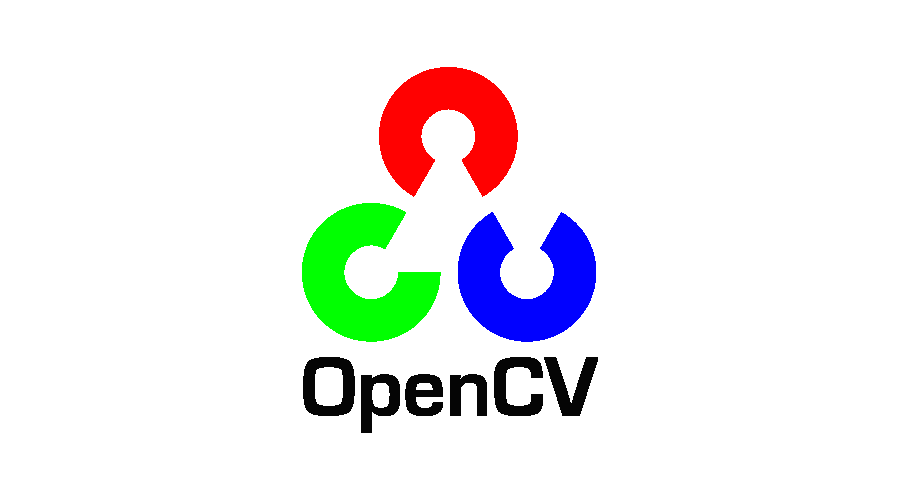
Introduction
Welcome to this post on OpenCV, a Python library for image and video processing. OpenCV is used for a wide range of image and video analysis tasks, including facial identification and detection, license plate reading, picture editing, sophisticated computer vision, optical character recognition and many more. Here, several Python examples will be worked through.
Installation
Install the packages using pip so you can start working with the OpenCV Library. For the sake of this tutorial, the basic package will suffice.
# Basic package
pip install opencv-python
# Full package
pip install opencv-contrib-python
To utilize OpenCV in all of your programmes where you want to alter or recognise image-based inputs, you must do the following.
- import cv2
- import matplotlib
- import numpy
Features
In order to properly analyze images and videos, it is important to first comprehend a few fundamental presumptions and paradigms.
- Almost all modern video cameras record in a manner that results in recordings that are really frames that are played back 30 to 60 times or more per second. However, they are fundamentally static frames, much like photographs. As a result, most of the techniques used in image identification and video analysis are the same.
- While certain tasks, like directional tracking, need a series of images (frames), others, like facial detection or object recognition, may be carried out using almost the same code on both still photos and moving pictures.
- Next, compressing the input as much as you can is key to a lot of picture and video analysis. A transformation to grayscale is nearly often the first step, although it can also be a color filter, gradient, or a mix of these.
- We may do a variety of analyses and source manipulations from here. Typically, a transformation is performed, followed by analysis, and then any overlays we choose to add are put to the original source.
- This is why you may frequently see the "final result" of perhaps object or facial identification being exhibited on a comprehensive image or video.
Read & Save Images
Now, OpenCV has to be able to read images in order to function on any picture. Here, we'll look at how to read a file and then save it after we're done. Let's examine the procedure:
imread() function in OpenCV
To read photos, we employ the imread function. The syntax for this function is as follows:
cv2.imread(path, flag)
The path of the picture to be read is represented by a string that is supplied as the path argument. The file must be in the working directory, or the complete path to the picture must be provided. The flag, which is used to designate how our picture should be read, is the other argument. The values that it could take and how they function are as follows:
cv2.IMREAD_COLOR: It states that the picture should be converted to a 3-channel BGR colour image. Any picture transparency will be disregarded. This is the standard flag. For this flag, we may also pass the integer value 1.
cv2.IMREAD_GRAYSCALE: It states that a picture should be converted to a single-channel grayscale image. As an alternative, we can set this flag's integer value to 0.
cv2.IMREAD_UNCHANGED: It directs the loading of a picture with the alpha channel present. As an alternative, we can set this flag's integer value to 1.
The imread() function typically produces an image that has been loaded from the given file, but in the event that this is not possible due to an unsupported file format, a missing file, an unsupported or corrupted format, or any other reason, it simply returns a matrix.
Code
Examples
- Reading, Writing and Displaying Images
2. Changing Color Spaces
3. Image Resizing
4. Edge Selection
5. Adaptive Thresholding
More advanced features, applications and explanations are covered in other Board Infinity blog posts. Do check them out if you are curious about the OpenCV Python Library.