Mastering Advanced Python Concepts
Lambda function in Python
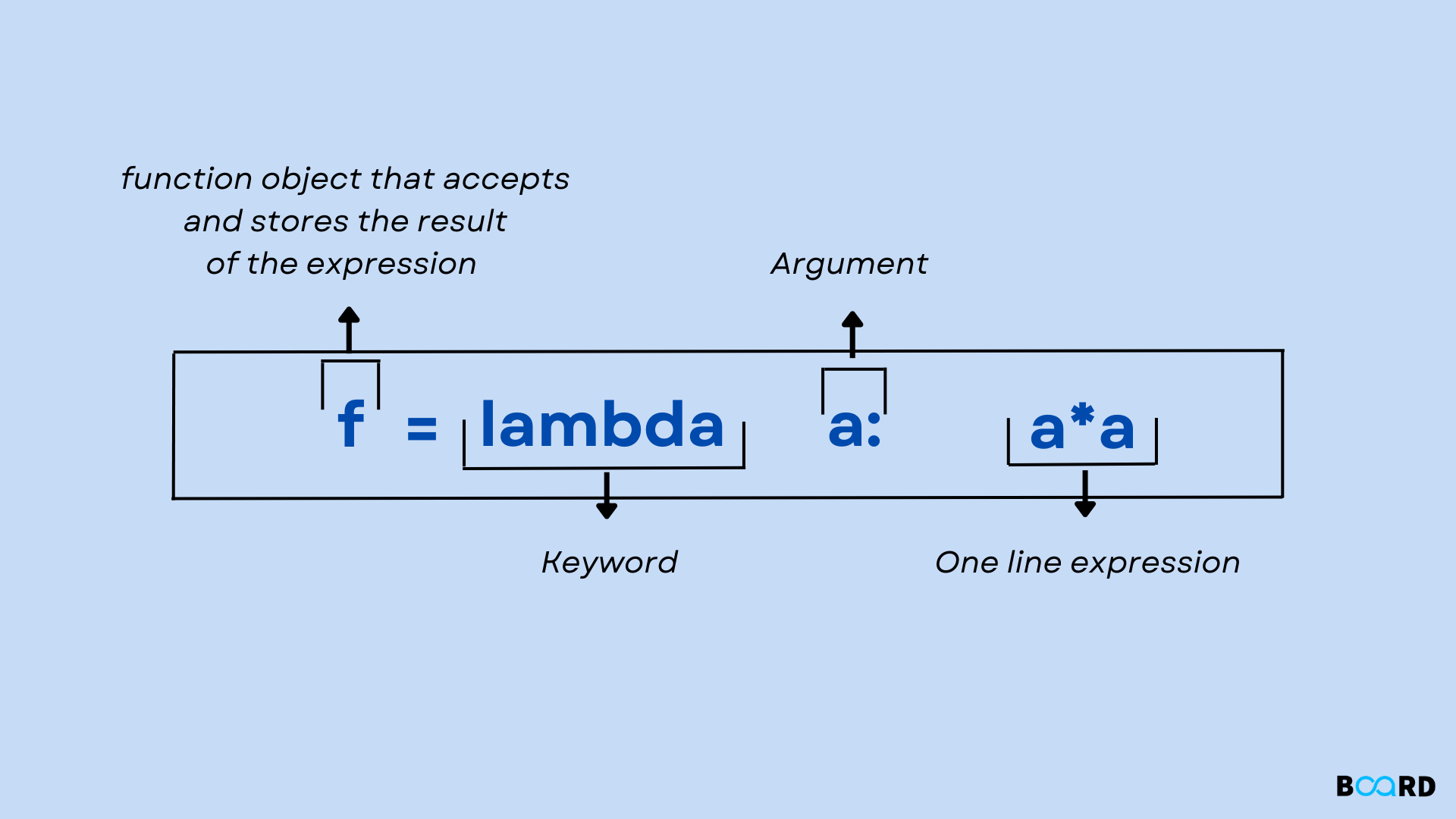
Introduction
Lambda function in Python are the anonymous function that means that the function has the anonymous name. As we already know, a standard Python function is defined using the def keyword. Similar to this, Python uses the lambda keyword to define an anonymous function.
Syntax
Below is the syntax used for defining the lambda function:
lambda arguments: expression
- There is only one expression that is evaluated and returned in this function, regardless of the number of parameters.
- Lambda functions can be used anywhere that function objects are required.
- The fact that lambda functions are syntactically limited to a single expression must always be kept in mind.
- In addition to several kinds of expressions in functions, it has a variety of purposes in specific programming disciplines.
Example
Now, we know some basics about the lambda function in python, lets see a basic example of how it works. In this example, we are just reversing the string.
Output:
Difference between lambda function and def keyword:
Some examples of Python Lambda Function
Example 1: Python lambda function with list comprehension.
In this example, we will be using lambda function for making lists.
Output:
Example 2: Python lambda function with if-else.
In this example, we will be using lambda function with if-else to find the minimum of two numbers.
Output:
Example 3: Using lambda() function with filter().
In this example, we will be filter out all the even numbers from the list.
Output:
Example 4: Using lambda() function with map().
In this example, we will be using map to update all the aray elements.
Output: