Mastering Advanced Python Concepts
Ternary Operator in Python
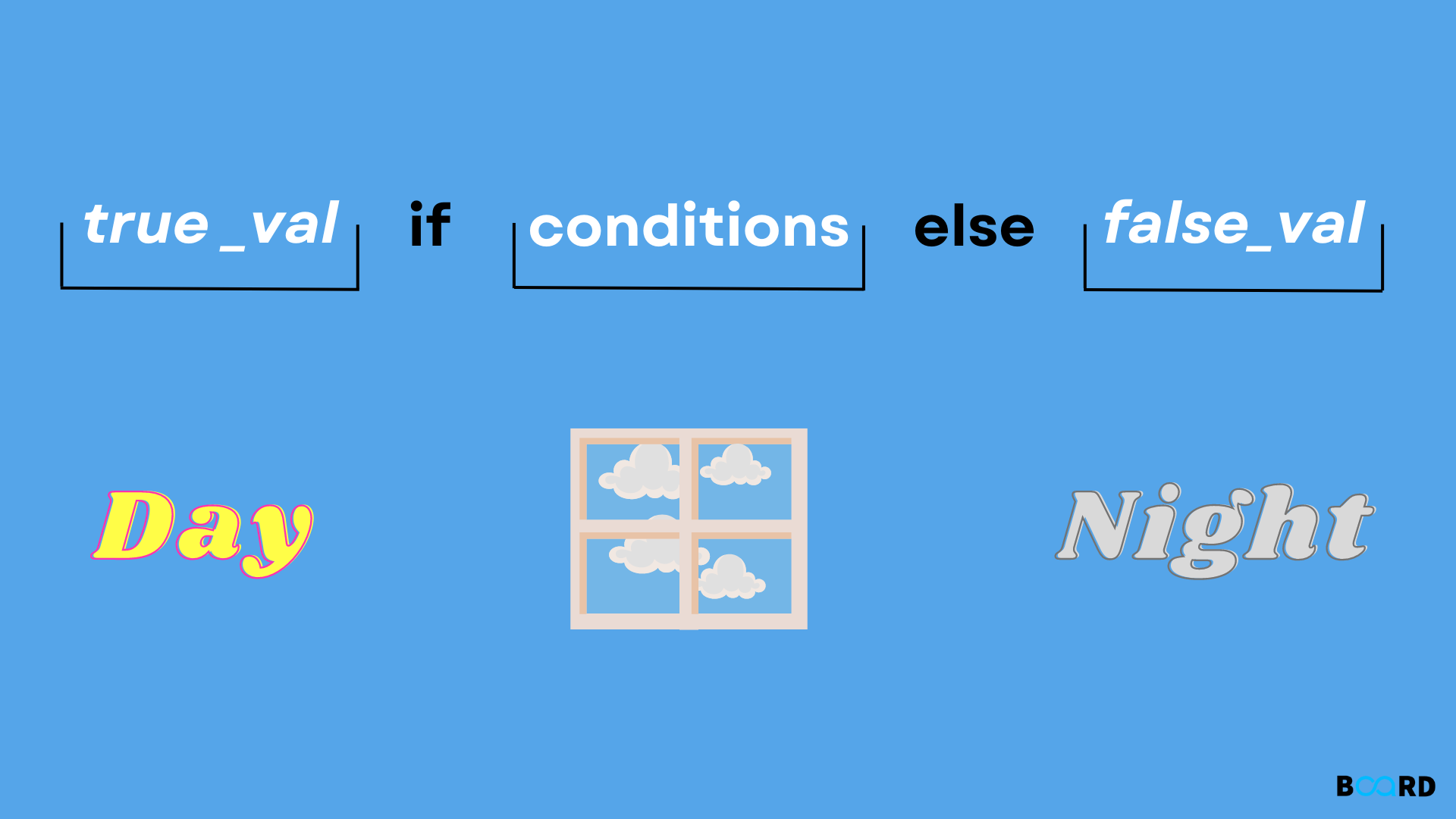
Why we need Ternary Operator in python?
If-else statements in Python are fairly simple to construct and read. They do, however, have a drawback. In other words, four lines are required to print something based on a condition.
There are just too many lines for such a straightforward task. You ask, "What's the solution?" Python's ternary operators
What is Ternary Operator in Python?
The Python ternary operator is nothing more than an if-else statement condensed into a single line. It offers an alternative to the multi-line if-else syntax that allows conditional statements to be written on a single line.
Syntax :
The ternary operator in Python has three operands:
1. Conditional expression: This is a boolean expression that can be either true or false when evaluated.
2. True value: The amount returned by the ternary operator when the conditional expression evaluates to True.
3. False value: The value that the ternary operator returns when the conditional statement evaluates to False.
Example 1: Conditional expression is a boolean value
Output:
Output:
Example 2: Conditional expression is a boolean expression
Output:
Example 3: Variable assignment
Output:
The variable largest gets the value of:
- a, if a is greater than b
- b, if a is not greater than b
Utilising Tuple, Ternary Operator
Using a tuple is an additional method for implementing ternary operations in Python. The if-else ternary operator can be easily replaced with this.
Syntax :
In this instance, the two components of the tuple are false value and true value. And in instead of an index, the conditional expression is enclosed in square bracket notation. Simply because True has a value of 1, and False has a value of 0, this works.
Therefore:
- The value is whether the conditional expression evaluates to True.
- The ternary expression then returns the element at index 1.
- And its value becomes 0 if the conditional expression evaluates to False.
- The element with index zero is then returned.
Ensure that the false_value at index 0 and true_value at index 1 in the tuple.
Output:
Output:
We can extend this syntax and use a list or a dictionary instead of a tuple.
Using a list, Ternary Operation
Example:
Output:
Using a dictionary, Ternary Operations
Here, the true value and false value take the place of the respective values for the keys True and False. The value corresponding to True is returned if the conditional expression evaluates to True. In all other cases, the False value is returned.
Example
Output:
Conclusion
We have now reached the end of the article. We learned about numerous Python ternary operator implementation strategies. We observed how ternary operators improve the readability and compactness of the code.
To make your code more "Pythonic," utilise ternary operators!