Mastering Advanced Python Concepts
Try, Except, Else and Finally in Python
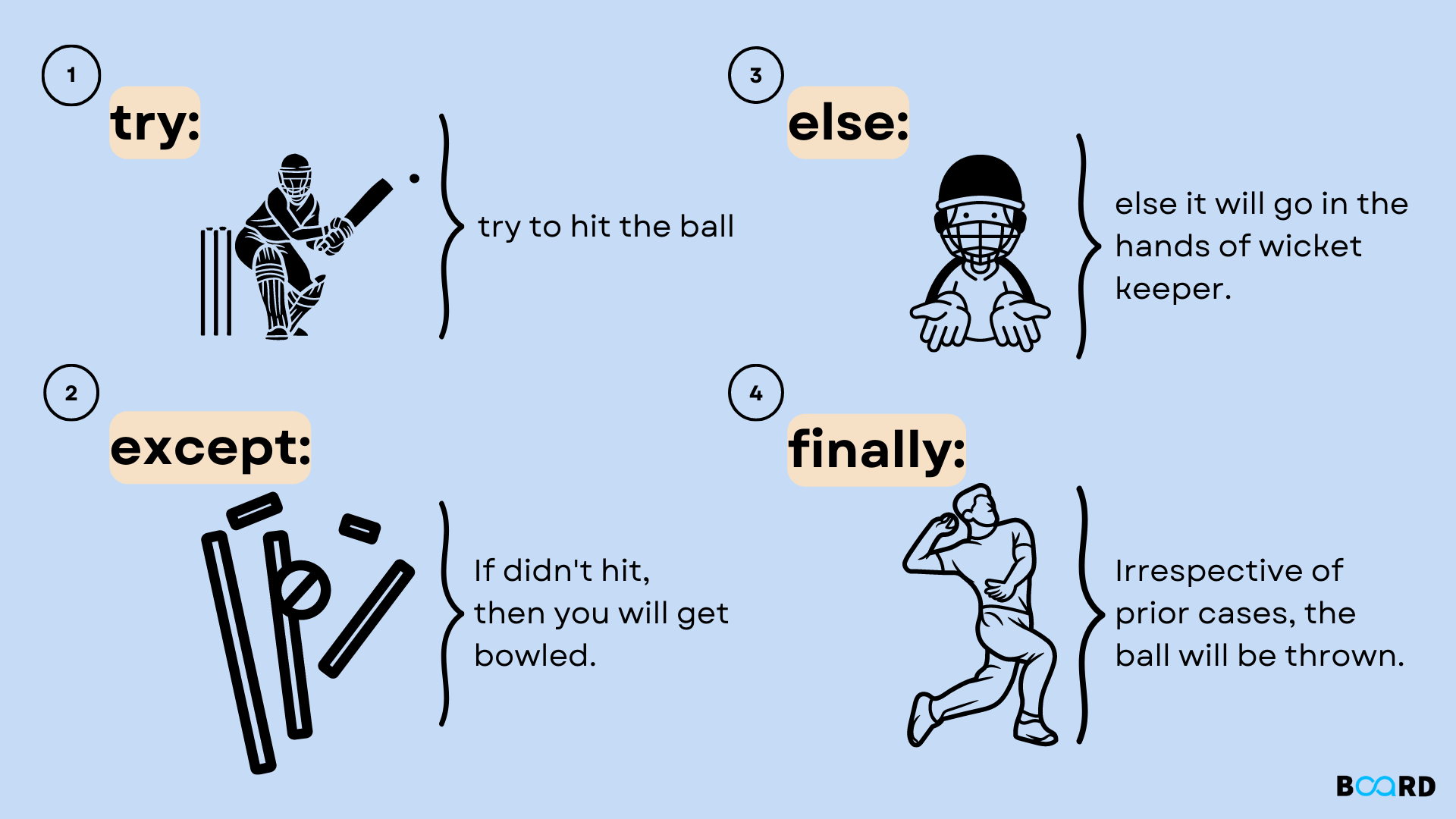
Introduction
In python, an error can be of two types:
- Syntax errors
- Exceptions
Syntax errors: Errors are defined as the problems that cause the program to stop its execution.
Exceptions: Exceptions cause a change in the normal flow of the program due to some internal events that occurred in the program.
Some common exceptions are:
IOError: When a file can not be opened this exception is raised.
KeyboardInterrupt: This exception is raised when an unwanted key is pressed.
ValueError: When a wrong argument is passed by the user this exception is raised.
EOFError: When reading a file, if the pointer hit End-Of-File is hit and no data is read from the file.
ImportError: When importing if a module is not found.
Try-Except in Python
In Python, to handle errors in a program, we use a try-except statement.
try block: The try block is used when the code is needed to check for some errors. If there are no errors in the code then the code under the try block will get executed.
except block: Whenever there is some error in the try block preceding the except block, the code under except block executes.
Syntax
How does the try-except work
- First, the try block is executed.
- If any exception is not there in the code, then the try block will execute, except the block is finished.
- If an exception occurs then the except block will be executed and the try block is skipped.
- If an exception is still raised and the except block is left unhandled then it is passed to the outer try blocks. If still it is left unhandled then eventually the execution stops.
- A try statement can have more than one except block.
Some examples
Code 1
There is no exception, so try block will execute.
Output
Code 2
An exception is present, so the except block will run.
Output
Else Block
An else block can also be added on the try-except block and it should be present after all the except blocks. If the try block successfully does not raise an exception then the code enters into the else block.
Syntax
Code
Output
Finally Keyword
In Python, we use the final keyword, which is executed always even if the exception is not handled and the try-except block is terminated. It is executed after the try-except block.
Syntax
Code
Output