Mastering Advanced Python Concepts
Try-Except Block in Python (Using with Examples)
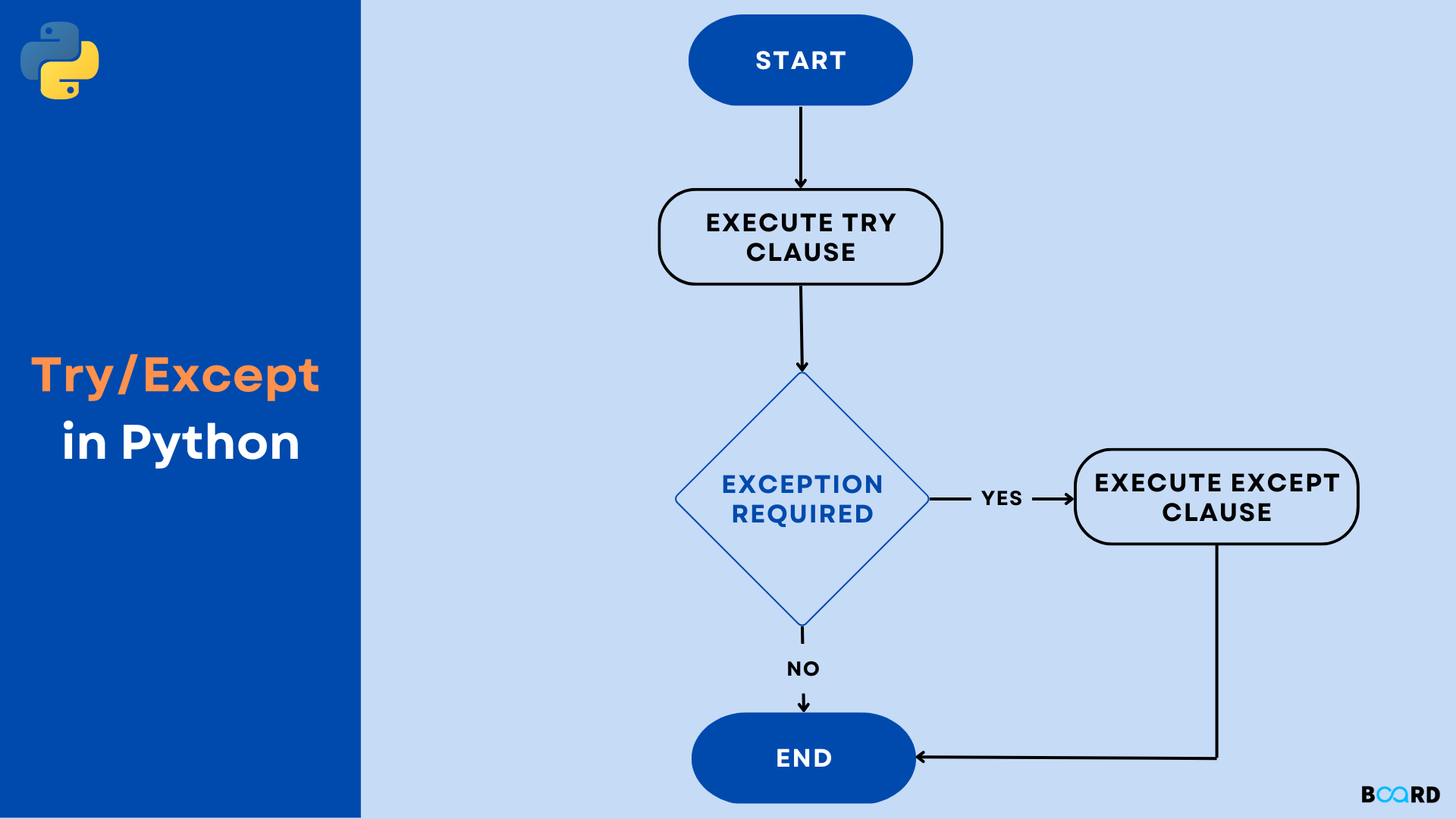
Introduction
With the aid of examples, you will learn how to use the try, except, and finally statements to manage errors in your Python script in this article.
Many built-in exceptions in Python are thrown when a problem is encountered by your application (something in the programme goes wrong). The Python interpreter pauses the running process and gives control to the caller process until these exceptions are addressed. The software will crash if it is not addressed. Consider a software where function A calls function B, and function B calls function C. An exception that happens in function C but isn't handled there is sent to B before being handled by A. If the mistake is never addressed, a notification is shown and our software abruptly and unexpectedly stops.
Catching Exceptions in Python
A try statement in Python can be used to manage exceptions. The try clause contains the crucial operation that can cause an exception. The except clause contains the code that manages exceptions. Thus, after we have identified the exception, we may decide what actions to take. Here is an easy illustration.
Output
We cycle over the values in the randomList list in this application. The try block contains the code that, as was discussed earlier, might lead to an exception. The unless block is bypassed and regular flow resumes if there is no exception (for last value). But the unless block handles any exceptions that do arise (1st and 2nd values). Here, we use the exc info() method in the sys module to output the name of the exception. As we can see, a results in ValueError whereas 0 results in ZeroDivisionError. We may alternatively complete the aforementioned work in the following manner because every exception in
Python derives from the basic Exception class:
The output from this script is identical to that of the one above.
Handling Specific Exceptions
The except clause in the aforementioned example lacked any specific exceptions. This is an inappropriate programming style since it will handle every error and circumstance equally. An except clause can specify which exceptions it should cover. A try clause may include as many except clauses as necessary to address the possible exceptions, but only one of them will be implemented in the event of an exception. A tuple of values can be used to specify several exceptions in an except clause. This is an example of pseudocode.
Raising Exceptions in Python
Exceptions are produced in Python programming when runtime issues happen. Using the raise keyword, we can manually raise exceptions as well. If we want to know why an exception was raised, we may optionally give data to the exception.
Try with else clause
If the code block within the attempt executed without any issues, you could occasionally wish to run another section of code. You can pair the try statement with the optional else keyword in certain situations. Note: The preceding except clauses do not apply to exceptions in the else clause.
Let's examine an illustration:
If we pass an even number, the reciprocal is computed and displayed.
However, if we pass 0, we get ZeroDivisionError as the code block inside else is not handled by preceding except.
Try...finally
In Python, a finally clause is an optional addition to the try statement. This phrase, which is typically used to release external resources, is always put into effect. For instance, we may be utilizing a file or a GUI while connecting through the network to a distant data center.
In either of these scenarios, whether the programme ran successfully or not, we must clear away the resource before it terminates. The finally clause carries out these operations (closing a file, shutting a GUI, or disconnecting from the network) to ensure execution. Here is a file operation example to demonstrate this.
Even if an exception arises while the script is running, this kind of technique ensures that the file is closed.