Mastering Pandas: Practical Data Handling in Python
NumPy Broadcasting
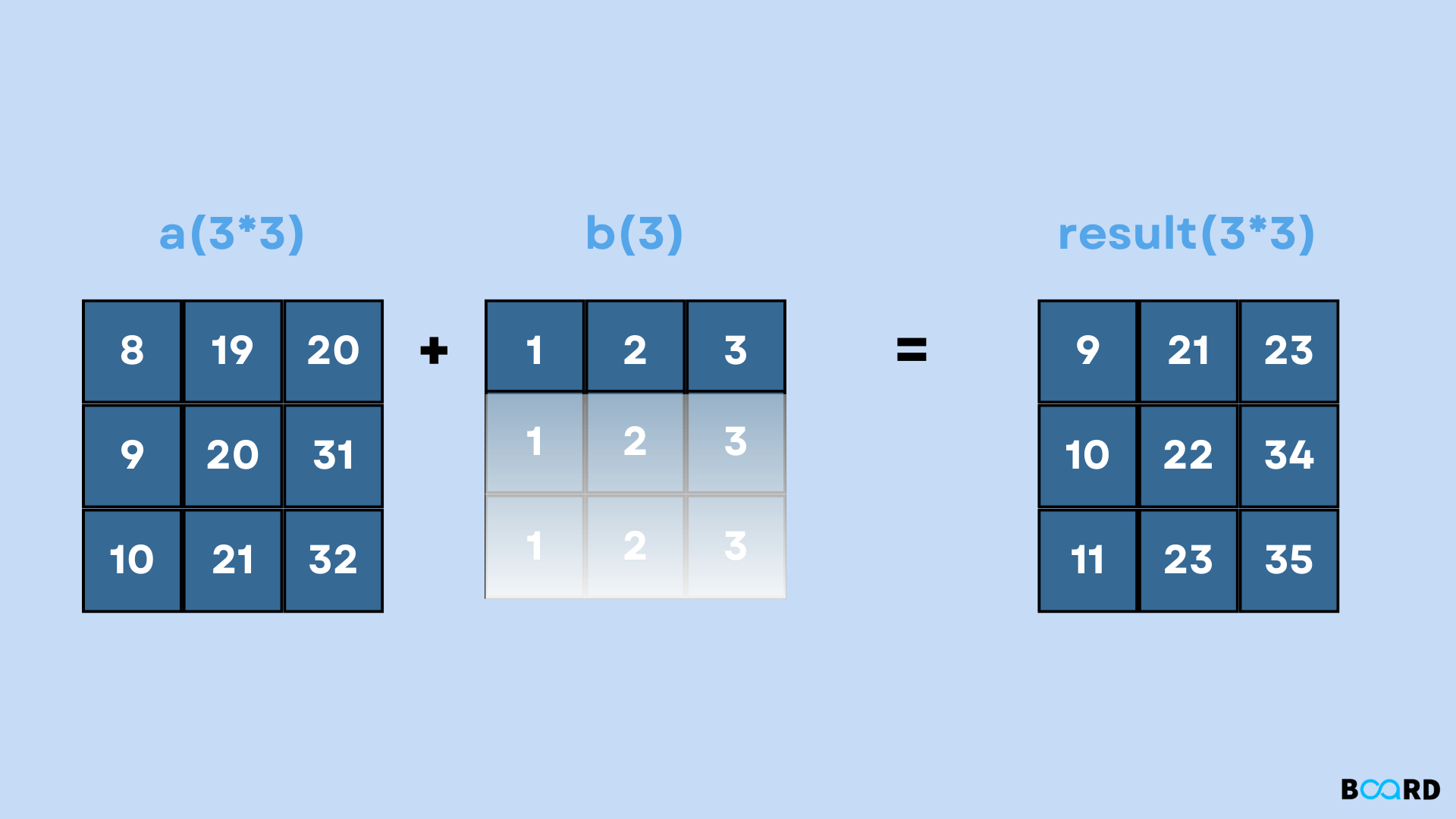
Overview
Numpy broadcasting is a powerful feature that allows numpy arrays with different shapes to be used together in arithmetic operations. It works by “stretching” or “duplicating” the smaller array along certain dimensions so that both arrays have the same shape. This makes it possible to perform element-wise operations between the arrays, which can be very useful in scientific computing and data analysis.
Broadcasting is particularly useful when working with arrays of different sizes, such as when you want to normalize an array by subtracting the mean and dividing by the standard deviation, or when you want to compute the dot product of two arrays. It can also be used to perform element-wise operations on multi-dimensional arrays, such as matrix multiplication or element-wise addition and subtraction.
The rules for broadcasting are simple: the shapes of the two arrays must be compatible, which means that the dimensions of the smaller array must either be 1 or match the corresponding dimension of the larger array. For example, if you have a 2D array of shape (3, 4) and a 1D array of shape (4), you can broadcast the 1D array to the 2D array by duplicating it along the first dimension. The result will be a 2D array of shape (3, 4).
You can also broadcast two arrays of the same shape. In this case, the operation will be performed element-wise, which means that the result will be an array with the same shape as the original arrays.
Broadcasting is a very efficient way to perform element-wise operations on arrays, as it avoids the overhead of looping over the elements of the arrays. It is also much faster than using Python loops or list comprehensions, as it takes advantage of the optimized C code that underlies numpy.
Here is an example of how to use broadcasting in numpy:
Implementation
Output
Summary
In summary, numpy broadcasting is a powerful feature that makes it easy to perform element-wise operations on arrays of different shapes. It is an essential tool for scientific computing and data analysis, and it is much faster and more efficient than using Python loops or list comprehensions.