Mastering Advanced Python Concepts
Local vs Global Variable in Python
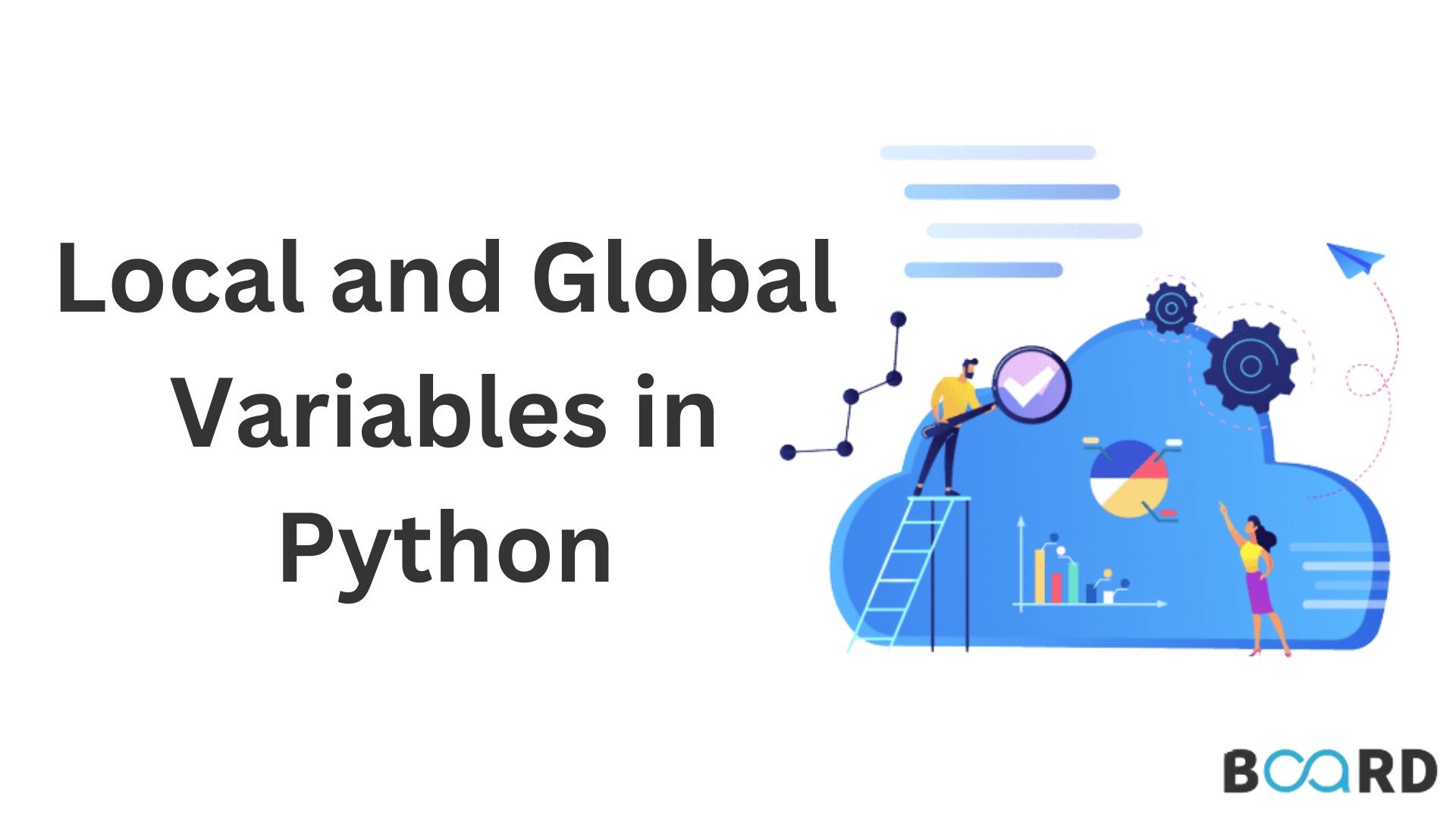
Introduction
All variables are not accessible at all locations. Certain variables accessible somewhere may not be reachable elsewhere. The scope of a variable determines where a given variable may be accessible. There are two types of scopes in Python namely local and global. Local as the term suggests is available only in the given block whereas global, on the other hand, is available everywhere and in every part of the program. The following sections will further elucidate these two types of variables
Global Variables
Global variables are those variables that are accessible at any part of the program and inside every function as well. These include variables declared at the top of the interpreter or outside any function or using the global keyword which will be discussed later
Example
Output
But a question may arise: What if there’s a variable having the same name inside the function? Let us understand this case through an example
Example
Output
In this case, Python creates a local variable name string inside the function, and the print statement prints the local value of the variable.
The next question arises, What if we try to modify the value inside the function? The answer is that it would result in an error, as Python would try to modify the value of “string” which would be treated as a local variable. However, this local variable has not been initialized and thus would cause an error
Example
Output
So how do we modify the value of the variable? This can be done with the help of the “global” keyword which informs that the variable inside this function is global and can be modified. As a result, the value of the variable is modified
Example
Output
Local Variables
Local variables are those which are created inside a function and can be accessed only within the function it has been created. For example,
Example
Output
Another question may arise. What happens if we try to access the value of a local variable outside its defined function
Example
Output
It results in an error since the scope of the variable is only limited to within the function and trying to access it from outside will cause an error
Importance of Local and Global Variables
Local and global variables play an important role when working on projects, especially while collaborating with different people who might have different approaches while working towards a solution. As a good programming practice, it is vital to ensure the minimum usage of global variables as it is not advisable as these variables are generally exposed and this is quite unsafe when dealing with sensitive information such as bank details, and personal details. Passing variables through functions and making them local only to the required function allows better readability and understandability of the code.
Conclusion
By the end of this section, you would have a clear idea of local and global variables as well as understand when and how to use them for appropriate applications. A global variable can be accessed from anywhere whereas a local variable can be accessed only within the function.