Mastering Advanced Python Concepts
Learn about Boolean in Python
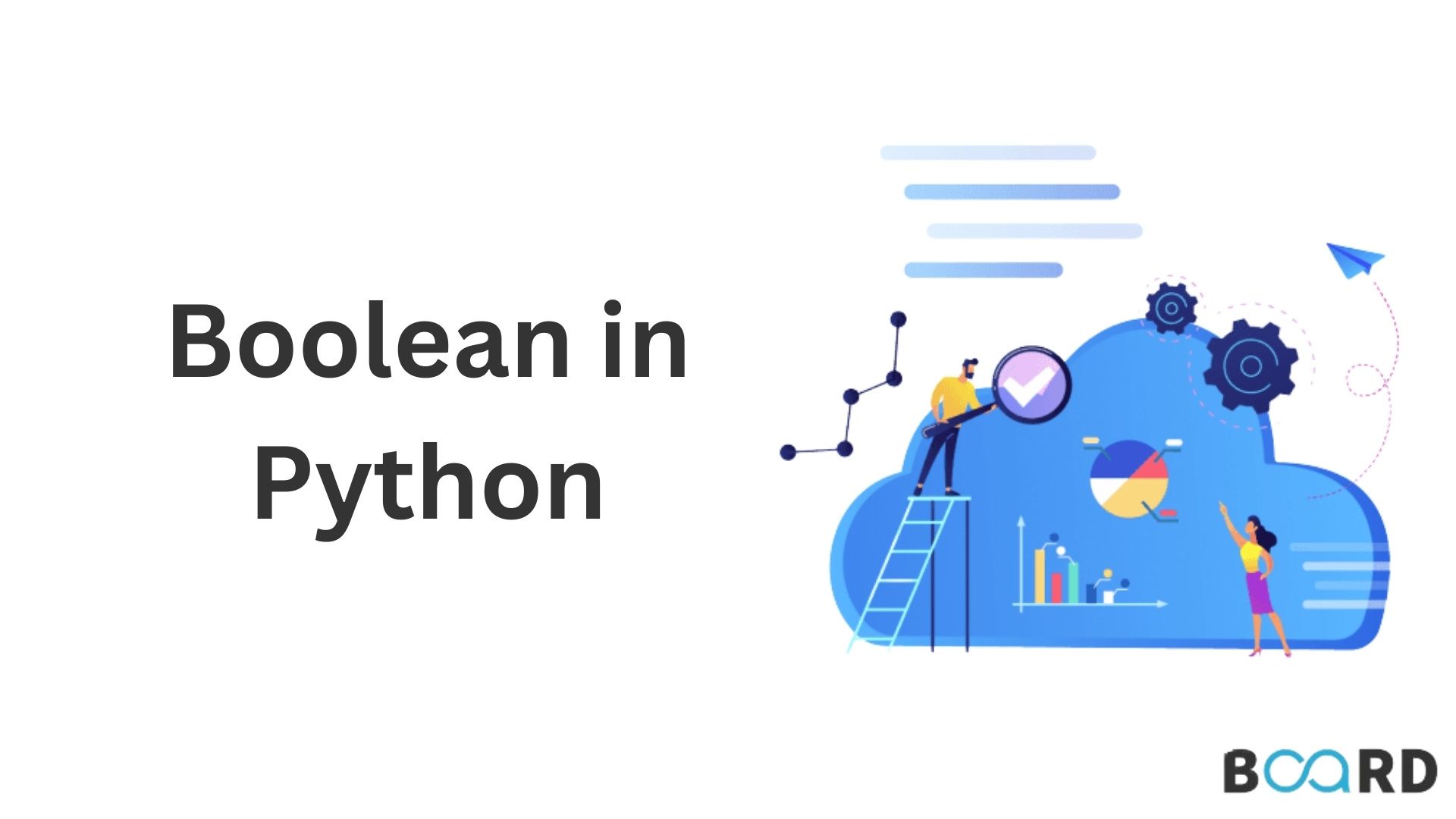
Introduction
A boolean variable is one that has the values True or False. We state that a variable's data type may be boolean. It resembles a number that can either be 0 or 1 in a numerical context. Electronics either have high or low voltage. You can compare it to a light switch because it can only be turned on or off. It is based on a bit, the smallest computing unit. 0 (true) or 1 (false) is the value of a bit. For now, you can think of "bit" as a synonym for "boolean."
Boolean in Python
A boolean value has two possible outcomes: false or true. Boolean built-ins in Python are capitalized, such as True and False. The data type can be set to boolean without having to be defined explicitly. As in C or Java, you don't need to explicitly state that you wish to use a boolean. As a result of the value you enter, Python understands that the variable is a boolean. Compare the boolean definition code below:
The foundation of Python and the majority of computer languages is boolean logic. It enables programmers to apply standard algorithms, run conditional statements, and do comparisons. Python's comparison operators include the "greater than" (>) and "equals to" (==) symbols, while its logical operators include and and or. The usage of Python's Boolean operators is covered in this lesson, along with explanations of Boolean logic and expressions.
Python requires the following syntax to define a boolean:
This generates a boolean with the value False and the variable name (a). You would type: if you wanted to turn it on.
The print function can be used to display a variable's value. You can simply type the variable name in the Python shell:
Even though you might assume that's a boolean, if you ask for the type, you'll get int (number, integer):
To turn a number into a boolean in Python, you must call the bool() method.
Things to remember
- A genuine number of any size, whether positive or negative, can never be false. False are the numbers 0 and 0.0.
- When performing mathematical operations on real numbers, rounding mistakes might produce results that are unclear or deceptive.
- Even though a variable "should" be zero, rounding processes may result in a very tiny non-zero value.
- This would be a True evaluation. A text, list, set, or dictionary that is empty evaluates to False.
- Data structures or strings that are not empty are True. None is a unique Python value that is False.
- The special values NaN (for non-representable or undefinable values), inf, and -inf are all True. A function is True at all times.
Cast boolean
Using the function bool()., you may convert a value to a boolean. The data type of a variable is altered through casting. The data type in the example below changes from int to boolean.
Using the type() method call, which returns the data type, you can confirm this.
Boolean strings
Functions occasionally return Boolean values. If you define a string, you can call multiple methods, each of which returns a boolean value.